Mastering Java Concurrency: Handling Condition Waits Effectively
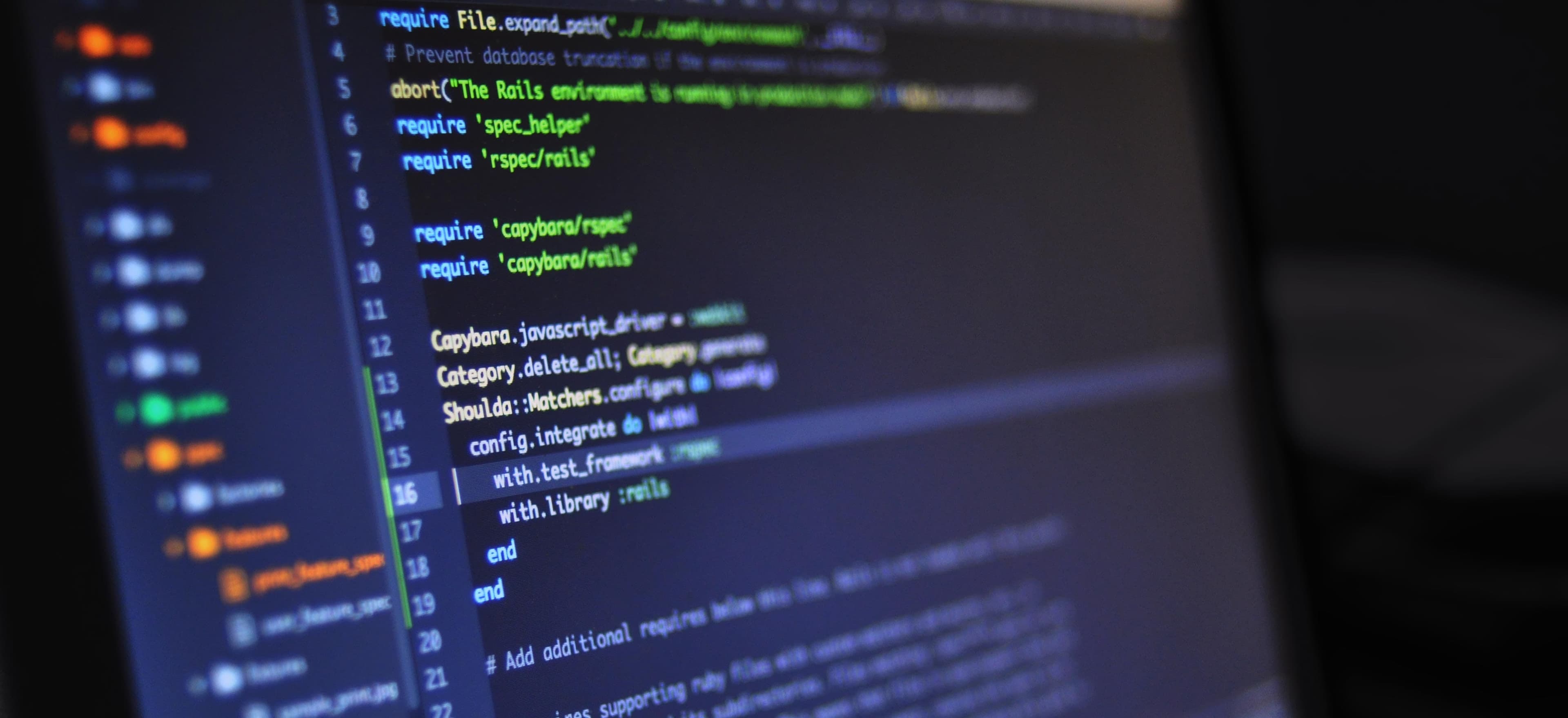
- Published on
Mastering Java Concurrency: Handling Condition Waits Effectively
Java is a robust programming language well-known for its concurrency utilities that allow developers to build highly efficient applications. Among these utilities, handling condition waits is a fundamental skill. In this blog post, we will explore how to effectively manage condition waits in Java, ensuring your applications can efficiently handle multiple threads in a synchronized manner.
Understanding Concurrency in Java
Concurrency is the ability of a program to execute multiple threads simultaneously. Threads are essentially lightweight processes that share the same resources but execute independently. Java provides built-in support for concurrent programming through the java.util.concurrent
package, which includes various utilities such as locks, condition variables, and executors.
For a deeper insight into Java concurrency, consider checking Java Concurrency in Practice, a classic resource.
What are Condition Waits?
Condition waits are used when you want a thread to pause its execution until a specific condition is met. This is crucial in scenarios where resources may not be available, and a thread needs to wait for notification from another thread.
In Java, the Object
class provides built-in methods for this purpose:
wait()
: Causes the current thread to wait until it is notified.notify()
: Wakes up a single thread that is waiting on this object's monitor.notifyAll()
: Wakes up all threads that are waiting on this object's monitor.
However, these methods should be used with caution. It's essential to work within a synchronized context to avoid issues.
Using Condition Variables
While you can directly use wait()
and notify()
methods for concurrency control, Java's java.util.concurrent
package introduces condition variables through ReentrantLock
. The advantage of using condition variables is that they provide a more flexible waiting mechanism compared to simple monitor locks. The condition object can also be easily separated from the lock that guards the shared resource.
Here's a simple example demonstrating how to use Condition variables:
Example Code
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class ConditionWaitExample {
private final Lock lock = new ReentrantLock();
private final Condition condition = lock.newCondition();
private int sharedResource = 0;
// Producer method
public void produce() throws InterruptedException {
lock.lock();
try {
while (sharedResource >= 5) {
condition.await(); // Wait until resource is available
}
sharedResource++;
System.out.println("Produced: " + sharedResource);
condition.signalAll(); // Notify that the resource is now available
} finally {
lock.unlock();
}
}
// Consumer method
public void consume() throws InterruptedException {
lock.lock();
try {
while (sharedResource <= 0) {
condition.await(); // Wait until the resource is produced
}
sharedResource--;
System.out.println("Consumed: " + sharedResource);
condition.signalAll(); // Notify that the resource has been consumed
} finally {
lock.unlock();
}
}
}
Commentary on the Code
- Synchronization: The
Lock
object ensures that only one thread can execute the critical section at a time, preventing race conditions. - Condition Object: The
Condition
variable allows threads to wait and signal each other without requiring the entire lock to be held at all times. This makes your application more efficient. - Awaiting and Signaling:
condition.await()
puts the current thread in a waiting state, releasing the lock it holds. After the resource is produced or consumed,condition.signalAll()
wakes up waiting threads, allowing them to proceed.
Implementing Producer-Consumer Problem
The Producer-Consumer problem is a classic example of using condition waits. It shows how threads can communicate effectively using shared resources while ensuring thread safety.
Complete Example
Here, we’ll illustrate both producing and consuming actions in a bounded buffer:
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class BoundedBuffer {
private final int[] buffer;
private int count, head, tail;
private final Lock lock = new ReentrantLock();
private final Condition notEmpty = lock.newCondition();
private final Condition notFull = lock.newCondition();
public BoundedBuffer(int size) {
buffer = new int[size];
}
public void put(int value) throws InterruptedException {
lock.lock();
try {
while (count == buffer.length) {
notFull.await(); // Buffer is full; wait to insert
}
buffer[tail] = value;
tail = (tail + 1) % buffer.length;
count++;
notEmpty.signal(); // Signal that there is a new item
} finally {
lock.unlock();
}
}
public int take() throws InterruptedException {
lock.lock();
try {
while (count == 0) {
notEmpty.await(); // Buffer is empty; wait to take
}
int value = buffer[head];
head = (head + 1) % buffer.length;
count--;
notFull.signal(); // Signal that there is space in buffer
return value;
} finally {
lock.unlock();
}
}
}
Key Takeaways
- Bounded Buffer: This implementation introduces a circular buffer with a maximum size and handles the logic for putting and taking values while managing concurrency.
- Thread Awareness: When the buffer is full, producers must wait, and when it’s empty, consumers must wait. This illustrates effective thread coordination.
- Efficient Resource Management: By signaling only when necessary (
notFull.signal()
andnotEmpty.signal()
), the application can avoid unnecessary wake-ups, thus optimizing performance.
In Conclusion, Here is What Matters
Mastering condition waits and effective use of concurrency tools like locks and conditions is essential for Java developers seeking to build responsive applications. The ability to manage multiple threads efficiently allows for better resource utilization and can significantly enhance the performance of your applications.
Remember, concurrency programming requires careful attention to detail, as mishandling waits and signals can lead to performance issues or deadlocks. For further reading on advanced Java concurrency concepts, the Official Java Documentation is an invaluable resource.
Embrace these concurrency patterns in your projects to unlock the full potential of Java, ensuring your applications are not only functional but also performant. Happy coding!