Fixing Common Issues with Missing Parameterized Tests
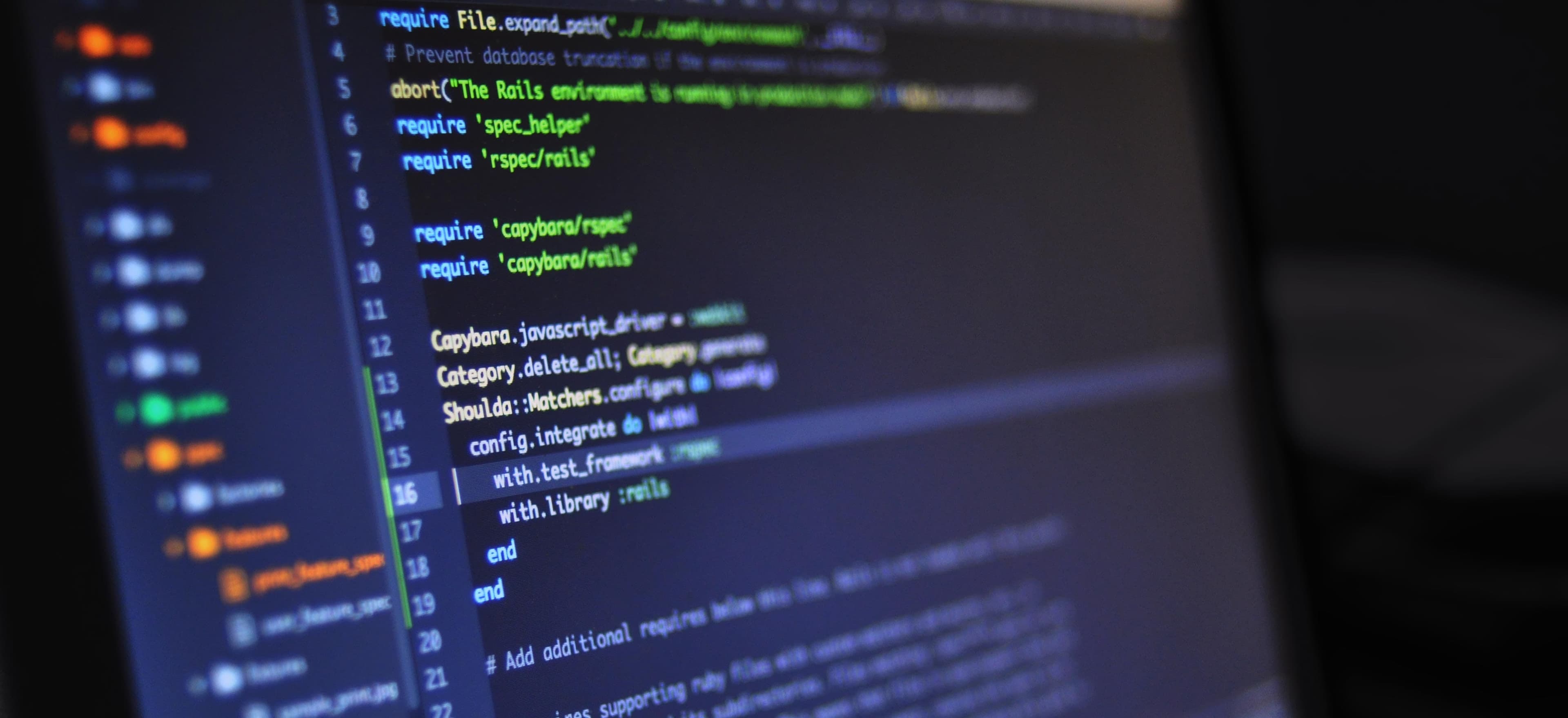
- Published on
Fixing Common Issues with Missing Parameterized Tests in Java
In the realm of software development, testing is an indispensable phase that ensures your application behaves as expected. In Java, one of the most powerful tools for writing tests is JUnit, particularly the Parameterized Test feature. However, developers often encounter pitfalls associated with missing parameterized tests, which can lead to unreliable or incomplete test coverage.
This blog post will explore common issues related to missing parameterized tests in Java and how to resolve them effectively. By understanding the importance and implementation of parameterized tests, you can enhance your testing strategy and ensure that your code remains robust.
Understanding Parameterized Tests
A parameterized test allows you to run the same test multiple times with different inputs. This capability is essential when you need to validate your code against various scenarios without duplicating code.
The primary advantages of using parameterized tests include:
- Code Reusability: Reduce redundancy by avoiding repetitive test cases.
- Clarity: Easily observe how your code behaves across different inputs.
- Efficiency: Reduce the time taken to write and maintain tests.
Basic Example of a Parameterized Test
To get started with a simple example, consider the following code snippet that demonstrates how to create a parameterized test in JUnit 5.
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class ParameterizedTestExample {
@ParameterizedTest
@ValueSource(strings = { "hello", "world", "JUnit", "5" })
void testStringNotEmpty(String input) {
assertTrue(!input.isEmpty(), "Expected non-empty string");
}
}
Commentary on the Code
In this example:
- @ParameterizedTest indicates that the method will be executed multiple times with different parameters.
- @ValueSource provides the inputs that will be passed to the test.
- The assertion checks that the input string is not empty.
This simple example showcases how parameterized tests can help you streamline testing with diverse inputs.
Common Issues with Missing Parameterized Tests
Despite the benefits of parameterized tests, some developers may overlook them. Here are some common issues that arise when parameterized tests are missing:
1. Lack of Diverse Test Cases
One of the most significant drawbacks of missing parameterized tests is the lack of diverse scenarios.
Fix: Create Parameterized Tests for Various Scenarios
When writing tests, consider every possible input your method might encounter. For instance, if you have a temperature conversion method, you should test negative temperatures, zero, and varying positive degrees.
Here’s how you might implement a parameterized test for a temperature conversion function:
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.CsvSource;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class TemperatureConverterTest {
@ParameterizedTest
@CsvSource({
"0, 32",
"100, 212",
"-40, -40",
"37, 98.6"
})
void testCelsiusToFahrenheit(double celsius, double expectedFahrenheit) {
assertEquals(expectedFahrenheit, convertToFahrenheit(celsius), 0.01);
}
double convertToFahrenheit(double celsius) {
return celsius * 9/5 + 32;
}
}
2. Difficulty in Tracking Bugs
When tests are not parameterized, pinpointing bugs becomes cumbersome. If similar tests exist for multiple inputs without using parameterization, a failure may provide little context on what went wrong.
Fix: Use Parameterized Tests to Isolate Test Cases
By employing parameterized tests, each input runs separately, allowing you to see failures more clearly.
3. Increased Maintenance Effort
Non-parameterized tests lead to increased maintenance as each input requires a separate test case.
Fix: Optimize with Parameterized Tests
Reduce maintenance efforts by consolidating multiple test cases into one parameterized test. This improvement not only simplifies your test structure but also enhances readability.
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.MethodSource;
import static org.junit.jupiter.api.Assertions.assertTrue;
public class EmailValidatorTest {
@ParameterizedTest
@MethodSource("emailProvider")
void testIsValidEmail(String email) {
assertTrue(isValidEmail(email));
}
static Stream<String> emailProvider() {
return Stream.of("test@example.com", "user.name+tag+sorting@example.com",
"user@example.co.in", "user@domain.com");
}
boolean isValidEmail(String email) {
// Basic email validation logic (to be implemented)
return email.contains("@") && email.contains(".");
}
}
Best Practices for Using Parameterized Tests
To make the most of parameterized tests, follow these best practices:
- Use Descriptive Test Names: Name your parameterized tests clearly to indicate what scenarios are being tested. JUnit allows you to customize the display name using the
name
attribute.
@ParameterizedTest(name = "{index} => input={0}, expected={1}")
@CsvSource({
"0, 0.0",
"1, 0.5",
"2, 1.0"
})
void testFactorial(int input, double expected) {
assertEquals(expected, factorial(input));
}
-
Group Related Tests: Use grouping to create hierarchy in your tests. Grouping similar tests makes your tests easier to manage and understand.
-
Document Edge Cases: Ensure to document edge cases clearly within your tests. This practice helps future developers understand what has been tested and why.
Closing Remarks
In the fast-evolving landscape of Java development, ensuring that your code is adequately tested is crucial. Parameterized tests provide a robust mechanism to validate your code against diverse inputs efficiently.
By understanding the common issues associated with missing parameterized tests and their solutions, you can significantly enhance your testing strategy. Implementing these practices will not only enhance code quality but also ease the maintenance burden and improve collaboration among developers.
For further exploration on unit testing in Java, check out JUnit 5 User Guide for comprehensive insights and advanced topics.
Your code deserves the best testing practices. Start using parameterized tests today, and witness your testing process transform into a more structured and effective routine. Happy coding!