Preventing Java Errors: Strategies Before Production Launch
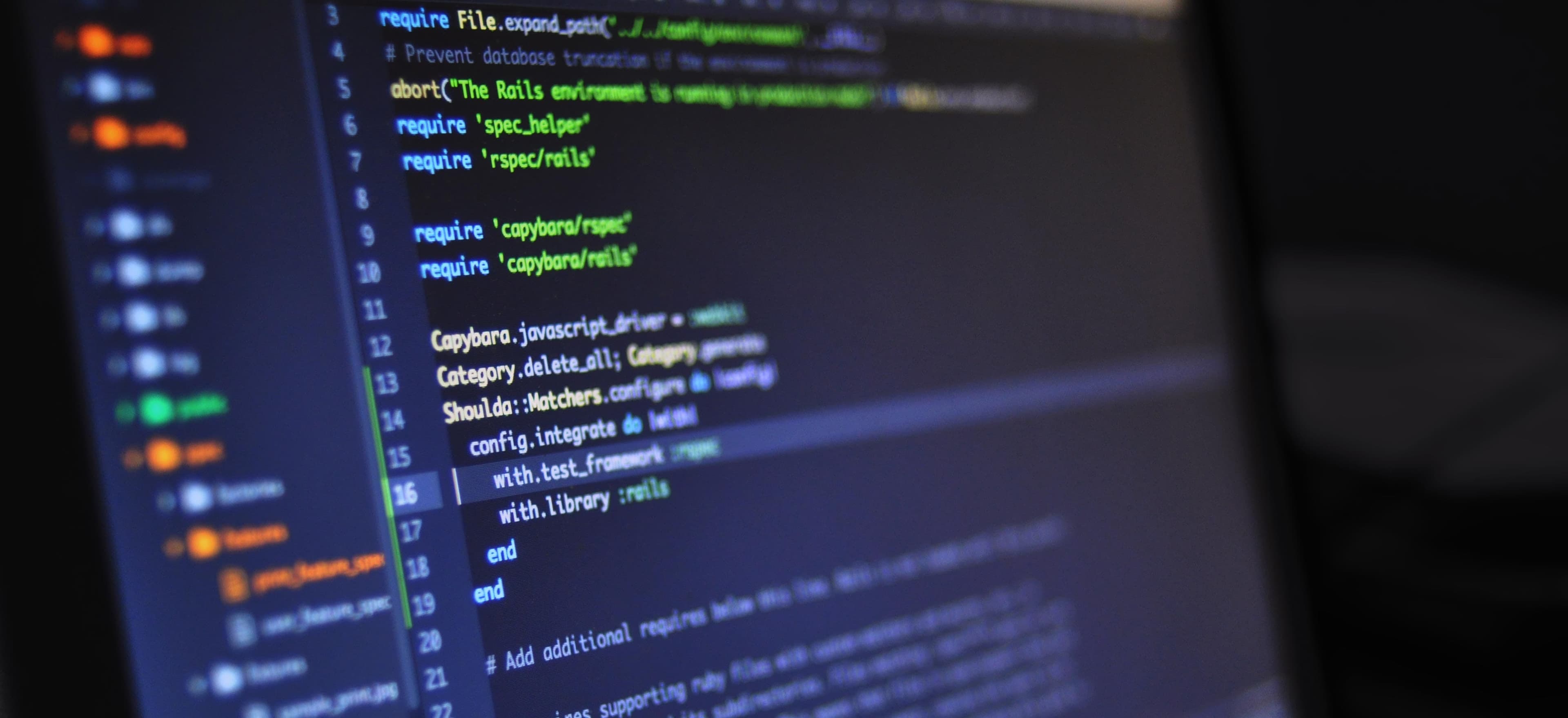
- Published on
Preventing Java Errors: Strategies Before Production Launch
Java is one of the most widely-used programming languages in the world, renowned for its portability, scalability, and robustness. However, like any other programming language, Java is not immune to errors. When these errors slip through before launching a production application, they can lead to disastrous consequences. In this blog post, we will explore effective strategies to help you prevent Java errors before your application goes live.
Understanding the Types of Errors
Before diving into preventive measures, it’s essential to understand the types of errors that can occur in Java applications. Generally, these can be categorized as:
- Syntax Errors: Mistakes in the code that violate the grammar of the Java language.
- Runtime Errors: Errors that occur while the application is running, often leading to crashes.
- Logical Errors: Flaws that lead to incorrect output without syntax or runtime notifications.
Recognizing these errors is the first step toward preventing them. So, how do you ensure that your Java application is ready for production?
Strategies for Minimizing Errors
1. Code Review and Pair Programming
One effective way to prevent errors is through rigorous code reviews. This approach involves having another developer scrutinize the code for mistakes or improvements. Pair programming, in which two developers work side-by-side, is also beneficial.
Example Code Review Recommendations:
public void calculateSalary(double baseSalary, double commissionRate) {
double commission = baseSalary * commissionRate; // Consider type-checking commissionRate
return baseSalary + commission;
}
Why Consider This?
Code reviews can catch mistakes such as incorrect data types or unhandled exceptions, preventing potential runtime errors. Official guidelines can also be established to ensure that no critical aspect gets overlooked.
2. Utilize Static Analysis Tools
Static analysis tools can analyze your Java code without execution. They look for potential issues, such as code smells, style violations, and more, before you run your applications.
Recommended Static Analysis Tools:
- SonarQube: Offers in-depth insights into code quality.
- Checkstyle: Ensures adherence to coding standards.
- SpotBugs: Identifies possible bugs in Java programs.
Integrating such tools into your Continuous Integration (CI) pipeline can help catch errors early.
3. Comprehensive Unit Testing
Testing is critical to ensuring that your software behaves as expected. Unit tests allow you to validate individual pieces of code thoroughly. Java developers often rely on testing frameworks like JUnit or TestNG for this purpose.
Example Unit Test:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class SalaryCalculatorTest {
@Test
public void testCalculateSalary() {
SalaryCalculator calculator = new SalaryCalculator();
double result = calculator.calculateSalary(1000, 0.1);
assertEquals(1100, result, 0.001); // Checking accuracy within a delta
}
}
Why Unit Testing?
Unit tests allow you to confirm the correctness of each part of your code independently. They serve as documentation and help identify changes that introduce new errors.
4. Use Exception Handling Wisely
Java provides a robust exception handling mechanism that can effectively deal with errors. Using try-catch blocks, you can gracefully manage exceptions and avoid sudden application crashes.
Example Exception Handling:
public void readFile(String filename) {
try {
FileReader reader = new FileReader(filename);
// Reading operations
} catch (FileNotFoundException e) {
System.err.println("File not found: " + filename);
// Log error or take alternative actions
}
}
Why Exception Handling?
Implementing effective exception handling prevents your application from crashing unexpectedly. Proper logging allows for easier identification and troubleshooting of the issues.
5. End-to-End Integration Testing
Before launching your application, it is essential to test the complete system to capture integration errors that may not surface during unit testing.
Example Integration Test Framework:
Consider using tools like JUnit, Spring Test, or Testcontainers which allow you to simulate entire application scenarios.
@SpringBootTest
public class ApplicationIntegrationTest {
@Test
public void testServiceIntegration() {
AppService service = new AppService();
String result = service.performDbOperation();
assertNotNull(result); // Validate the integration layer
}
}
Why Integration Testing?
Integration tests help you evaluate how various components of your application work together, catching miscommunication between systems.
6. Performance Testing
Performance testing can reveal potential bottlenecks or heavy-load issues in your application that can lead to crashes.
Performance Testing Tools:
- JMeter: For load testing.
- Gatling: Focused on high-performance applications.
7. Maintain Version Control
Using a Version Control System (VCS) like Git enables you to revert to previous stable versions of your application if new changes cause issues.
Why Use Version Control?
Version control keeps your project organized, allowing quick recovery from errors introduced by recent changes or features.
8. Create Robust Documentation
Documentation not only serves as a guideline for current and future developers but also becomes a reference point for error resolution.
Why Documentation?
Having a clear structure and guidelines fosters a better understanding of the application and enhances the team's ability to troubleshoot any future problems effectively.
Ensure Continuous Monitoring
After the production launch, continuous monitoring lays the groundwork for ongoing error detection, enabling you to quickly discover potential issues. Tools like New Relic or Prometheus can be set up to monitor the performance and reliability of your application in real-time.
Closing Remarks
Preventing Java errors before a production launch is not just about writing code; it's about instituting a culture of quality, testing, and continuous improvement. By employing techniques such as code reviews, static analysis, unit testing, and documentation, you can mitigate risks significantly. Implement these strategies, and your Java application is likely to soar to greater heights without the hiccups of unwanted errors.
If you're interested in deepening your understanding further, feel free to check out additional resources on Java Testing Techniques or Best Practices in Java.
Happy coding!