Maximizing Jenkins API Integration for Seamless Automation
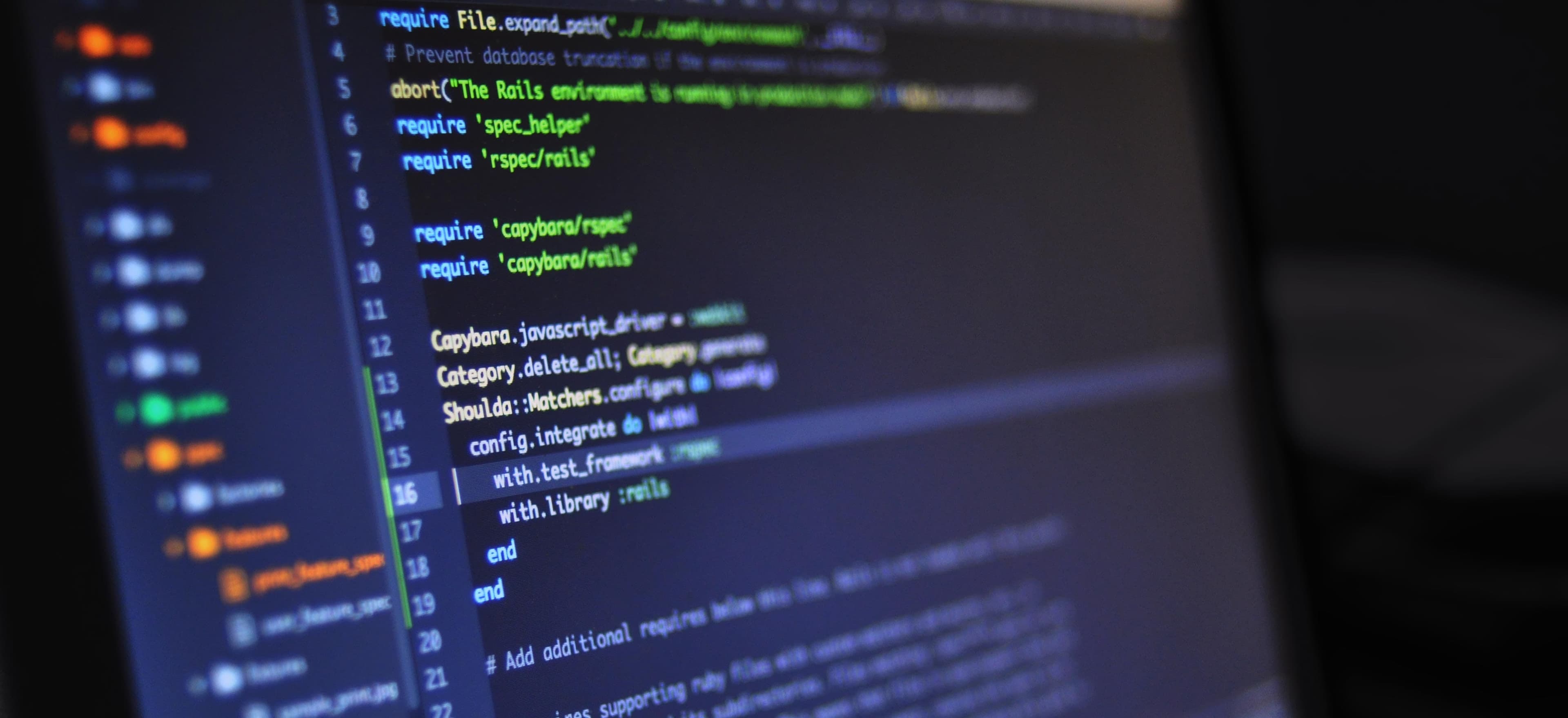
- Published on
Maximizing Jenkins API Integration for Seamless Automation
In the realm of continuous integration and delivery, Jenkins has emerged as a powerful automation tool. However, to truly harness its potential, leveraging its API is essential. In this blog post, we will delve into the intricacies of Jenkins API integration using Java, exploring how it can be optimized for seamless automation.
Understanding Jenkins API
Jenkins provides a rich set of APIs that allow for interaction with its core functionalities. These APIs enable developers to create, update, and manage jobs, as well as trigger builds and retrieve build results programmatically.
Setting Up the Jenkins API Client in Java
To begin, let's set up a Java client to interact with the Jenkins API. We can utilize the jenkins-client-java
library, which provides a convenient way to make HTTP requests to the Jenkins server.
import com.offbytwo.jenkins.JenkinsServer;
import com.offbytwo.jenkins.model.*;
public class JenkinsAPIClient {
private static final String JENKINS_URL = "http://localhost:8080";
private static final String USERNAME = "your_username";
private static final String API_TOKEN = "your_api_token";
public static void main(String[] args) {
JenkinsServer jenkins = new JenkinsServer(new URI(JENKINS_URL), USERNAME, API_TOKEN);
// Perform API operations here
}
}
Here, we initialize the JenkinsServer
object with the Jenkins server URL, username, and API token. This sets the foundation for making API requests to the Jenkins server.
Retrieving Job Information
One of the fundamental operations when integrating with the Jenkins API is retrieving job information. Let's initiate a request to fetch details about a specific job.
public void getJobDetails(String jobName) throws IOException {
JobWithDetails job = jenkins.getJob(jobName);
System.out.println("Job Name: " + job.getName());
System.out.println("Last Build Number: " + job.getLastBuild().getNumber());
// Additional job details can be accessed here
}
In this snippet, we obtain the JobWithDetails
object for a specific job and display basic details such as the job name and the last build number. This illustrates the simplicity of accessing job information through the Jenkins API.
Triggering a Build
Automation is at the core of Jenkins, and programmatically triggering a build is a pivotal capability. Let's explore how to initiate a build for a particular job using the API.
public void triggerBuild(String jobName) throws IOException {
jenkins.getJob(jobName).build();
System.out.println("Build triggered successfully for job: " + jobName);
}
By invoking the build
method on the job object, we can seamlessly trigger a new build for the specified job.
Customizing Build Parameters
Many Jenkins jobs require parameters for customization, such as branch names or build configurations. The API enables us to dynamically set these parameters when triggering a build.
public void triggerBuildWithParameters(String jobName, Map<String, String> parameters) throws IOException {
jenkins.getJob(jobName).build(parameters);
System.out.println("Build triggered successfully with parameters for job: " + jobName);
}
Here, we pass a Map
of parameter names and values to the build
method, allowing for flexible customization of build parameters during automation.
Retrieving Build Results
After triggering a build, it's often necessary to retrieve the build results for further analysis or reporting. Let's see how we can obtain build details using the Jenkins API.
public void getBuildResult(String jobName, int buildNumber) throws IOException {
Build build = jenkins.getJob(jobName).getBuildByNumber(buildNumber);
System.out.println("Build Status: " + build.details().getResult());
// Additional build details can be accessed here
}
In this code snippet, we fetch the build object by its number and extract crucial details such as the build status. This demonstrates the seamless process of accessing build results through the Jenkins API.
Making the Most of Jenkins API Integration
While the examples above provide a glimpse into Jenkins API integration, it's essential to consider best practices for optimizing this integration:
-
Error Handling: Incorporate robust error handling to gracefully manage failures when interacting with the Jenkins API. This ensures the reliability of automation workflows.
-
Security Considerations: Safeguard sensitive information such as API tokens and credentials by utilizing secure storage and transmission mechanisms, adhering to security best practices.
-
Testing and Validation: Thoroughly test the API integration components and validate their behavior across different Jenkins environments to ensure consistency and reliability.
-
Version Compatibility: Stay updated with the latest Jenkins API version and align the integration to accommodate any changes or deprecations in subsequent releases.
The Bottom Line
Jenkins API integration opens doors to streamlined automation and orchestration, empowering teams to amplify their CI/CD pipelines. By leveraging the capabilities of the Jenkins API through Java, developers can architect robust and efficient automation solutions.
Incorporating error handling, adhering to security best practices, and staying abreast of API version updates are pivotal in maximizing the potential of Jenkins API integration, fostering a culture of seamless automation.
In conclusion, harnessing the full power of Jenkins API integration in Java prompts a paradigm shift towards agile, scalable, and resilient automated workflows.
To deepen your understanding of Jenkins API integration, I recommend exploring the official Jenkins API Documentation and Jenkins Java API Client for further insights and resources.
Remember, the convergence of Jenkins and Java paves the way for unparalleled automation prowess, propelling software delivery to new heights.