Leveraging Repeatable Annotations in Java 8
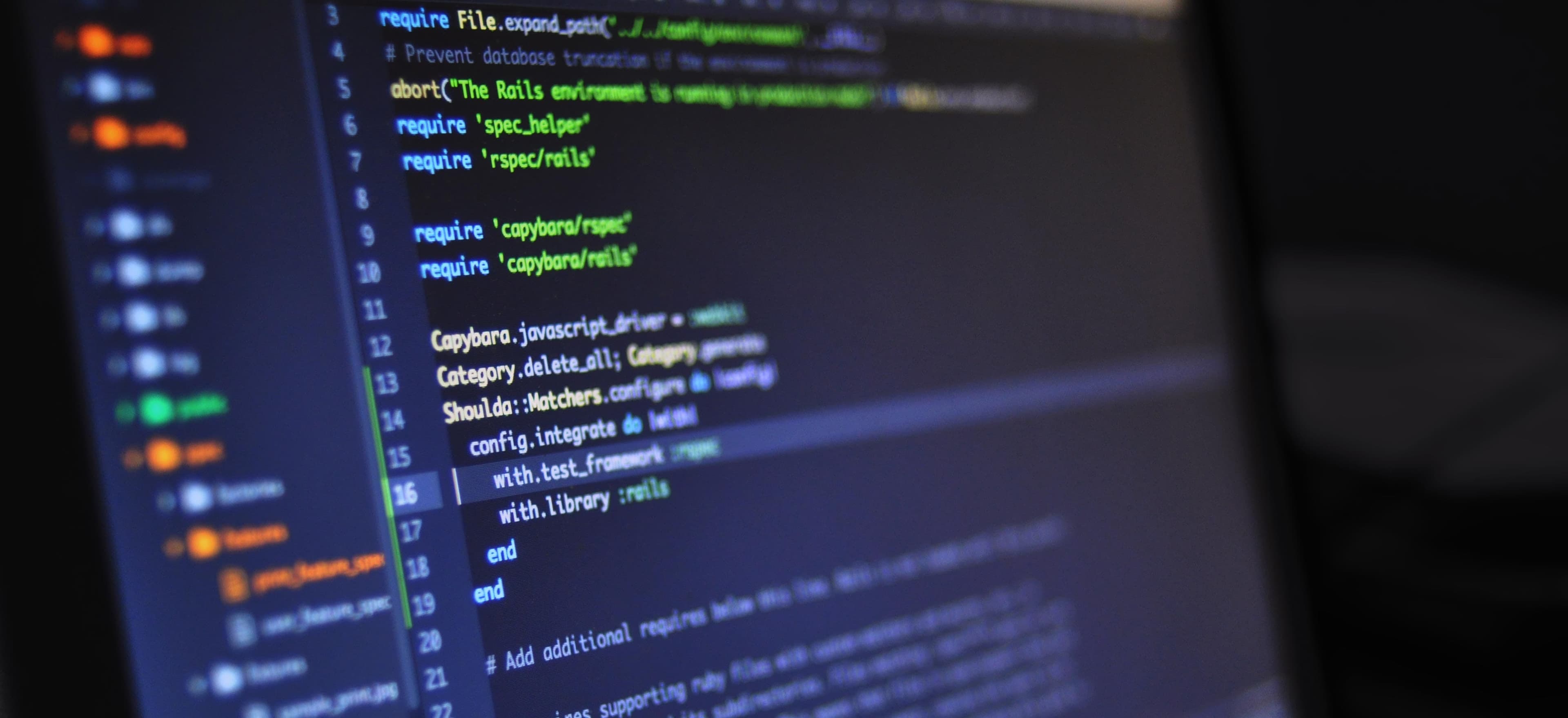
- Published on
In the world of programming, Java has been a predominant force for decades. With each new iteration, the language introduces powerful features that enhance the developer's capabilities. Java 8, in particular, brought about a significant evolution with the introduction of repeatable annotations. This feature allows developers to apply the same annotation multiple times to the same declaration. In this blog post, we will delve into the world of repeatable annotations in Java 8, understand their significance, and explore how to leverage them to write cleaner and more maintainable code.
Understanding Annotations in Java
Before we dive into the concept of repeatable annotations, it's essential to have a solid grasp of annotations in Java. Annotations provide a way to add metadata to your code. They serve as a form of syntactic metadata that can be added to Java source code. Annotations can be applied to packages, classes, methods, fields, parameters, and local variables. They enable developers to convey information about the code to the compiler or runtime environment, or simply to enhance readability and maintainability.
The Limitation of Single Annotations
Prior to Java 8, the usage of annotations had a limitation - you could only use an annotation once at a specific code location. This restriction posed a challenge in scenarios where multiple instances of the same annotation were required. For instance, consider a situation where a class needs to have multiple authors specified using annotations, or when a method requires several types of constraints to be imposed. In such cases, the inability to apply the same annotation multiple times led to code clutter and decreased readability.
Enter Repeatable Annotations in Java 8
Java 8 addressed this limitation by introducing repeatable annotations. With repeatable annotations, an annotation type can be marked as @Repeatable
, allowing it to be applied more than once to the same declaration or type. This not only makes the code cleaner but also improves its readability and maintainability significantly. By using repeatable annotations, developers can convey the intended meaning more effectively, without resorting to convoluted workarounds.
Creating Repeatable Annotations
To define a repeatable annotation type, the @Repeatable
meta-annotation is used. Let's consider a practical example to demonstrate this concept.
Suppose we are building an application where we need to annotate a class with multiple tags. In pre-Java 8, achieving this would have been cumbersome. However, with repeatable annotations, we can create a @Tag
annotation and mark it as @Repeatable
.
import java.lang.annotation.*;
@Repeatable(Tags.class)
@Retention(RetentionPolicy.RUNTIME)
public @interface Tag {
String value();
}
@Retention(RetentionPolicy.RUNTIME)
public @interface Tags {
Tag[] value();
}
In this example, we have created a repeatable annotation @Tag
to annotate the class with a tag and a container annotation @Tags
to hold multiple @Tag
annotations. The use of @Repeatable
meta-annotation facilitates applying multiple tags to the same class without any additional complexity, promoting clean and concise code.
Leveraging Repeatable Annotations
Now that we have a clear understanding of repeatable annotations, let's explore how to leverage them in practice. Consider a scenario where we have a Document
class, and we want to apply multiple tags to it.
@Tag("java")
@Tag("programming")
public class Document {
// Class implementation
}
With repeatable annotations, we can apply multiple tags directly to the Document
class without the need for a container annotation. This not only simplifies the code but also makes it more intuitive and descriptive.
Processing Repeatable Annotations
When it comes to processing repeatable annotations, it's crucial to understand how to retrieve and process the multiple occurrences of a repeatable annotation. In Java, the getAnnotationsByType
method is used to obtain all annotations of a specified type, including repeatable annotations.
Tag[] tags = Document.class.getAnnotationsByType(Tag.class);
By using the getAnnotationsByType
method, we can retrieve all the @Tag
annotations applied to the Document
class, providing a straightforward way to process repeatable annotations without the complexities that existed in earlier versions of Java.
Benefits of Repeatable Annotations
The introduction of repeatable annotations in Java 8 has ushered in a more elegant and expressive way of annotating code. The benefits of using repeatable annotations include:
-
Improved Readability: By allowing the same annotation to be applied multiple times, the code becomes more readable as the intended metadata is clearly expressed without clutter.
-
Simplified Usage: Applying repeatable annotations is straightforward and intuitive, eliminating the need for container annotations or complex workarounds.
-
Enhanced Maintainability: Code maintainability is improved as annotations are better organized, making it easier to comprehend and modify the metadata associated with the codebase.
In Conclusion, Here is What Matters
Repeatable annotations in Java 8 have revolutionized the way annotations are applied, offering a cleaner and more intuitive approach to annotating code with multiple occurrences of the same annotation. By enabling developers to convey their intentions more clearly and concisely, repeatable annotations contribute to the overall readability and maintainability of Java codebases. As you continue to explore the depths of Java programming, consider implementing repeatable annotations to enhance the expressiveness and elegance of your code.
Incorporating new features into your Java applications can greatly affect their performance. If you're interested in learning more about improving the performance of your Java applications, check out this guide on Java Performance Tuning.
Hopefully, this article has shed light on the significance and practical application of repeatable annotations in Java 8. Embrace this powerful feature and leverage it to write cleaner and more maintainable code in your Java projects. Happy coding!