Why Test Doubles Are Crucial in Refactoring
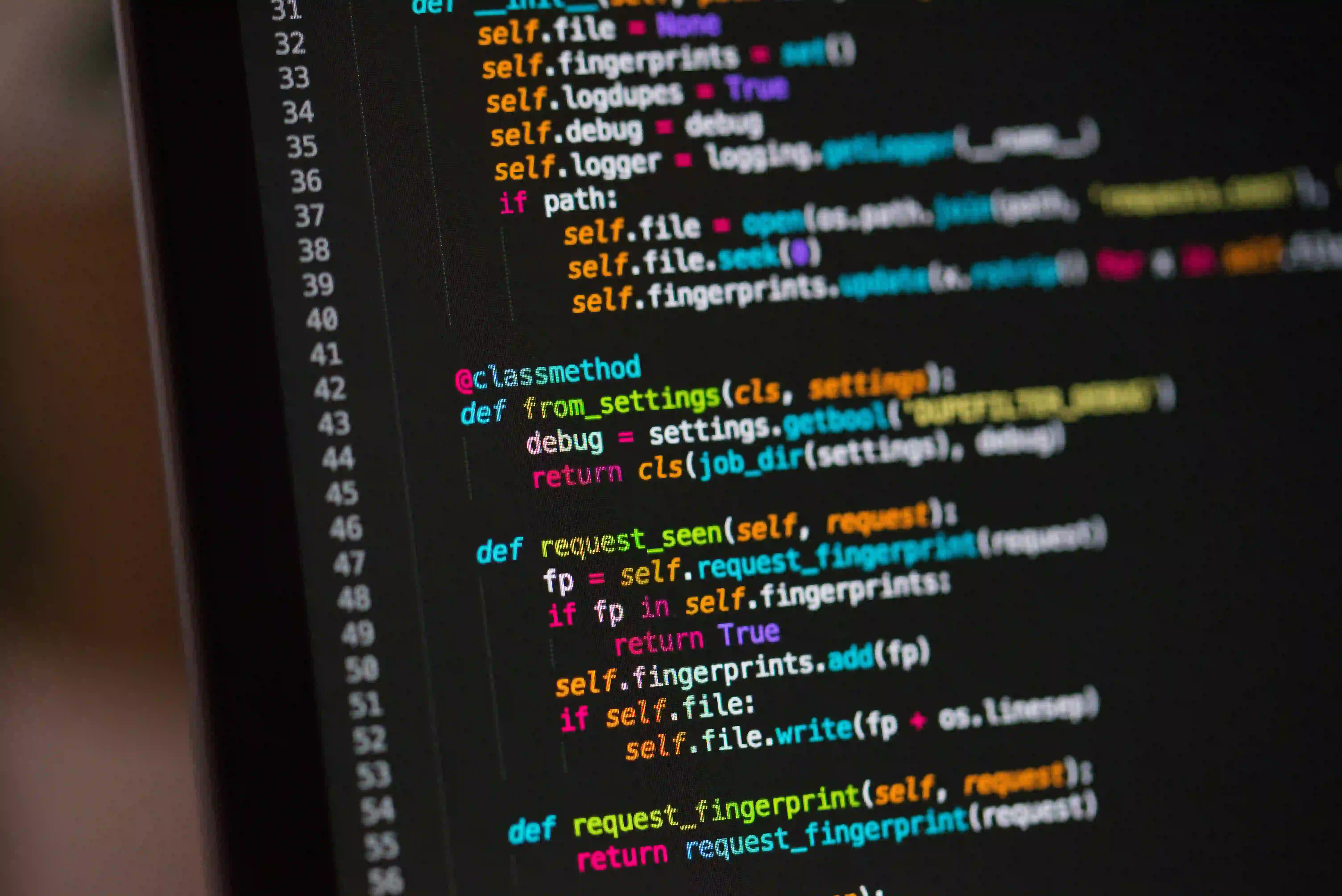
Why Test Doubles Are Crucial in Refactoring
In the world of software development, refactoring is a common practice aimed at improving the structure and quality of existing code. However, modern applications often have various interdependencies that can make this process daunting. Enter test doubles—powerful tools that can help you isolate and test components of your code without affecting others. In this post, we'll discuss the different types of test doubles, why they are essential during refactoring, and how to effectively implement them in your Java projects.
Understanding Test Doubles
Test doubles are simplified objects used in place of real components during testing. They allow developers to test specific parts of code while controlling the behavior of dependencies. There are four main types of test doubles:
-
Dummy: These are objects that are passed around but never actually used. They can fulfill parameter requirements but don’t provide meaningful functionality.
-
Fakes: Fakes have working implementations, but usually do not match the production code in complexity. They are easier to work with and can simulate some of the behavior of the component they replace.
-
Stubs: Stubs provide pre-defined responses to calls made during tests. They do not contain any real logic but return hard-coded data.
-
Mocks: Mocks are pre-programmed with expectations that form part of the test. If the expectations are not met, the test fails. They are especially useful for verifying interactions between components.
In this post, we will focus primarily on mocks and stubs, as they are particularly effective for refactoring.
Why Are Test Doubles Crucial for Refactoring?
Refactoring is not merely about changing code for the sake of change; rather, it involves improving the internal structure without altering its external behavior. Effective refactoring relies on ensuring that existing functionalities are maintained. This is where test doubles shine. Here are several reasons why they are essential during refactoring:
1. Isolation of Code
Test doubles allow you to isolate the code you are refactoring. By replacing dependencies with mocks or stubs, you can focus on the unit of work you want to improve. This isolation helps ensure that a change made in one part of the application doesn't inadvertently affect another.
2. Controlled Environments
When refactoring, you want to control your test environments. Test doubles let you simulate various scenarios—such as error cases or different data inputs—without changing the underlying dependencies. This means you can ensure your refactored code behaves correctly across a range of potential scenarios.
3. Faster Feedback Loop
Test doubles generally lead to faster tests. By using lightweight objects instead of full implementations, your tests run quicker. This immediacy accelerates the feedback loop and enhances developer productivity during refactoring.
4. Ease of Maintenance
Refactored code will often introduce new logic or patterns. By using test doubles, you can maintain clarity and specificity in your tests, making them easier to understand and maintain. This can greatly aid other developers who might need to work with your code in the future.
5. Encouraging TDD Practices
Test-driven development (TDD) encourages writing your tests before the actual implementation. Test doubles assist in this by allowing you to write tests first without needing to worry about the actual behaviors and dependencies, which might not be implemented yet.
Implementing Test Doubles in Java
To illustrate how test doubles can be utilized in Java, let's walk through a simple example. Suppose we have a service that retrieves user data from a database. Our initial implementation might look like this:
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(Long id) {
return userRepository.findById(id);
}
}
In this code, UserRepository
is a dependency we will need to handle when testing.
Creating a Stub
To test the UserService
without a real database, we can create a stub for the UserRepository
.
class UserRepositoryStub implements UserRepository {
@Override
public User findById(Long id) {
return new User(id, "John Doe"); // returning a dummy user
}
}
// Test case using the Stub
public class UserServiceTest {
@Test
public void testGetUserById() {
UserRepositoryStub userRepositoryStub = new UserRepositoryStub();
UserService userService = new UserService(userRepositoryStub);
User user = userService.getUserById(1L);
assertEquals("John Doe", user.getName());
}
}
Creating a Mock
If we want to ensure that our UserService
interacts with the UserRepository
correctly, we can use a mocking framework like Mockito.
import static org.mockito.Mockito.*;
public class UserServiceMockTest {
@Test
public void testGetUserById() {
UserRepository userRepositoryMock = mock(UserRepository.class);
when(userRepositoryMock.findById(2L)).thenReturn(new User(2L, "Jane Doe"));
UserService userService = new UserService(userRepositoryMock);
User user = userService.getUserById(2L);
assertEquals("Jane Doe", user.getName());
verify(userRepositoryMock).findById(2L); // Verifying interaction
}
}
Use Cases for Each Type
-
Stubs are often used when you want predictable responses, such as returning a fixed set of data.
-
Mocks are beneficial for checking if specific methods are invoked, or to enforce a certain order of calls.
By choosing the appropriate test double based on your specific needs during refactoring, you can retain the quality and maintainability of your code.
In Conclusion, Here is What Matters
Test doubles are indispensable allies in the refactoring process. They provide isolation, control, and feedback, enabling developers to improve their code confidently. Leveraging techniques like stubs and mocks in Java can significantly enhance your testing strategy and ensure that the underlying structure remains clean and optimized.
Remember, refactoring is a continuous journey. When done thoughtfully and supported by robust testing practices, your codebase will be easier to understand, maintain, and expand in the future.
Further Reading
Feel free to reach out with any questions regarding the implementation of test doubles or other refactoring techniques in Java. Happy coding!