Finding the Parent Node in Binary Search Tree
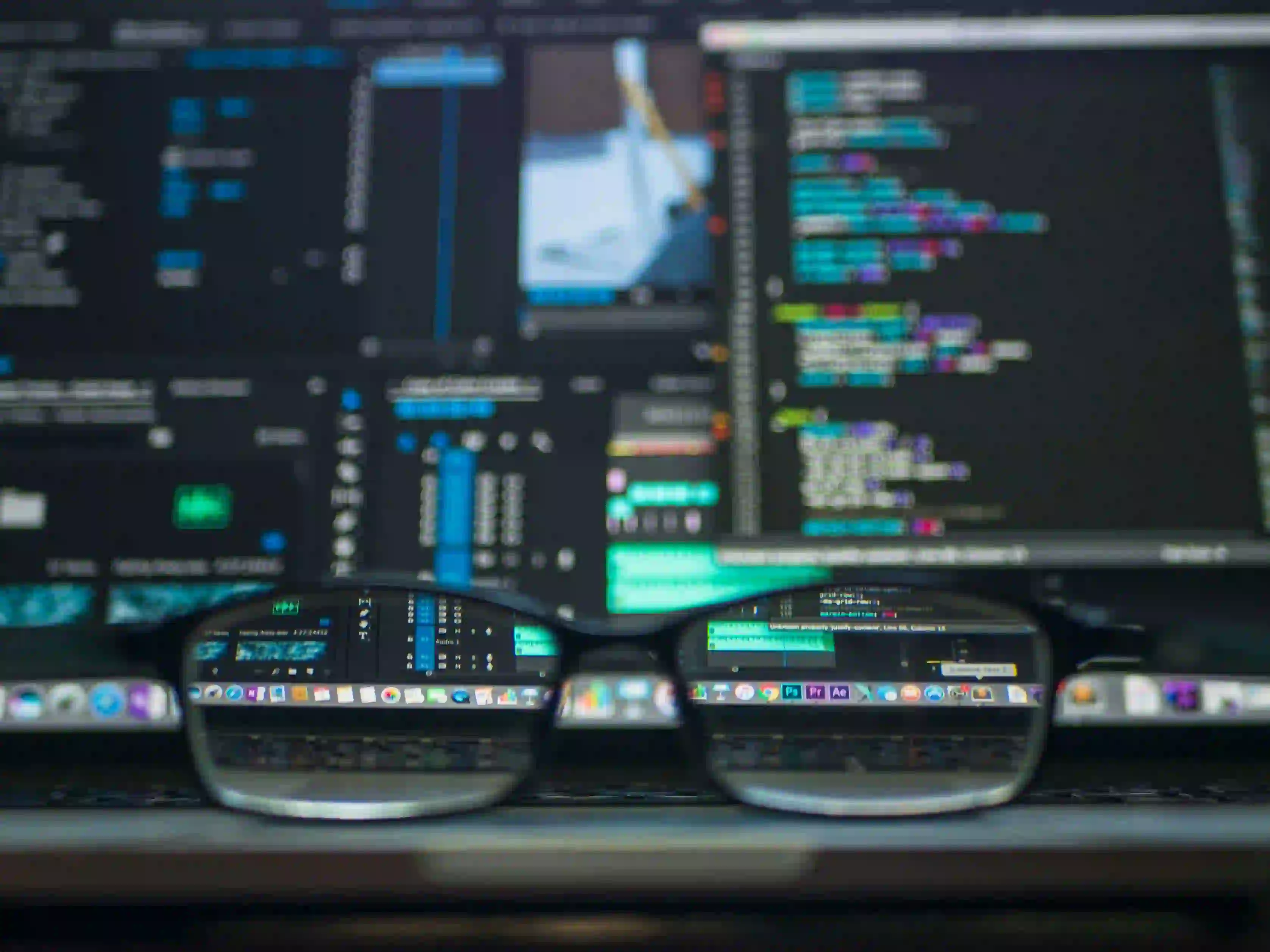
Understanding the Parent Node in Binary Search Trees (BSTs)
Binary Search Trees (BSTs) are a fundamental data structure in computer science and are used to efficiently store and retrieve data. In a BST, each node has at most two child nodes, referred to as the left child and the right child. One important operation when working with BSTs is finding the parent node of a given node. In this article, we will explore different approaches to finding the parent node in a BST and discuss their implementation in Java.
Basic Structure of a Node in a BST
Before delving into the methods for finding the parent node, let's first define the basic structure of a node in a BST. In Java, this can be represented using a class, with each node containing a value, a left child, and a right child.
class Node {
int data;
Node left;
Node right;
public Node(int data) {
this.data = data;
this.left = null;
this.right = null;
}
}
Approach 1: Traverse the Tree
One common approach to finding the parent node in a BST is to traverse the tree until the parent of the given node is found. We can start from the root of the tree and compare the value of the current node with the value of the given node. By keeping track of the parent node while traversing, we can identify the parent node when we reach the given node.
public Node findParent(Node root, Node node) {
Node parent = null;
Node current = root;
while (current != null) {
if (node.data < current.data) {
parent = current;
current = current.left;
} else if (node.data > current.data) {
parent = current;
current = current.right;
} else {
// Node found
break;
}
}
return parent;
}
In this implementation, we start from the root and traverse the tree based on the comparison of node values. We keep track of the parent node as we move down the tree, and once the given node is found, we return its parent node.
Approach 2: Recursive Approach
Another approach to finding the parent node involves using a recursive function to traverse the tree. By recursively moving down the tree and passing the current node as a parameter, we can keep track of the parent node as the recursion unwinds back up the tree.
public Node findParentRecursive(Node root, Node node, Node parent) {
if (root == null) {
return null;
}
if (root.data == node.data) {
return parent;
} else if (node.data < root.data) {
return findParentRecursive(root.left, node, root);
} else {
return findParentRecursive(root.right, node, root);
}
}
This recursive approach provides a concise way to find the parent node while traversing the tree. We pass the parent node as a parameter in each recursive call, allowing us to track the parent node of the given node.
Key Takeaways
In summary, we have explored two fundamental approaches to finding the parent node in a Binary Search Tree. The first approach involves iterative traversal of the tree, while the second approach uses recursion for a more concise solution. Both approaches have their advantages, and the choice between them may depend on the specific requirements and constraints of the application.
Understanding how to find the parent node in a BST is crucial when working with tree data structures and can be applied in various algorithms and operations. Implementing these approaches in Java provides a solid foundation for navigating and manipulating BSTs efficiently.
For more in-depth insights into BSTs and Java programming, you can refer to GeeksforGeeks and Oracle's official Java documentation.