Top 5 Mistakes to Avoid in Java Development
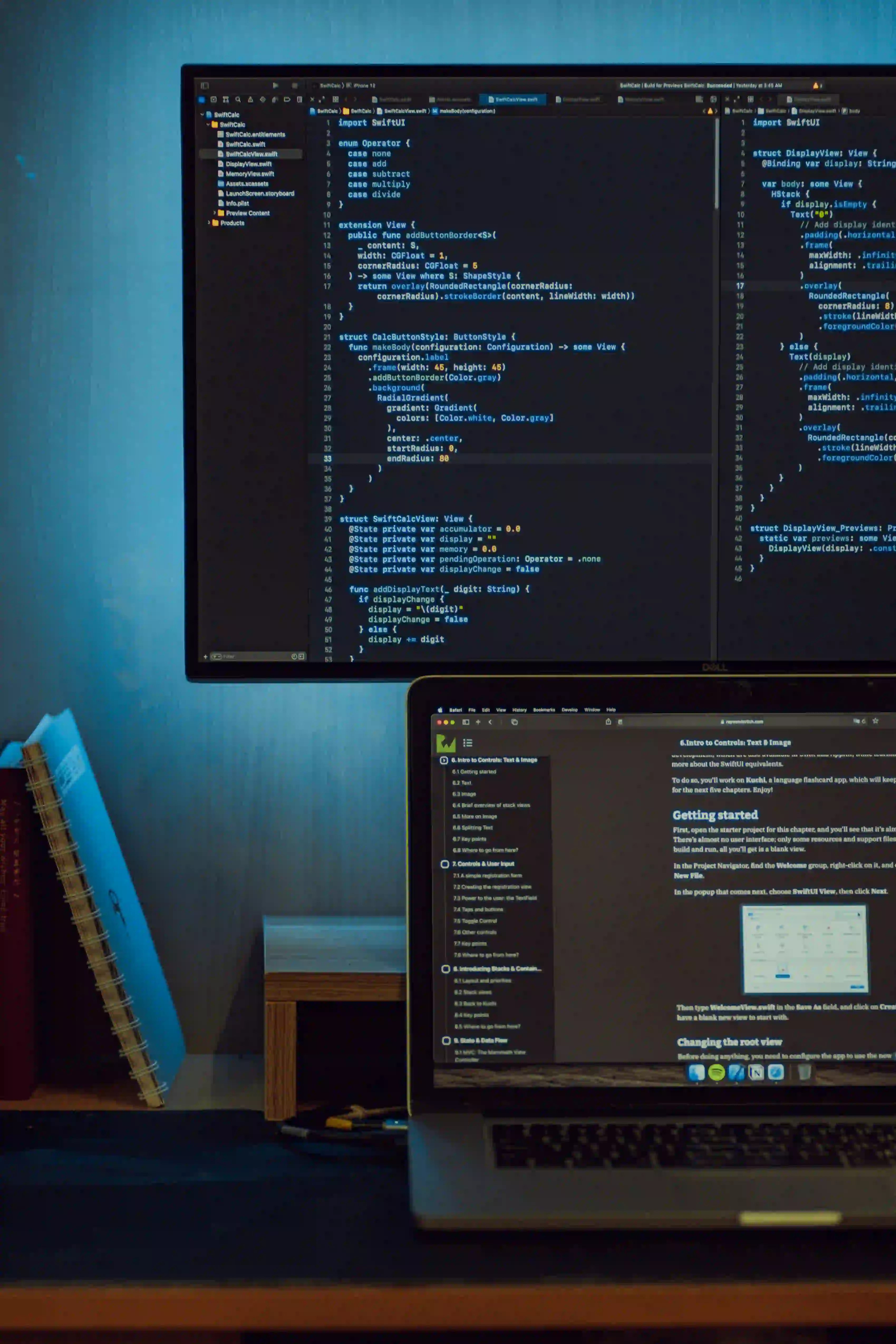
In the world of Java development, even seasoned developers can fall into common pitfalls that can impact the performance and maintainability of their code. Whether you're a newcomer or a veteran, steering clear of these common mistakes is crucial for writing clean, efficient, and maintainable Java code.
1. Neglecting Exception Handling
Exception handling is a fundamental aspect of Java programming. Failing to appropriately handle exceptions can lead to unpredictable behavior and potential application crashes. It's essential to handle exceptions at the right level of abstraction and to provide meaningful error messages to aid in debugging.
try {
// Code that may throw an exception
} catch (SpecificException ex) {
// Handle the specific exception
} catch (AnotherException ex) {
// Handle a different specific exception
} catch (Exception ex) {
// Handle any other exceptions
} finally {
// Optional cleanup code
}
When handling exceptions, it's vital to log the exception details for troubleshooting, gracefully handle the error, and avoid hiding exceptions by catching them silently.
For a deeper understanding of exception handling in Java, you can refer to this article The Complete Java Exceptions Guide.
2. Overlooking Memory Management
Java provides automatic memory management through garbage collection. However, this doesn't mean that memory management can be disregarded. Inefficient memory use can lead to performance issues such as increased garbage collection overhead and potential memory leaks.
It's important to be mindful of memory allocation, especially when working with large data sets or in performance-critical applications. Using tools like JVisualVM or Java Mission Control to analyze and optimize memory usage can be immensely beneficial.
// Inefficient memory allocation
List<Object> list = new ArrayList<>();
// Better approach using initial capacity
List<Object> list = new ArrayList<>(1000);
By paying attention to memory management, you can ensure that your Java applications perform optimally and are free from memory-related issues.
3. Ignoring Java Naming Conventions
Java has well-established naming conventions for classes, methods, variables, and packages. Neglecting these conventions can make the codebase harder to understand and maintain, especially when collaborating with other developers.
Adhering to naming conventions, such as using camelCase for method names and PascalCase for class names, not only improves the readability of the code but also helps in maintaining a consistent style across the project.
// Incorrect method name
int CalculateTotal() {
// Method implementation
}
// Corrected method name following conventions
int calculateTotal() {
// Method implementation
}
By following naming conventions, you contribute to writing clear and consistent code that is easily comprehensible by other developers, ultimately enhancing the maintainability of the codebase.
4. Failing to Optimize Loops
Loops are a fundamental part of Java programming, and inefficient loop structures can significantly impact the performance of an application, especially when dealing with large datasets or executing intensive computational tasks within loops.
// Inefficient loop over a collection
for (int i = 0; i < list.size(); i++) {
// Accessing list elements
}
// Optimized loop using enhanced for loop
for (Object obj : list) {
// Accessing list elements
}
Utilizing enhanced for loops, parallel streams, or iterator patterns when iterating over collections can lead to clearer and more efficient code. Additionally, minimizing work within loops and avoiding unnecessary iterations are essential for optimal loop performance.
5. Neglecting Code Reusability and Modularity
Writing redundant code and neglecting code reusability can lead to bloated codebases and increased maintenance efforts. Embracing modularity and ensuring code reusability through methods, classes, and interfaces promotes a more organized and maintainable codebase.
// Redundant code without reusability
// Method 1
...
// Method 2
...
// Better approach with code reusability
// Common method
void commonMethod() {
// Reusable implementation
}
By encapsulating functionality into reusable components and promoting modularity, you can enhance the flexibility and maintainability of your Java codebase.
In conclusion, by being mindful of these common Java development mistakes, you can ensure that your code is not only efficient and maintainable but also follows best practices in the industry. In the dynamic world of software development, learning from these mistakes and continuously improving your coding practices is paramount to becoming a proficient Java developer.
What are some other common mistakes in Java development that you think developers should avoid? Share your thoughts and experiences in the comments below.