Revolutionize JUnit Testing: Embrace Jasmine's Approach!
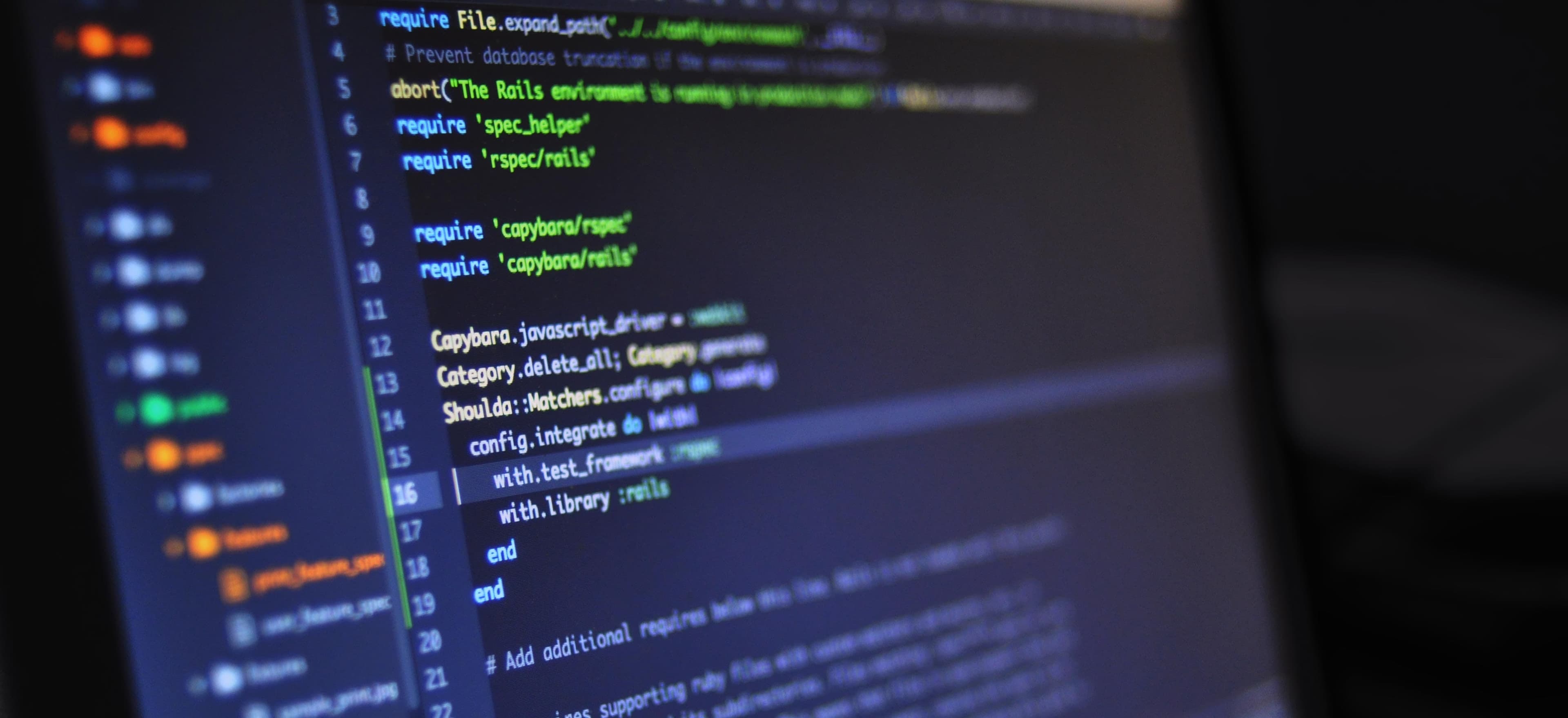
- Published on
Revolutionize JUnit Testing: Embrace Jasmine's Approach!
JUnit has long stood as the standard framework for unit testing in Java applications. However, as the software development landscape evolves, so too should our testing practices. In this blog post, we will explore how the principles and approaches derived from Jasmine, a popular JavaScript testing framework, can enhance JUnit testing.
Understanding JUnit and Jasmine
JUnit is a widely used framework in the Java ecosystem, providing tools and annotations for creating and running tests with ease. It focuses heavily on Java code and is best known for its simple constructs such as @Test
, @Before
, and @After
.
Jasmine, on the other hand, is designed for testing JavaScript code. It emphasizes behavior-driven development (BDD) principles, allowing developers to express their intentions clearly. While these two frameworks serve similar purposes, they embody different philosophies.
Why Look to Jasmine for Inspiration?
- Readable Syntax: Jasmine encourages a clearer, narrative style of test writing, making tests easier to read and understand.
- Descriptive Testing: Jasmine's
describe
andit
methods provide context to tests, leading to better documentation. - Mocking and Spying: Jasmine offers powerful built-in functions for creating spies and mocks. This allows for more controlled and isolated testing scenarios.
Adopting Jasmine Principles in JUnit
Let's delve into how we can introduce Jasmine's approach into JUnit testing. Below are a few core concepts that can transform your testing experience.
1. Descriptive Test Methods
JUnit tests often appear monotonous with standard naming conventions. Jasmine tests, however, use expressive names that describe the behavior under test.
JUnit Example:
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAdd() {
// test implementation
}
}
Improved JUnit Example:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void shouldReturnSum_WhenAddingTwoNumbers() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
Why this matters: Descriptive method names serve as self-documenting code, making it simpler for anyone who reads your tests to understand their purpose.
2. Organizing Tests with @Before
and @After
In Jasmine, tests are grouped using describe
blocks. Similarly, you can emulate this in JUnit with @Before
and @After
annotations to set up and tear down your test environment.
JUnit Example:
import org.junit.Before;
import org.junit.After;
import org.junit.Test;
public class UserServiceTest {
private UserService userService;
@Before
public void setUp() {
userService = new UserService();
}
@After
public void tearDown() {
userService = null;
}
@Test
public void testGetUser() {
// test implementation
}
}
Why this matters: This organizational structure improves readability and minimizes code duplication, making tests cleaner and more manageable.
3. Mocking and Spying
One of the standout features of Jasmine is its ability to create spies, allowing developers to track function calls and their arguments. In Java, libraries like Mockito provide similar functionalities.
JUnit Example with Mockito:
import static org.mockito.Mockito.*;
public class UserServiceTest {
@Test
public void testGetUserCallsUserRepository() {
UserRepository mockUserRepository = mock(UserRepository.class);
UserService userService = new UserService(mockUserRepository);
userService.getUserById(1);
verify(mockUserRepository).findById(1);
}
}
Why this matters: Mocking dependencies not only isolates tests, but also ensures that unit tests are focused and do not rely on the external state which could lead to flakiness.
4. Fluent Assertion APIs
Jasmine includes fluent assertions that can chain methods together, making it possible to create concise and expressive tests. Libraries like AssertJ can elevate JUnit tests to a similar level of fluency.
JUnit Example with AssertJ:
import static org.assertj.core.api.Assertions.assertThat;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertThat(result)
.isEqualTo(5)
.as("Check result of addition"); // Intent clarified
}
}
Why this matters: Enhanced readability makes it easier for other developers to follow your tests, supporting effective collaboration.
Maintaining a Continuous Testing Culture
Embracing Jasmine's principles in JUnit creates an opportunity to cultivate a continuous testing culture in your development team. Here are some tools and practices to maintain this culture:
- Continuous Integration (CI): Set up CI pipelines that automatically run JUnit tests after each commit. Tools like Jenkins, GitHub Actions, and CircleCI enable seamless integration.
- Code Review Processes: Encourage team members to review test cases as part of the code review process. This can drive a shared understanding of effective testing practices.
- Regular Refactoring: Just like production code, tests require maintenance. Regularly analyze test code to improve clarity and leverage new approaches.
My Closing Thoughts on the Matter
JUnit has served us well for unit testing Java applications, but there's always room for improvement. By incorporating Jasmine's methodologies into your JUnit tests, you can create a more readable, maintainable, and effective testing environment.
For more in-depth reading on improving testing practices, consider exploring the official JUnit documentation here or check out Mockito here.
By embracing and adapting best practices from different frameworks, we position ourselves for success in a rapidly evolving field. Start transforming your JUnit tests today by implementing these strategies!