Common Pitfalls When Using JUnit 5 Lifecycle Extensions
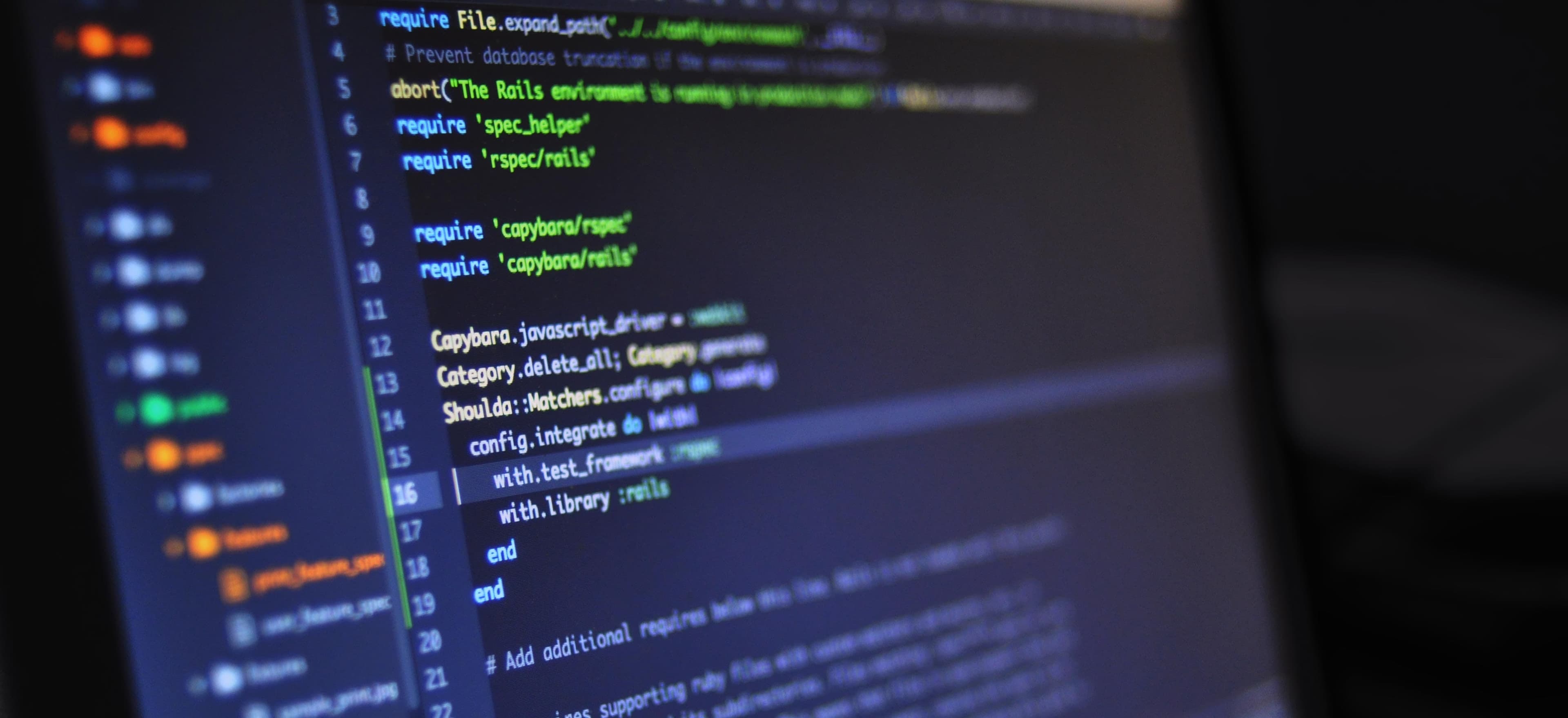
- Published on
Common Pitfalls When Using JUnit 5 Lifecycle Extensions
JUnit 5 has revolutionized the way we write tests in Java. Its architecture allows developers to structure their test cases better and create robust testing strategies with ease. One of the features that make JUnit 5 powerful is its lifecycle extensions, which lets users hook into various stages of the testing lifecycle. However, with great power comes great responsibility. In this blog post, we will discuss some common pitfalls developers encounter when using JUnit 5 lifecycle extensions and provide best practices to avoid them.
What Are JUnit 5 Lifecycle Extensions?
JUnit 5 lifecycle extensions allow you to define custom behavior before and after tests are executed. This can include setup and teardown operations that can be applied to both individual test methods and entire test classes. This flexibility means your tests can be cleaner and more maintainable.
Key Annotations
Before we dive into common pitfalls, let's quickly review some key lifecycle annotations:
@BeforeEach
: This method executes before each test method.@AfterEach
: This method executes after each test method.@BeforeAll
: This method executes once before all tests in a class are run.@AfterAll
: This method executes once after all tests in a class have been executed.
Example of Using Lifecycle Extensions
Here's a simple code snippet that demonstrates the use of these annotations:
import org.junit.jupiter.api.*;
public class LifecycleExample {
@BeforeAll
static void setupBeforeAll() {
System.out.println("Setting up system resources for all tests.");
}
@BeforeEach
void setupBeforeEach() {
System.out.println("Setting up before each test.");
}
@Test
void testOne() {
System.out.println("Running test one.");
Assertions.assertTrue(true);
}
@Test
void testTwo() {
System.out.println("Running test two.");
Assertions.assertTrue(true);
}
@AfterEach
void teardownAfterEach() {
System.out.println("Cleaning up after each test.");
}
@AfterAll
static void teardownAfterAll() {
System.out.println("Cleaning system resources after all tests.");
}
}
Why Use Lifecycle Extensions?
- Code Reusability: They allow common setup/teardown logic to be reused across multiple tests.
- Readability: They can make your tests cleaner and easier to understand.
- Performance:
@BeforeAll
and@AfterAll
can optimize performance by reducing setup/teardown overhead for expensive operations like opening a database connection.
Common Pitfalls
1. Misusing Static Annotations
One of the common mistakes is confusing the requirements of @BeforeAll
and @AfterAll
with those of @BeforeEach
and @AfterEach
.
Pitfall Explanation
@BeforeAll
and @AfterAll
must be declared as static methods. If you try to use them without declaring them static, you will encounter a TestInstanceException
.
Best Practice
Always mark these methods as static. Here's an example correction:
@BeforeAll
static void setup() {
// Initialization code
}
2. Forgetting the Lifecycle of Test Instances
JUnit 5 offers flexible test instance lifecycle management. Developers can use @TestInstance(Lifecycle.PER_CLASS)
to modify how instances are created. However, mishandling this can lead to inconsistencies.
Pitfall Explanation
Using @BeforeAll
or @AfterAll
in a class with Lifecycle.PER_METHOD
(the default) will cause @BeforeAll
and @AfterAll
not to execute.
Best Practice
Explicitly declare the test lifecycle in your test class to avoid misunderstandings:
@TestInstance(TestInstance.Lifecycle.PER_CLASS)
class MyTests {
@BeforeAll
void setup() {
// Setup code
}
}
3. Ignoring Exception Handling
Not handling exceptions properly in lifecycle methods can lead to unexpected behavior in your tests. If a setup method fails, it might not be apparent why other tests fail.
Pitfall Explanation
If an exception is thrown in a @BeforeEach
or @BeforeAll
method, it can cause your tests to skip unexpectedly.
Best Practice
Implement proper exception handling or logging in your lifecycle methods to know when and why a failure occurs:
@BeforeEach
void setup() {
try {
// setup code
} catch (Exception e) {
// Log the exception
e.printStackTrace();
throw e; // Optionally rethrow the exception if needed
}
}
4. Overusing Global State
Using shared mutable state across tests can lead to flaky tests and make your tests interdependent.
Pitfall Explanation
If you modify a global state in a @BeforeEach
or @BeforeAll
, it might affect subsequent tests, leading to unexpected results.
Best Practice
Always try to isolate tests. Use local variables within your methods or re-initialize globals in your lifecycle extensions.
private SomeSharedResource resource;
@BeforeEach
void setup() {
resource = new SomeSharedResource();
// Additional setup
}
5. Overcomplicating Lifecycle Methods
Another common pitfall is writing overly complex logic in lifecycle methods, which can lead to hard-to-maintain and obscure tests.
Pitfall Explanation
Complex lifecycle methods can make it hard to determine the flow of execution in test cases, leading to challenges in debugging.
Best Practice
Keep lifecycle methods focused and straightforward. Complex logic should be moved into helper methods to maintain clarity:
@BeforeEach
void setup() {
initializeCommonResources();
}
private void initializeCommonResources() {
// Logic to initialize resources
}
Helpful Resources
For additional knowledge about JUnit 5 lifecycle annotations and best practices, consider these resources:
Key Takeaways
JUnit 5 lifecycle extensions offer a formidable toolkit for managing your testing lifecycle. However, they bring with them complexities that can lead to pitfalls if not handled cautiously.
By recognizing these common issues and implementing the best practices discussed here, you can ensure that your tests are clean, maintainable, and effective. Adopt a disciplined approach to using lifecycle methods, and your testing strategy will yield significant benefits. Happy testing!