Elevating Switch Statements: Common Pitfalls to Avoid
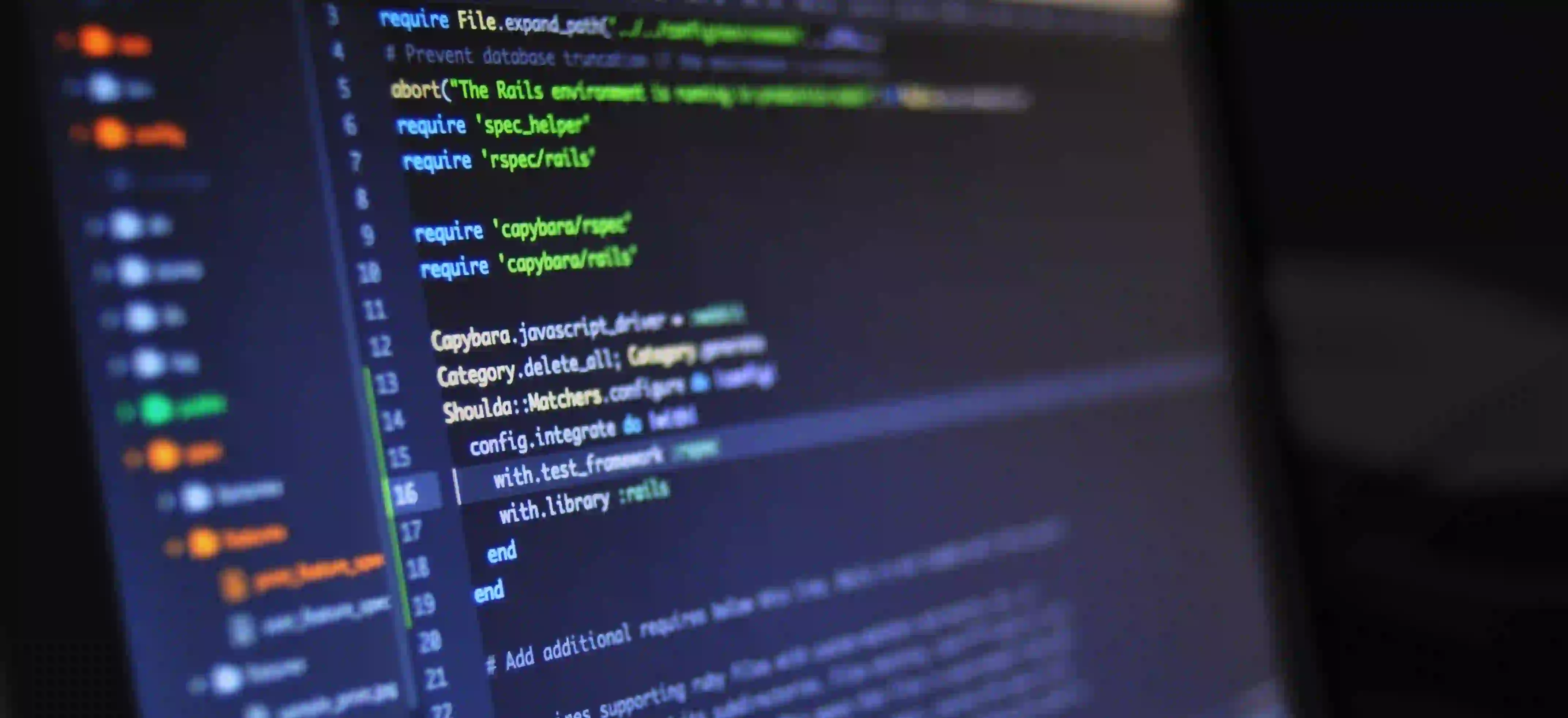
Elevating Switch Statements: Common Pitfalls to Avoid
Switch statements are a fundamental feature in many programming languages, including Java. They provide a way to execute different blocks of code based on the value of a variable. However, despite their utility, switch statements can lead to several pitfalls if not used correctly. In this blog post, we will explore common problems while using switch statements in Java and how to elevate your code by avoiding those pitfalls.
Understanding Switch Statements in Java
Before we dive into the common pitfalls, let's revisit how switch statements work in Java.
Basic Syntax
The switch statement evaluates a variable (often called the "switch expression") and executes the corresponding case block that matches the variable's value. If no cases match, an optional default block can be executed.
Here's a simple code example:
public class WeekdayExample {
public static void main(String[] args) {
int day = 3; // Let's say 3 represents Wednesday
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
case 5:
System.out.println("Friday");
break;
default:
System.out.println("Weekend");
break;
}
}
}
In this code snippet, a variable day
is evaluated. When day
equals 3, the program prints "Wednesday".
Pitfall 1: Forgetting Break Statements
One of the most common pitfalls when using switch statements is neglecting the break
statement. Without a break, execution will "fall through" and execute subsequent case blocks, which can produce unexpected results.
Code Example:
public class MistakeExample {
public static void main(String[] args) {
int day = 3;
switch (day) {
case 1:
System.out.println("Monday");
case 2:
System.out.println("Tuesday");
case 3:
System.out.println("Wednesday");
default:
System.out.println("Invalid Day");
}
}
}
Why This Is a Problem
In the code snippet above, forgetting break
statements means that if day
is 3, the program will also print "Invalid Day" after "Wednesday". Always using break
statements after each case is crucial to prevent fall-through behavior.
Solution:
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
default:
System.out.println("Invalid Day");
break;
}
Pitfall 2: Using Non-constant Expressions
Switch statements in Java only work with certain types of expressions such as integers, enumerated types, and strings. Using non-constant expressions will create compilation errors.
Code Example:
public class NonConstantExample {
public static void main(String[] args) {
final int value = 2;
switch (value + 1) { // Valid as long as the expression resolves to constant
case 2:
System.out.println("Two");
break;
default:
System.out.println("Not Two");
}
}
}
Why This Matters
While the example above works, using complex expressions or non-final variables can lead to unexpected errors. It is often recommended to design your code to use a single valid variable in a switch statement.
Pitfall 3: Modifying the Switch Variable Within the Statement
Another issue arises when you mistakenly modify the variable being switched within the switch statement.
Code Example:
public class ModifyInsideSwitch {
public static void main(String[] args) {
int day = 1;
switch (day) {
case 1:
day++;
System.out.println("Day incremented to " + day);
break;
case 2:
System.out.println("Today is Tuesday");
break;
default:
System.out.println("Invalid Day");
}
}
}
Consequences of This Mistake
In the example, day
is incremented, which can lead to confusion or unintended behavior. The case should relate to the original value, and modifications should be avoided.
Solution:
Keep the switch operation read-only regarding the switch variable. If necessary, create a new variable to hold any value changes.
int originalDay = day; // Create a separate variable
switch (originalDay) {
case 1:
System.out.println("Day is Monday");
break;
{ ... }
}
Pitfall 4: Overusing Switch for Complex Logic
While switch statements can make code cleaner in some scenarios, overusing them for complex logic can lead to unreadable code.
Example of Complex Logic:
public class ComplexSwitch {
public static void main(String[] args) {
int input = ...; // get input from somewhere
switch (input) {
case 1:
// lots of logic here
// ...
break;
case 2:
// other complex logic
break;
default:
// default case
break;
}
}
}
Why It Can Be a Problem
When the switch statement contains excessive logic, it can undermine the clarity and maintainability of your code. When logic becomes heavily nested or exceedingly broad, consider refactoring into methods instead.
Solution:
Refactor your complex cases into functions.
public void handleCase1() {
// Complex logic dedicated for case 1
}
public void handleCase2() {
// Complex logic dedicated for case 2
}
public void main(String[] args) {
int input = ...;
switch (input) {
case 1:
handleCase1();
break;
case 2:
handleCase2();
break;
default:
handleDefault();
break;
}
}
Pitfall 5: Not Considering Enum for Fixed Constants
If you are switching over a fixed set of constants, you can improve code readability and maintainability by utilizing enum
types instead.
Example:
public enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY
}
public class EnumSwitch {
public static void main(String[] args) {
Day day = Day.WEDNESDAY;
switch (day) {
case MONDAY:
System.out.println("It's Monday");
break;
case TUESDAY:
System.out.println("It's Tuesday");
break;
case WEDNESDAY:
System.out.println("It's Wednesday");
break;
// continue with the rest
}
}
}
Why Use Enum?
Using enums makes the code more readable and reduces the risk of errors when checking the values in the switch statement. This practice ensures type safety since enums are derived from a single type.
In Conclusion, Here is What Matters
In this blog post, we explored common pitfalls when using switch statements in Java, emphasizing how to avoid them for better, cleaner, and more maintainable code. By adhering to best practices, such as always utilizing break statements, avoiding modifications to the switch variable within the statement, and using enums for fixed constants, you enhance not only your coding skills but also the overall quality of your Java applications.
For further reading on this topic, visit Java Documentation or delve into effective Java for more insights on advanced programming practices.
Switch wisely!