Understanding Stack Trace Mysteries in Debugging
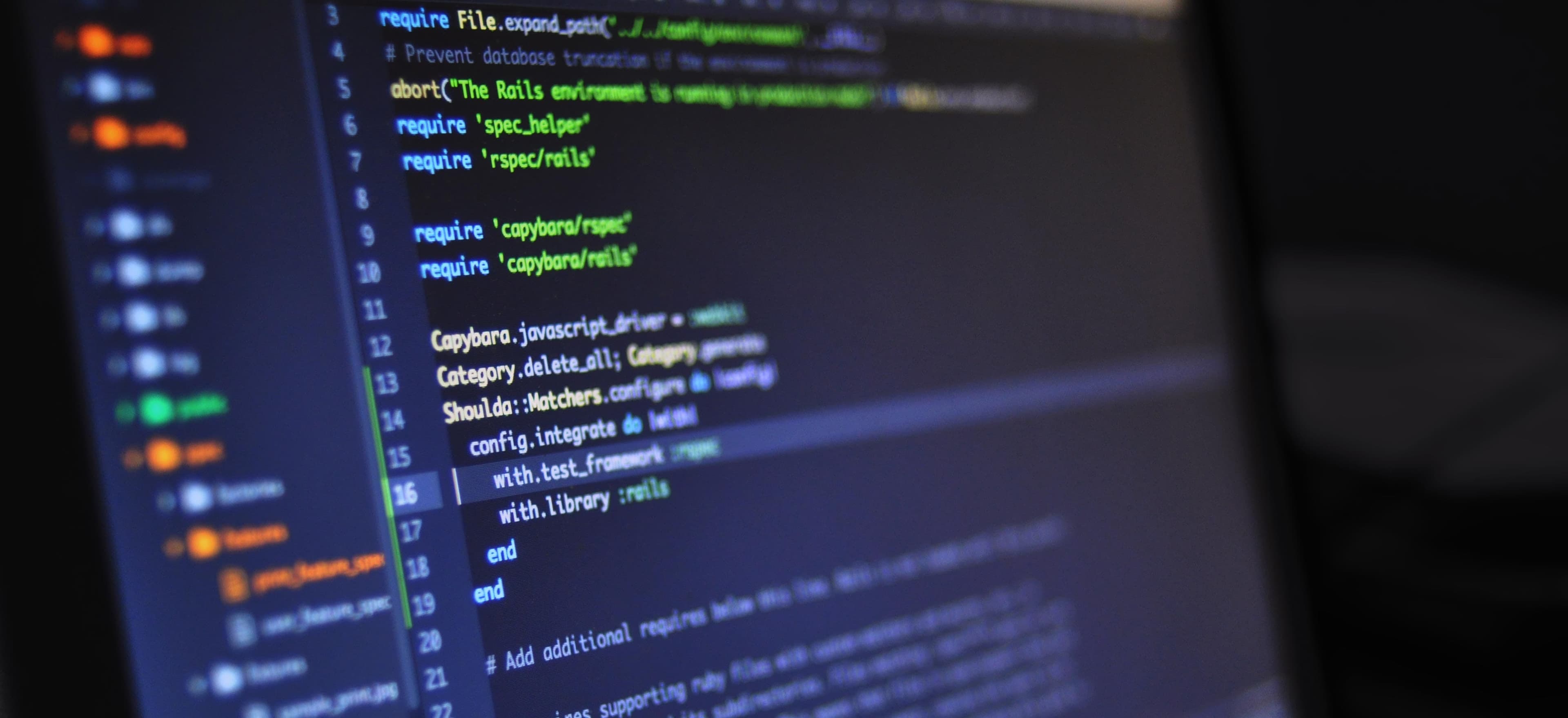
- Published on
Understanding Stack Trace Mysteries in Debugging
Debugging is a critical skill for any software engineer, and understanding stack traces is an essential part of that process. In Java, a stack trace provides valuable information that can help you pinpoint the source of an error. In this blog post, we will explore how stack traces work, how to read them, and how to use that information effectively to debug your Java applications.
What is a Stack Trace?
A stack trace is a report that contains information about the active stack frames at a certain point in time during the execution of a program. When an exception occurs in Java, the Java Virtual Machine (JVM) captures the stack trace and prints it to the console (or logs it depending on your configuration).
A typical stack trace includes several key components:
- Exception Type: This denotes the type of exception thrown (e.g.,
NullPointerException
,ArrayIndexOutOfBoundsException
). - Message: A description providing some context about what went wrong.
- Stack Frames: A list of method calls that were active at the time of the exception. Each frame shows the class name, method name, and the line number where the call occurred.
When is a Stack Trace Generated?
A stack trace is generated when an exception is thrown and not caught. Consider the following simple Java code, which will produce a stack trace when executed:
public class StackTraceExample {
public static void main(String[] args) {
try {
faultyMethod();
} catch (Exception e) {
e.printStackTrace(); // prints the stack trace to standard error
}
}
public static void faultyMethod() {
int[] numbers = {1, 2, 3};
// This will throw ArrayIndexOutOfBoundsException
System.out.println(numbers[5]);
}
}
Explanation of the Code
- In the
main
method, we callfaultyMethod()
wrapped in a try-catch block to handle the exception gracefully. - Inside
faultyMethod()
, we try to access an index that is out of bounds of the array, which leads to anArrayIndexOutOfBoundsException
. - The method
printStackTrace()
of the caught exception object is invoked, which displays the exception type, message, and stack trace to the console.
Running this program will output:
java.lang.ArrayIndexOutOfBoundsException: Index 5 out of bounds for length 3
at StackTraceExample.faultyMethod(StackTraceExample.java:9)
at StackTraceExample.main(StackTraceExample.java:5)
Here, you can see the exception type and message clearly indicating what went wrong, along with the stack frames.
Reading Stack Traces
Understanding how to read a stack trace is crucial for effective debugging. The order of stack frames is from the most recent to the oldest, with the first line indicating the origin of the exception.
- Identify the Exception Type: This gives you a clue about what the issue is. Knowing that you have a
NullPointerException
orClassCastException
, for instance, helps narrow down your search. - Follow the Stack Frames: Each stack frame indicates where the error occurred, starting from the method that raised the exception until the entry point of the thread.
- Check the Line Numbers: The line numbers are especially helpful in pinpointing the precise location in your code that caused the problem.
Common Java Exceptions and Their Causes
When debugging, you might encounter various exceptions. Here are some common ones:
NullPointerException
: Occurs when you try to access an object or call a method on a null reference.ClassCastException
: Raised when you attempt to cast an object of one type to another incompatible type.IndexOutOfBoundsException
: Happens when accessing elements in an array or collection that are outside its bounds.
Understanding these exceptions' common causes will prepare you for troubleshooting.
Using Stack Traces to Your Advantage
Let’s dive deeper into how to leverage stack traces to solve problems:
1. Analyze the Exception Type
Look closely at the type of exception. For example, a FileNotFoundException
suggests an issue with file paths. You might need to verify the file's existence and path correctness.
2. Trace Back the Call Hierarchy
Follow the flow backward through the stack frames. Each method needs to be checked for potential issues that might have led to the exception.
For instance, if you find that the exception occurred in a utility method, check how that method is invoked and with what parameters.
3. Test Hypotheses
Once you've formed hypotheses based on your analysis, test your ideas by modifying the variables, adding print statements, or using a debugger. This is an iterative process and may take multiple cycles to arrive at a solution.
Example: Debugging a NullPointerException
Consider a method that processes a user's profile:
public void processUserProfile(User user) {
System.out.println(user.getName()); // Might throw NullPointerException if user is null
}
If a NullPointerException
occurs here, you would check:
- Ensure that the
user
object isn't null before calling methods on it. - Investigate who calls
processUserProfile
and ensure they always pass a validUser
object.
You could modify the method to handle null gracefully:
public void processUserProfile(User user) {
if (user == null) {
System.out.println("Cannot process profile: User is null.");
return;
}
System.out.println(user.getName());
}
4. Utilize Logging
Instead of merely printing the stack trace using e.printStackTrace()
, incorporate a logging framework like SLF4J or Log4j. Logging gives you more control over output format, level, and destination (e.g., files, console).
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserProfileProcessor {
private static final Logger logger = LoggerFactory.getLogger(UserProfileProcessor.class);
public void processUserProfile(User user) {
if (user == null) {
logger.warn("User object is null.");
return;
}
logger.info("Processing user: {}", user.getName());
}
}
5. Use Your IDE's Debugger
Modern IDEs like IntelliJ IDEA or Eclipse come with powerful debugging tools. Utilizing these allows you to set breakpoints, step through code, and inspect variables dynamically. It's a significant advantage over only relying on stack traces.
Bringing It All Together
Understanding Java stack traces is indispensable for any developer. It serves as your first source of information when something goes wrong. By analyzing the exception type, deciphering the stack frames, and employing thoughtful debugging techniques, you will enhance your ability to resolve issues efficiently.
Remember to practice regularly, familiarize yourself with different exceptions, and continuously seek to improve your debugging skills.
For further reading, you can check out Java Exception Handling to understand how Java handles exceptions within its framework and Effective Java for broader insights into best practices in Java programming.
Happy coding!