Uncovering Java Finalizer Pitfalls in File I/O Streams
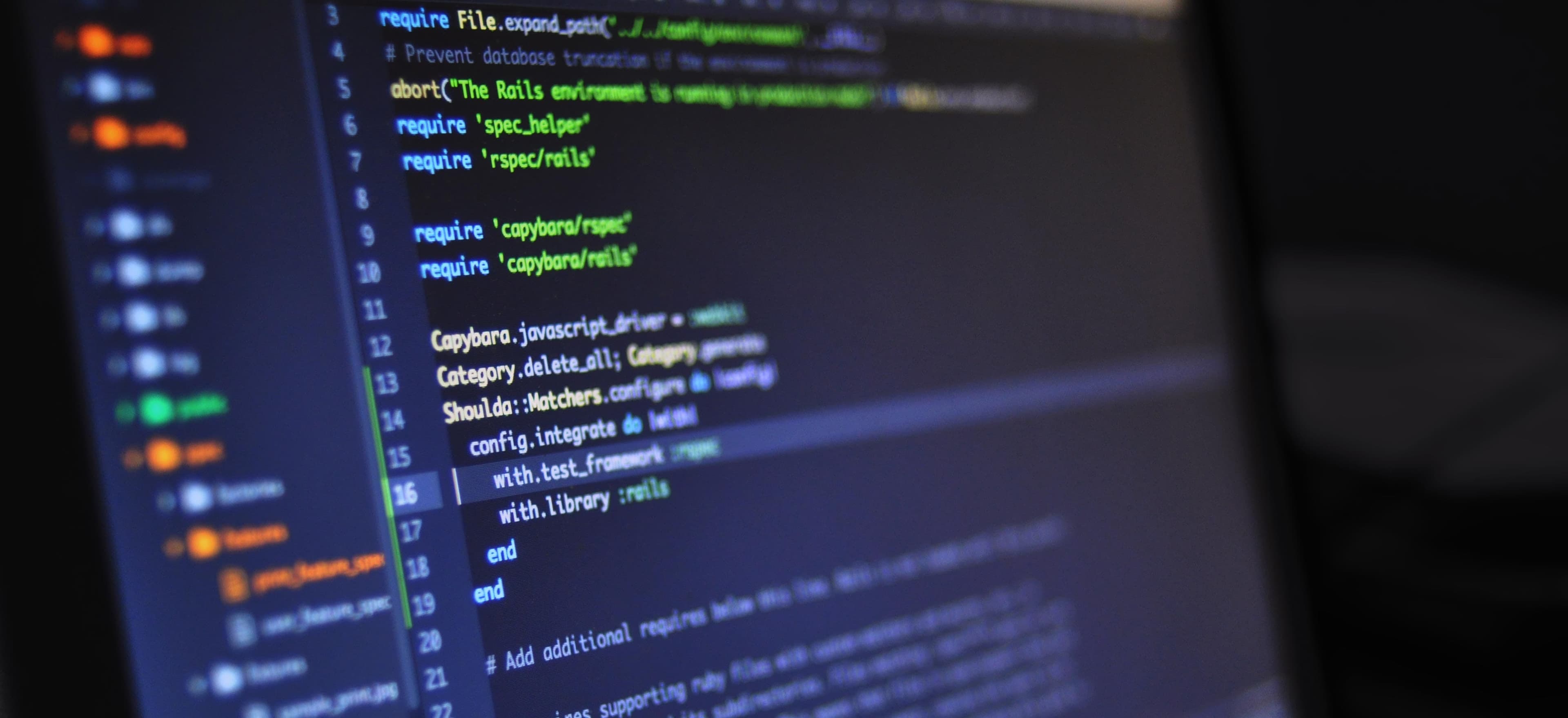
- Published on
Uncovering Java Finalizer Pitfalls in File I/O Streams
Java is a powerful programming language, renowned for its robustness, portability, and ease of use. However, like any language, it has its share of pitfalls and intricacies, especially when working with resources such as file input/output (I/O) streams. One of the most frequently misused areas is the finalizer mechanism. This post will delve into the issues associated with using finalizers in Java, particularly with file I/O streams, and suggest better alternatives.
Understanding Finalizers in Java
A finalizer is a special method in Java, finalize()
, that is called by the garbage collector on an object when there are no more references to it. The purpose of finalizers is to allow an object to perform cleanup before it is removed from memory.
Why Use Finalizers?
You may think that finalizers are a good safety net for cleaning up resources like file handles, socket connections, or database connections. The concept is straightforward: when your object is about to be garbage-collected, the finalizer will take care of closing resources that your object may be holding.
Here's a basic example:
public class FileReaderWithFinalizer {
private FileInputStream fileInputStream;
public FileReaderWithFinalizer(String filePath) throws FileNotFoundException {
this.fileInputStream = new FileInputStream(filePath);
}
@Override
protected void finalize() throws Throwable {
try {
if (fileInputStream != null) {
fileInputStream.close();
}
} finally {
super.finalize();
}
}
}
In this example, if the FileReaderWithFinalizer
object becomes unreachable, the finalize()
method attempts to close the FileInputStream
. However, this approach is prone to various pitfalls.
The Pitfalls of Using Finalizers
While the intention behind using finalizers may seem noble, the execution can lead to several issues:
1. Unpredictable Timing
The garbage collection process in Java is not deterministic. This means that there is no guarantee on when, or if, the finalizer will be invoked. Therefore, holding resources until the finalizer is called can lead to resource leaks.
2. Performance Overhead
Finalizers can affect performance. Every object with a finalizer must go through an additional garbage collection phase, known as the finalization phase, which can slow down performance significantly.
3. Potential Deadlocks
If a finalizer tries to access other resources (which are already held by another part of your application), it can potentially lead to deadlocks. This is especially relevant in multi-threaded environments.
4. Exception Handling
Exceptions thrown in finalizers are ignored by the Java runtime. This creates a complete lack of visibility into failures during the cleanup process, which can lead to unnoticed failures and resource leaks.
Best Practices for Resource Management in Java
Given the pitfalls associated with finalizers, the Java community recommends using other mechanisms for resource management. Here are more effective practices:
1. Using try-with-resources
Introduced in Java 7, the try-with-resources statement is a beautiful alternative for managing resources. It ensures that each resource is closed at the end of the statement, reducing the chances of leaks significantly.
public void readFile(String filePath) {
try (FileInputStream fileInputStream = new FileInputStream(filePath)) {
// Perform file read operations here
} catch (IOException e) {
e.printStackTrace();
}
}
Why use try-with-resources? It simplifies code and automatically closes resources when they are no longer needed. No explicitly defined finalizers are required, and the likelihood of resource leaks is substantially reduced.
2. Manual Resource Management
If you cannot use the try-with-resources statement (due to legacy code or other constraints), ensure you manage resources manually with a finally
block.
public void readFile(String filePath) {
FileInputStream fileInputStream = null;
try {
fileInputStream = new FileInputStream(filePath);
// Perform file read operations here
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fileInputStream != null) {
try {
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Why is this important? Using a finally
block ensures that your resources are always cleaned up, regardless of whether an exception is thrown in the try
block.
3. Implementing AutoCloseable
If you're building your own classes that manage resources, you can implement the AutoCloseable
interface, allowing your users to leverage try-with-resources.
public class Resource implements AutoCloseable {
public Resource() {
// Acquire resource
}
@Override
public void close() {
// Cleanup resource
}
}
public void useResource() {
try (Resource resource = new Resource()) {
// Use the resource here
}
}
Why use AutoCloseable? It integrates seamlessly with the try-with-resources statement, offering both simplicity and safety.
A Final Look
In summary, while finalizers in Java might appear convenient for cleaning up resources, their unpredictable nature, performance overhead, and potential complications make them a risky choice. Instead, by utilizing try-with-resources and properly managing resources with the AutoCloseable
interface, Java developers can safely and efficiently handle file I/O and other resource management tasks.
Further Reading
If you’re interested in deepening your understanding of resource management in Java, consider checking out the following:
- Java Tutorials: Try-With-Resources
- Effective Java: Item 8 - Avoid finalizers - A comprehensive guide that discusses best practices in resource handling.
By following these recommendations, your Java applications will be more reliable, efficient, and easier to maintain. Happy coding!