How to Tackle Performance Issues from JIT Compilation Jitter
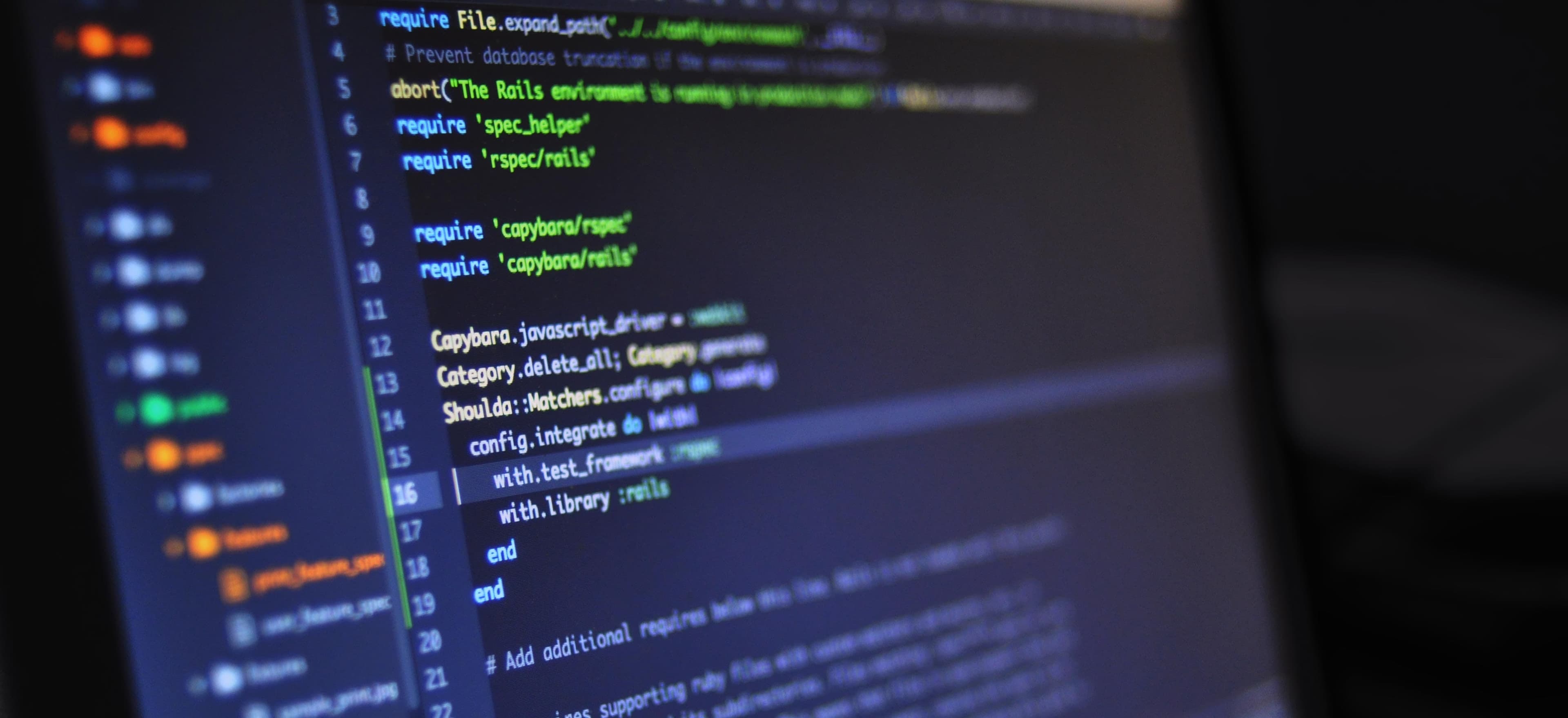
- Published on
How to Tackle Performance Issues from JIT Compilation Jitter
Java has long been celebrated for its portability, maintainability, and performance optimizations, primarily through its Just-In-Time (JIT) compilation. However, developers may sometimes encounter performance hiccups due to JIT compilation jitter. This blog post delves into the causes of JIT jitter and offers practical strategies to mitigate performance issues associated with it.
Understanding JIT Compilation Jitter
JIT compilation translates Java bytecode into native machine code during runtime to improve performance. The JVM (Java Virtual Machine) dynamically decides which methods to compile based on their execution frequency.
Despite its advantages, such as optimizing hot paths and reducing repetitive interpretation overhead, JIT compilation jitter can introduce latency in applications. This jitter occurs when the JIT compiler optimizes code, leading to variations in response times.
Causes of JIT Compilation Jitter
-
Warm-Up Time: JIT compilation may take some time to analyze and optimize the code effectively. During this period, the performance can be inconsistent.
-
Compilation Decisions: The JVM makes decisions on when and what to compile based on runtime profiling. These decisions may not always lead to optimal performance.
-
Garbage Collection (GC): Frequent GC events can interrupt the JIT compilation process and lead to performance stalls.
-
Dynamic Code Features: Applications relying heavily on dynamic features like reflection or proxies can cause unpredictable JIT behavior.
Identifying JIT Performance Issues
Before addressing performance problems, it's critical to identify that they stem from JIT compilation jitter. Below are some methods to diagnose JIT-related performance issues:
- Profiling Tools: Using profilers such as VisualVM or YourKit can help monitor application performance and track where time is spent.
- JVM Flags: Enable JIT-related JVM flags (e.g.,
-XX:+PrintCompilation
) to obtain insights into what methods are being compiled and when. - Benchmarking: Perform controlled benchmarks, including warm-up iterations, to measure performance nuances accurately.
Strategies to Mitigate JIT Compilation Jitter
1. Optimize Application Warm-Up Time
One of the simplest ways to counteract the effects of jitter is to ensure that the application has a sufficient warm-up period. This can be accomplished with the following strategy:
public class WarmUpExample {
public static void main(String[] args) {
for(int i = 0; i < 1_000_000; i++) {
performSomeOperation();
}
// At this point, JIT optimizations are likely in effect
long startTime = System.nanoTime();
// Call methods to benchmark here
System.out.println("Execution time: " + (System.nanoTime() - startTime) + " ns");
}
private static void performSomeOperation() {
// Simulate work
Math.sin(Math.random());
}
}
Why: By executing warm-up iterations before measuring performance, you allow the JVM to optimize your code paths, reducing jitter when you perform actual benchmarks.
2. Adjusting Compiler Options
The JVM includes several options that can be fine-tuned to mitigate JIT performance issues. For example, using the -XX:CompileThreshold
option, you can adjust the number of method invocations before the method is JIT-compiled.
java -XX:CompileThreshold=200 -jar yourApp.jar
Why: Reducing the threshold allows methods that are called less frequently to get compiled, which can help reduce performance bottlenecks in cases where certain methods are heavily-used but not commonly hit.
3. Profile-Guided Optimizations
Use profiling data collected during the application's runtime to inform JIT decisions. The JVM can use historical execution paths to make better decisions about optimizations.
- Tools: Use tools and options such as
-XX:+UseProfiledCompilation
, which lets the JVM take advantage of profiling data.
Why: By leveraging actual performance data, it can lead to more informed and efficient JIT compilation strategies, improving overall performance.
4. Minimize Dynamic Features
If your application often uses reflection or dynamically generated classes that require JIT compilation, consider minimizing their use.
public class ReflectionExample {
public static void main(String[] args) throws Exception {
Class<?> clazz = Class.forName("java.lang.String");
Method method = clazz.getMethod("length");
Object instance = "Hello";
// Dynamically invocate method
System.out.println(method.invoke(instance)); // This often causes jitter
}
}
Why: Reflection can prevent the JIT compiler from fully optimizing for dynamic method invocations. Where possible, static calls should be preferred to provide the JIT compiler the chance to apply optimizations effectively.
5. Memory Management Considerations
Garbage collection (GC) can interfere with JIT compilation. Consider tuning your JVM's garbage collection settings or even deploying a different GC strategy based on your application's needs.
java -XX:+UseG1GC -jar yourApp.jar
Why: The G1 garbage collector aims to provide predictable latency, which can reduce the impact of GC on JIT compilation, thus minimizing interruptions.
6. Use of Ahead-of-Time (AOT) Compilation
Consider using AOT compilation where suitable. This method compiles bytecode to native code ahead of execution. While this does negate some dynamic optimization capabilities inherent in JIT, it can provide more consistent startup performance.
native-image -jar yourApp.jar
Why: Pre-compiling using AOT can mitigate JIT-related jitter by reducing the number and frequency of overhead JIT compilations at runtime.
In Conclusion, Here is What Matters
Navigating the complexities of JIT compilation jitter requires utilizing a multi-faceted approach. By considering warm-up times, refining compiler options, tuning memory management, and minimizing dynamic language features, Java developers can significantly enhance application performance. Be proactive and analyze your application with profiling tools, and never hesitate to experiment with various JVM configurations to find an optimal setup for your needs.
For additional information about Java optimization strategies, please refer to the official Java Performance Tuning Guide and the Java Documentation.
Happy coding!
Checkout our other articles