Optimistic Locking: Handling Data Concurrency Efficiently
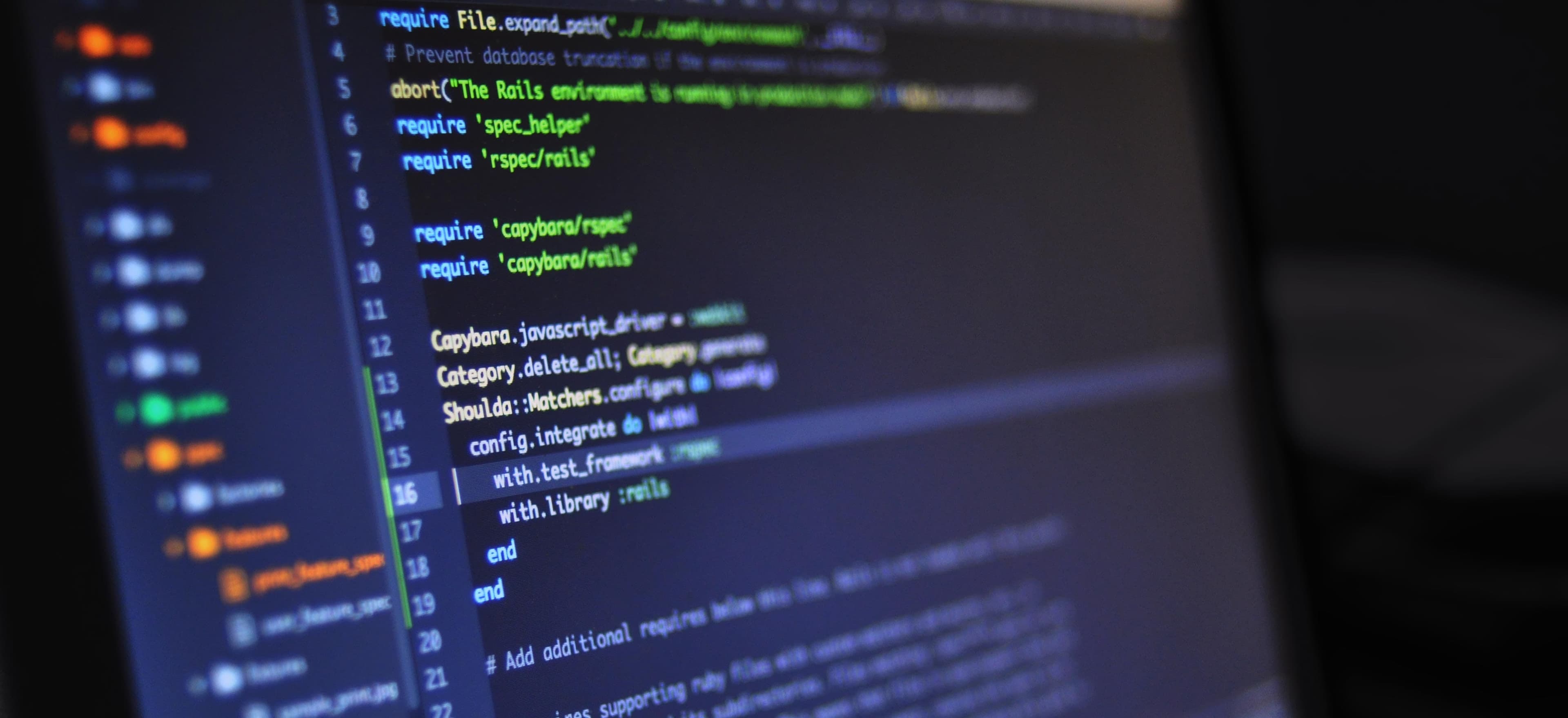
- Published on
Optimistic Locking: Handling Data Concurrency Efficiently
In a multi-user environment, data concurrency is a critical challenge for any application dealing with a database. When multiple users or processes attempt to modify the same piece of data simultaneously, conflicts and data inconsistencies can arise.
Optimistic locking is a technique used to manage data concurrency by assuming that conflicts are unlikely to occur. It is based on the premise that concurrent transactions are rare, and therefore, the system should proceed with the operations without acquiring locks on the data. However, it employs a strategy to detect and resolve conflicts if they occur. This approach is in contrast to pessimistic locking, where locks are acquired on data during read and write operations to prevent conflicts, incurring the risk of potential performance bottlenecks due to contention.
How Does Optimistic Locking Work?
The concept of optimistic locking revolves around the versioning of data. Each record in the database is associated with a version number or a timestamp. When a user retrieves a record, the version or timestamp is also fetched. Upon updating the record, the system checks if the version in the database matches the version that was initially retrieved. If the versions match, the update proceeds as expected, and the version number is incremented. However, if there is a mismatch, it indicates that another process has modified the record in the interim, leading to a conflict resolution mechanism such as rejecting the update or prompting the user to reconcile the differences.
Implementing Optimistic Locking in Java
Let's delve into a Java application to understand how optimistic locking can be implemented using JPA (Java Persistence API) for managing database concurrency.
Entity Class with Versioning
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.Version;
@Entity
public class Product {
@Id
private Long id;
private String name;
private double price;
@Version
private long version;
// Getters and setters
}
In the above example, the @Version
annotation is used to indicate the version attribute for the Product
entity. This attribute will be automatically managed by the JPA provider, and its value will be incremented whenever an update operation is performed on the entity.
Updating Entity with Optimistic Locking
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.EntityTransaction;
import javax.persistence.Persistence;
public class ProductDAO {
private EntityManagerFactory entityManagerFactory = Persistence.createEntityManagerFactory("persistenceUnit");
public void updateProductPrice(Long productId, double newPrice) {
EntityManager entityManager = entityManagerFactory.createEntityManager();
EntityTransaction transaction = entityManager.getTransaction();
try {
transaction.begin();
Product product = entityManager.find(Product.class, productId);
product.setPrice(newPrice);
entityManager.merge(product);
transaction.commit();
} catch (Exception e) {
if (transaction.isActive()) {
transaction.rollback();
}
// Handle the exception
} finally {
entityManager.close();
}
}
}
In the updateProductPrice
method, we retrieve the Product
entity using entityManager.find
, and then update its price before merging the changes back into the database with entityManager.merge
. If another transaction has modified the same Product
instance concurrently, an OptimisticLockException
will be thrown. Proper exception handling and conflict resolution strategies should be implemented based on the specific application requirements.
Benefits of Optimistic Locking
-
Reduced contention: Optimistic locking minimizes the need for acquiring locks on database records, leading to reduced contention and improved performance in high-concurrency scenarios.
-
Improved scalability: By allowing concurrent transactions to read and potentially update the same data without blocking each other, optimistic locking supports better scalability for applications.
-
Conflict resolution: Since conflicts are detected at the time of update, applications can implement custom conflict resolution strategies such as prompting users to merge changes or automatically resolving conflicts based on predefined rules.
Best Practices for Optimistic Locking
-
Use versioning: Always leverage versioning for entities managed through optimistic locking. Most JPA providers offer seamless support for versioning through annotations like
@Version
. -
Handle optimistic lock exceptions: Incorporate robust error handling and conflict resolution logic to deal with
OptimisticLockException
and ensure data consistency. -
Isolate update operations: When performing updates within a transaction, encapsulate the read-modify-write operations to limit the exposure to concurrent modifications.
Bringing It All Together
Optimistic locking offers an efficient approach to handle data concurrency in applications. By assuming that conflicts are infrequent and employing versioning to detect and resolve concurrent modifications, it strikes a balance between ensuring data consistency and minimizing performance impact. When implemented thoughtfully, optimistic locking can contribute to the overall robustness and scalability of database-driven applications.
Understanding how optimistic locking works and its practical implementation in Java empowers developers to design data access layers that are resilient to concurrency challenges, ultimately delivering a more reliable and responsive user experience.
To delve further into the world of Java and database concurrency, consider exploring Java Concurrency in Practice, a comprehensive guide to writing concurrent and multicore applications in Java.