Troubleshooting Capitalization Errors in Java Quarkus with GraalVM
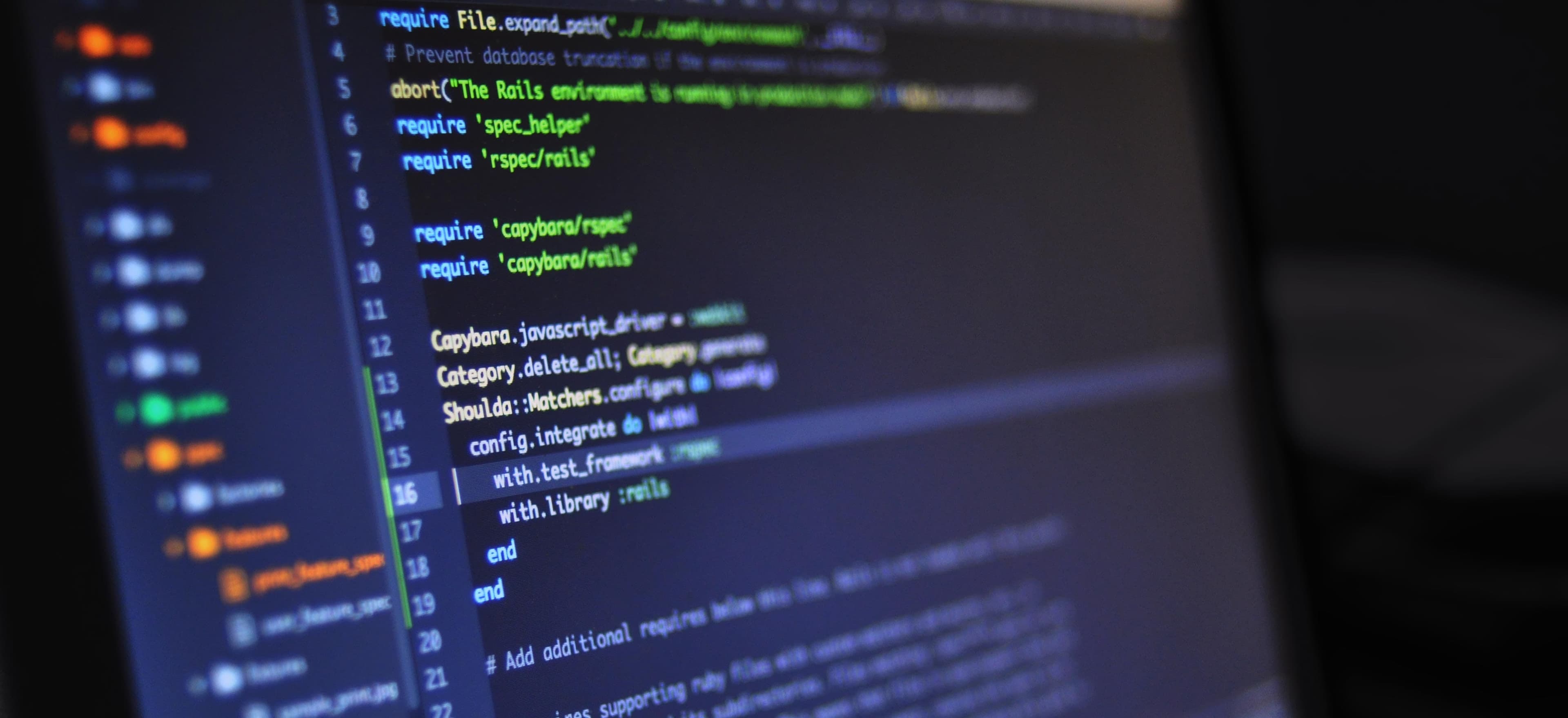
- Published on
Troubleshooting Capitalization Errors in Java Quarkus with GraalVM
When developing applications in Java, especially when using modern frameworks like Quarkus and GraalVM, it's common to encounter capitalization errors that can be challenging to debug. In this blog post, we'll explore common scenarios where capitalization errors occur in Java applications built with Quarkus and GraalVM, and we'll provide practical solutions to troubleshoot and fix these issues.
Understanding Capitalization Errors
Capitalization errors in Java can manifest in various ways, such as incorrect package names, class names, or method names. These errors can occur due to differences in how different operating systems handle file and directory names, as well as discrepancies in case sensitivity.
When developing applications with Quarkus and GraalVM, capitalization errors can become more pronounced due to the ahead-of-time (AOT) compilation performed by GraalVM, which enforces strict adherence to case sensitivity rules.
Common Scenarios
Package Name Mismatch
One common scenario is a package name mismatch between the import statements in your Java code and the actual package structure on the file system. For example, you may have an import statement like this:
import com.example.myapp.MyClass;
However, the actual package structure on the file system is:
com/example/myapp/MyClass.java
This discrepancy in capitalization can lead to compilation errors when building the application with GraalVM.
Class Name Mismatch
Another scenario is a mismatch between the class name used in your Java code and the actual class file name on the file system. For example, you may have a class declaration like this:
public class MyService {
// ...
}
However, the actual file name on the file system is:
MyService.java
If the capitalization doesn't match exactly, GraalVM may fail to locate the correct class during the AOT compilation process.
Method Name Mismatch
Similarly, a mismatch in method names due to capitalization errors can lead to runtime errors when running the application compiled with GraalVM. For instance, if you have a method declaration like this:
public void processData() {
// ...
}
But the method invocation in your code is:
processData();
With the incorrect capitalization, GraalVM may not be able to resolve the method invocation correctly.
Troubleshooting and Solutions
Use Consistent Capitalization
The most effective way to avoid capitalization errors is to ensure consistent capitalization across all package names, class names, and method names in your Java code and file system. This involves meticulously reviewing and standardizing the capitalization of all identifiers to match the file system structure.
Leverage IDE Refactoring Tools
Modern integrated development environments (IDEs) such as IntelliJ IDEA and Eclipse offer powerful refactoring tools that can assist in standardizing capitalization. Utilize IDE features like "Rename" to refactor package, class, and method names seamlessly across your codebase. This can help ensure consistency and reduce the likelihood of capitalization errors.
Perform Case-Sensitive File System Checks
Before building your Quarkus application with GraalVM, perform a case-sensitive check of the file and directory names in your project. This ensures that the package names, class names, and method names in your code exactly match the file system structure, thereby preventing capitalization errors during the AOT compilation process.
Verify JAR Packaging
When packaging your Quarkus application into a JAR file, ensure that the file and directory names within the JAR maintain consistent capitalization. This can be achieved by validating the JAR contents using tools like jar tf
and correcting any discrepancies in capitalization.
Use GraalVM Configuration
GraalVM provides configuration options to customize the AOT compilation process. By configuring the GraalVM build to be case-insensitive, you can mitigate capitalization errors during compilation. However, this approach should be used with caution, as it may compromise the intended case sensitivity of your application.
Closing the Chapter
Capitalization errors can be a source of frustration when developing Java applications with Quarkus and GraalVM. By understanding common scenarios and employing the troubleshooting solutions outlined in this blog post, you can effectively mitigate capitalization errors and ensure smooth AOT compilation and runtime execution of your applications.
To further delve into the nuances of configuring GraalVM, check out the official GraalVM documentation. Additionally, for insights into best practices for Java application development with Quarkus, refer to the Quarkus project website.
By incorporating these strategies into your development workflow, you'll be better equipped to tackle capitalization errors and optimize the performance of your Quarkus applications with GraalVM.