Why Reflection Can Complicate Your Testing Process
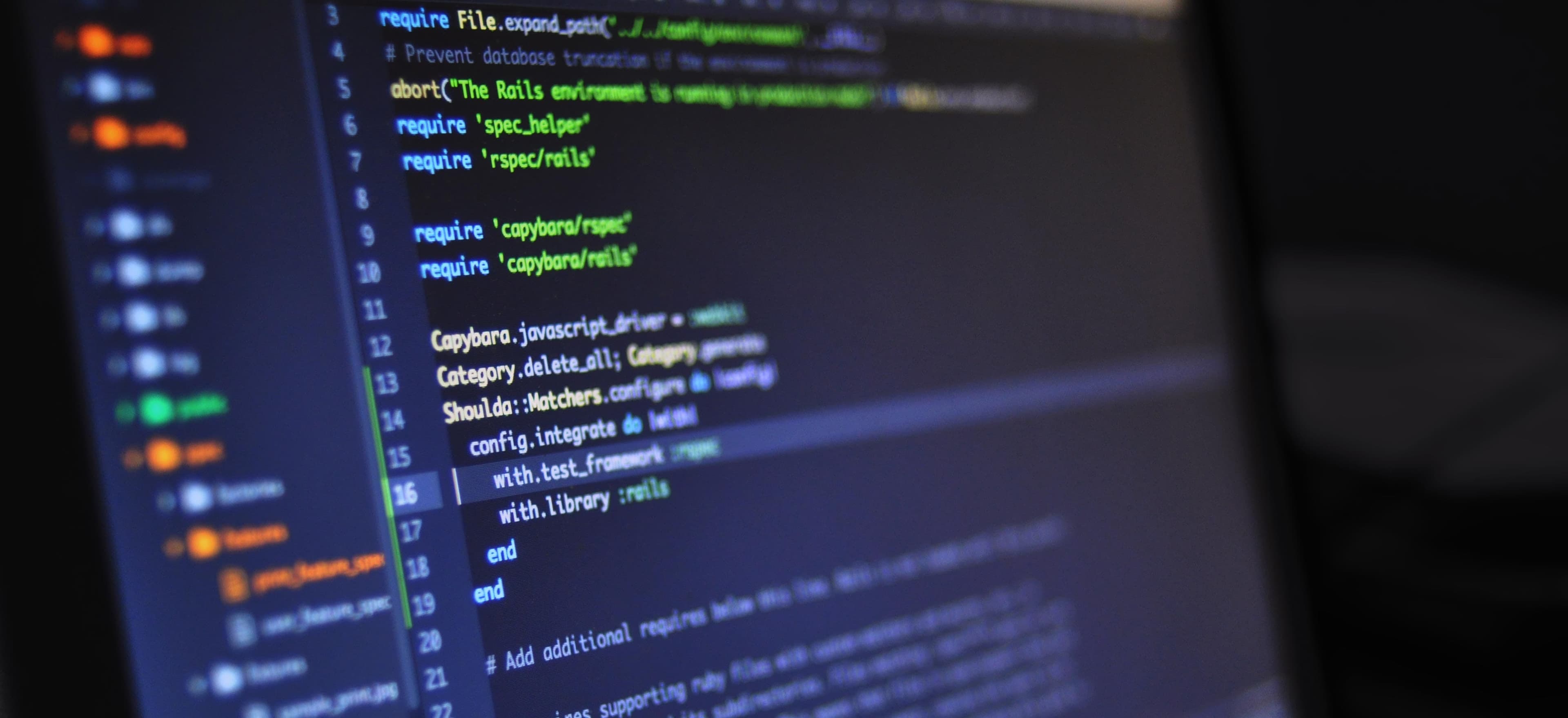
- Published on
Why Reflection Can Complicate Your Testing Process in Java
Reflection is a powerful feature in Java, allowing developers to inspect classes, methods, and fields at runtime. While it offers flexibility and can simplify certain tasks, it can also complicate the testing process. In this blog post, we’ll discuss the implications of using reflection in your Java applications, how it can hinder your testing efforts, and best practices to minimize these issues.
Understanding Reflection
Before diving into the complications that reflection brings to testing, it’s essential to clarify what reflection is and how it works.
Reflection in Java allows you to:
- Inspect Class Information: Check the class name, methods, fields, and even annotations.
- Access Private Members: Invoke private methods and access private fields, bypassing encapsulation.
- Create Objects at Runtime: Instantiate classes whose names are known only at runtime.
Here’s a simple example:
import java.lang.reflect.Method;
public class ReflectionExample {
private void secretMethod() {
System.out.println("This is a secret method");
}
public static void main(String[] args) throws Exception {
ReflectionExample example = new ReflectionExample();
Method method = ReflectionExample.class.getDeclaredMethod("secretMethod");
method.setAccessible(true); // Bypassing encapsulation
method.invoke(example); // Invoking the secret method
}
}
Why Use Reflection?
Java developers may leverage reflection for various reasons:
- Framework Development: Many modern frameworks use reflection (like Spring and Hibernate) to instantiate classes and inject dependencies.
- Dynamic Behavior: Applications that need dynamic behavior based on user input can use reflection to adapt to various conditions at runtime.
However, with great power comes great responsibility.
Complications of Using Reflection in Testing
Reflection can introduce various challenges during the testing phase of your application. Let’s look at the most prominent issues.
1. Lack of Compile-time Checks
With reflection, you lose the benefits of compile-time checks. This means potential errors in your code could go unnoticed until runtime. For example:
Method method = Example.class.getMethod("nonexistentMethod");
This would compile fine but throw a NoSuchMethodException
at runtime. Consequently, unit tests may not catch this error until a specific test case is executed.
2. Increased Complexity and Fragility
Reflection can lead to fragile code. If the structure of your class changes, your reflective code may break, leading to failing tests that seem unrelated.
Consider a scenario where a method name changes:
Method method = Example.class.getDeclaredMethod("oldMethodName");
If oldMethodName
was changed to newMethodName
, your tests that rely on reflection will fail without clear indication of the issue's root cause.
3. Performance Overhead
Reflection inherently comes with a performance cost. While this may not be a concern during single requests, it can accumulate significantly during unit tests, especially when running large test suites.
Unit tests should ideally be fast. The use of reflection can slow down the execution time, leading to longer feedback loops for the developers.
4. Testing Private Members
While one can use reflection to test private members, this practice often indicates that the code is poorly structured. Unit tests should ideally test the public behavior of a class. Relying on reflection to access private members can obscure the purpose of your tests and make them less meaningful.
Method privateMethod = MyClass.class.getDeclaredMethod("privateMethod");
privateMethod.setAccessible(true);
This code snippet demonstrates the ability to access a private method but raises the question: should you be testing that at all?
Best Practices to Mitigate Reflection Issues
Reflection does have its place, but it's vital to use it judiciously. Here are some best practices to minimize its complications during testing.
1. Limit Reflection in Production Code
Whenever possible, limit the use of reflection in your production code. If you find yourself needing reflection often, consider refactoring your design. Utilization of interfaces and design patterns like Dependency Injection can eliminate many reflective needs. The SOLID principles can guide you in structuring robust code bases.
2. Use Frameworks to Handle Reflection
If leveraging reflection is unavoidable, consider using established frameworks that manage reflection for you. Spring, for example, abstracts many complexities. When using Spring Framework, you can directly use annotations for class dependency injections, which may simplify tests as well.
@Component
public class MyService {
private final MyRepository myRepository;
@Autowired
public MyService(MyRepository myRepository) {
this.myRepository = myRepository;
}
}
3. Mock External Dependencies
If you must test classes using reflection, utilize mocking frameworks such as Mockito to mock external dependencies, allowing your tests to focus on logic rather than state.
when(mockedDependency.someMethod()).thenReturn(someValue);
By mocking, you can ensure that your tests remain focused, fast, and reliable.
4. Test Public APIs Only
Strive to test only the public API of your classes. This not only improves the clarity of your tests but also ensures that if changes happen to private methods, tests remain intact unless the public interface itself changes.
5. Utilize Code Coverage Tools
Finally, to track how well your tests cover your code, utilize code coverage tools like JaCoCo or Cobertura. These tools can help identify untested paths, including those using reflection.
Final Thoughts
Reflection is one of the many powerful tools available in Java, allowing for runtime class inspection and manipulation. However, while it offers compelling use cases, it can also complicate your testing process, introducing risks such as runtime errors, increased complexity, and performance issues.
When using reflection, adhere to best practices by limiting its use, leveraging robust frameworks, sticking to public APIs, and utilizing mocking frameworks. By doing so, you can enjoy the benefits of reflection while mitigating its potential challenges.
Reflect wisely, and your Java applications will remain robust and maintainable, even as your code evolves.
For more insights on Java best practices, consider reading Effective Java by Joshua Bloch or Clean Code by Robert C. Martin, both of which offer invaluable advice on writing better Java code.
Keep coding smart!