Improving Test Coverage Analysis in SonarQube.
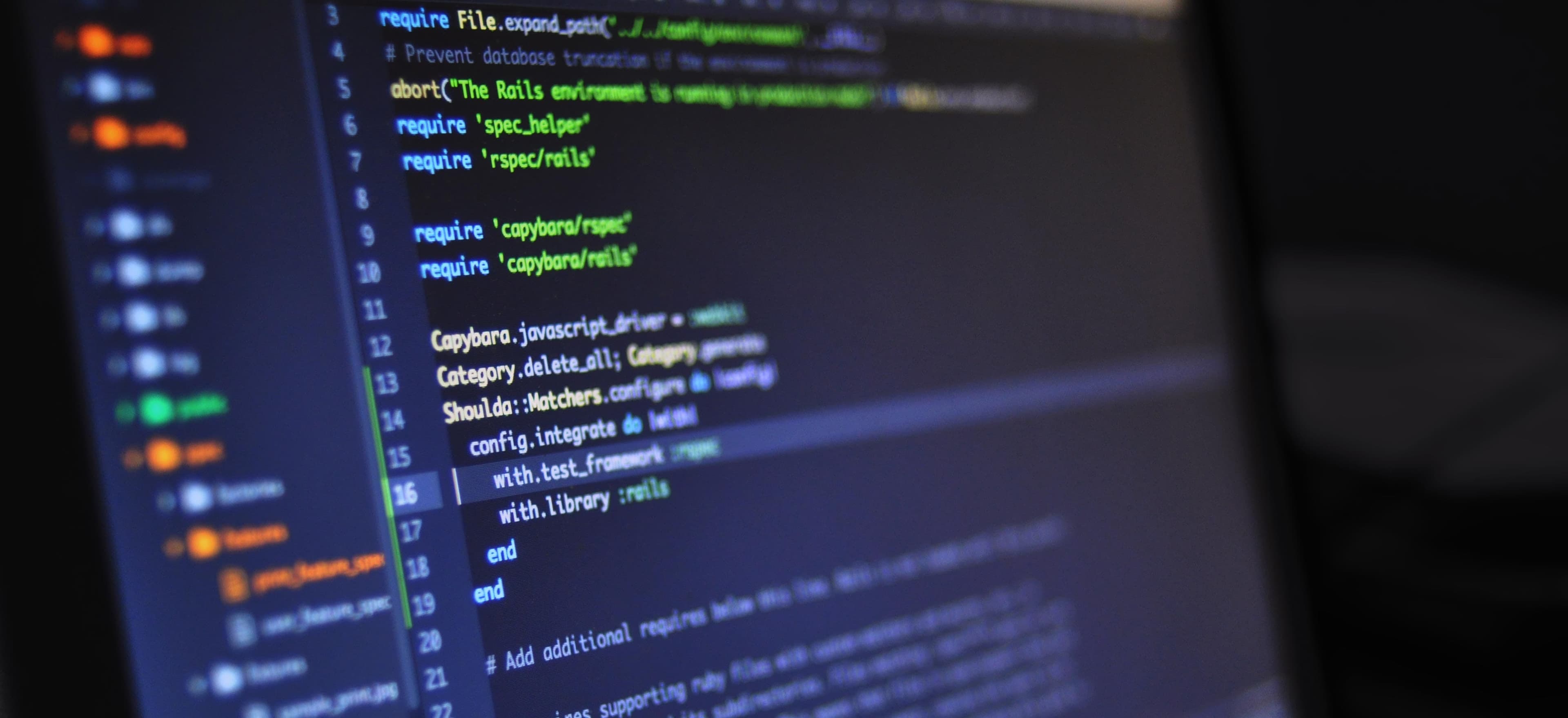
- Published on
Improving Test Coverage Analysis in SonarQube
A Brief Overview
Test coverage analysis is a critical aspect of ensuring code quality and identifying areas that lack sufficient testing. SonarQube is a popular tool that provides static code analysis to detect bugs, vulnerabilities, and code smells. In addition to these features, SonarQube also offers test coverage analysis to evaluate the effectiveness of the test suite. In this article, we will explore some strategies to enhance test coverage analysis in SonarQube, utilizing Java as the primary programming language.
Understanding Test Coverage Analysis
Before delving into improving test coverage analysis, it's crucial to grasp the concept of test coverage. Test coverage is a measure used to describe the degree to which the source code of a program is executed when a particular test suite runs. It helps in identifying areas of the code that are not adequately exercised by the existing tests.
Why is Test Coverage Analysis Important?
- Enhances code quality.
- Identifies untested areas in the codebase.
- Aids in creating more effective test cases.
Configuring SonarQube for Test Coverage Analysis
Integration with Build Tools
SonarQube integrates seamlessly with popular build tools such as Maven and Gradle. By setting up the necessary configurations in the project's build file, test coverage reports can be generated and fed into SonarQube for analysis. Let's take a look at how this can be accomplished using Maven.
<build>
<plugins>
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.7</version>
<executions>
<execution>
<id>prepare-agent</id>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
<execution>
<id>report</id>
<goals>
<goal>report</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
In the above snippet, we are configuring the jacoco-maven-plugin
to generate test coverage reports during the build process.
SonarQube Configuration
After setting up the build tool integration, the next step is to configure the SonarQube server to analyze the generated test coverage reports. This can be done by defining properties in the sonar-project.properties
file or through the SonarQube UI.
Example configuration in sonar-project.properties
:
sonar.projectKey=my-project
sonar.projectName=My Project
sonar.projectVersion=1.0
sonar.sources=src
sonar.tests=src/test
sonar.language=java
sonar.java.coveragePlugin=jacoco
sonar.jacoco.reportPaths=target/jacoco.exec
In this configuration, we specify the project key, name, version, source directories, test directories, and the coverage plugin to be used (in this case, JaCoCo). Additionally, we provide the path to the Jacoco coverage report generated during the build.
Strategies for Enhancing Test Coverage Analysis
Identify Untested Code Paths
SonarQube provides insights into untested code paths, allowing developers to focus their testing efforts on areas that lack adequate coverage. By regularly monitoring these uncovered paths, developers can ensure that a broader range of scenarios in the codebase are being tested, leading to more resilient and reliable software.
Writing Effective Unit Tests
Improving test coverage analysis ultimately comes down to writing effective unit tests that exercise various code paths. Unit tests should be designed to cover different branches, conditions, and edge cases within the code. Utilizing mocking frameworks such as Mockito can aid in simulating complex scenarios and dependencies, thereby increasing the comprehensiveness of the tests.
Example of Writing a Unit Test using JUnit and Mockito:
@Test
public void testCalculateOrderTotal() {
// Mocking dependencies
Product product1 = mock(Product.class);
Product product2 = mock(Product.class);
when(product1.getPrice()).thenReturn(10.0);
when(product2.getPrice()).thenReturn(20.0);
ShoppingCart cart = new ShoppingCart();
cart.addProduct(product1);
cart.addProduct(product2);
// Executing the method to be tested
double total = cart.calculateOrderTotal();
// Assertions
assertEquals(30.0, total, 0.001);
}
In this example, we are testing the calculateOrderTotal
method of a ShoppingCart
class by mocking Product
instances using Mockito to simulate the scenario.
Continuous Integration and Test Coverage
Integrating test coverage analysis into the continuous integration/continuous delivery (CI/CD) pipeline is vital for ensuring that test coverage metrics are consistently monitored. Incorporating SonarQube analysis into the CI/CD workflow allows teams to detect any significant drops in test coverage early on and take necessary corrective measures.
Closing the Chapter
Improving test coverage analysis in SonarQube involves a multi-faceted approach including effective test writing, consistent monitoring of code coverage metrics, and seamless integration with the build and CI/CD processes. By leveraging the capabilities of SonarQube and adopting best practices in test-driven development, teams can enhance the quality and reliability of their software products.
Incorporating these strategies will not only lead to better test coverage analysis in SonarQube but also contribute to overall improvements in code quality and maintainability. By monitoring test coverage and ensuring the effectiveness of the test suite, development teams can proactively identify and address potential issues, ultimately delivering higher quality software to end users.
To further explore the capabilities of SonarQube for test coverage analysis, refer to the official SonarQube documentation.
Remember, successful test coverage analysis is not just about meeting a numerical threshold, but about instilling confidence in the codebase and the resilience of the software it builds.