Common Pitfalls in Java EE 7 with WildFly and Docker
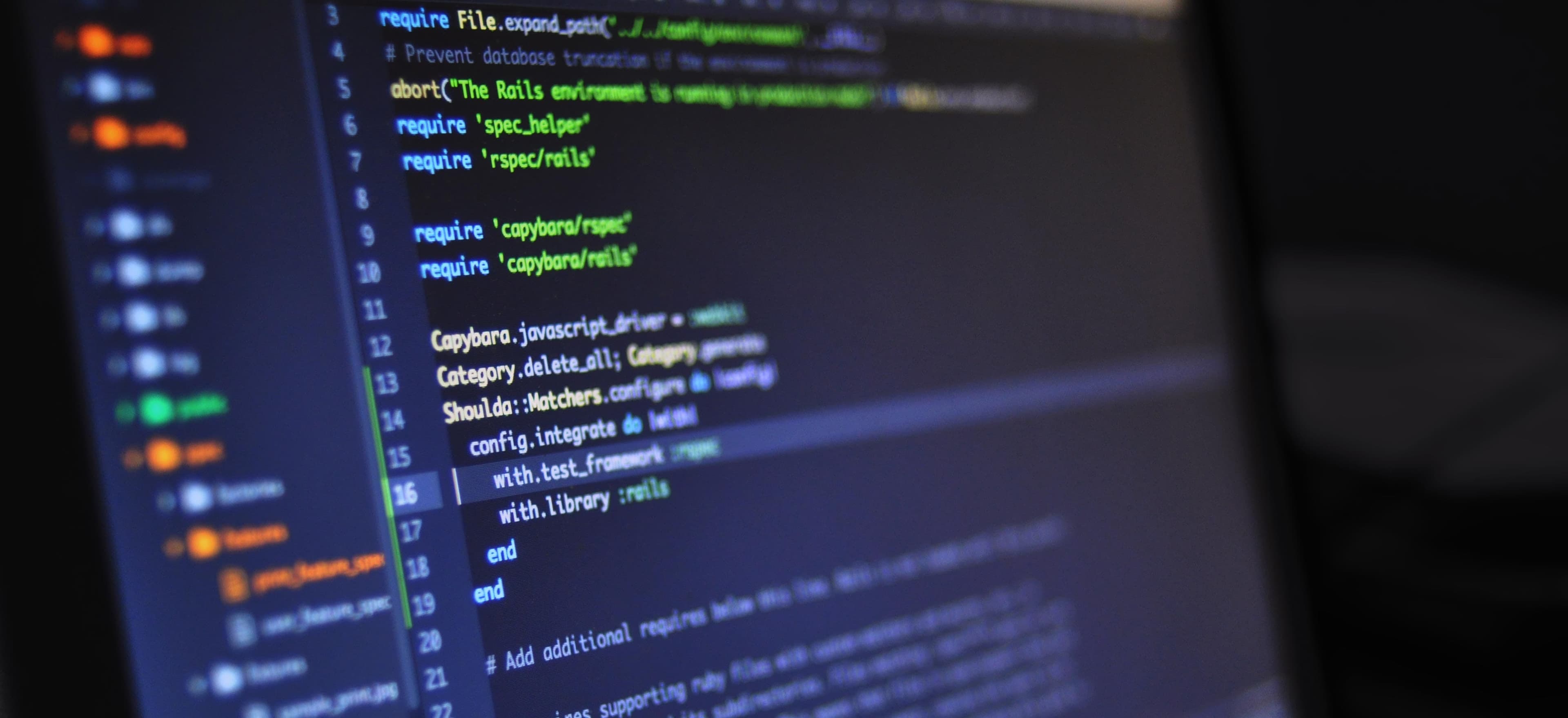
- Published on
Common Pitfalls in Java EE 7 with WildFly and Docker
Java EE (Enterprise Edition) 7 is a robust platform for developing large-scale server-side applications. When combined with WildFly, which is an application server that implements Java EE specifications, and Docker, a tool for creating and managing containers, the power of Java EE can be unleashed effectively. However, as developers venture into this territory, several common pitfalls can emerge. This blog post explores these pitfalls while providing tips for best practices to enhance your Java EE projects.
Understanding Java EE 7 and WildFly
Java EE 7 introduced a plethora of specifications that boost enterprise application development. These include:
- JAX-RS (RESTful Web Services): Simplifies the creation of RESTful applications.
- EJB (Enterprise JavaBeans): Manages business logic and provides a robust transaction mechanism.
- JPA (Java Persistence API): Facilitates data manipulation and database interaction.
WildFly implements these Java EE specs, providing a lightweight and powerful server to deploy applications. This server is often paired with Docker, which allows developers to package applications into containers, ensuring smooth deployment across various environments.
Pitfall #1: Misconfiguring WildFly
The Importance of Proper Configuration
Misconfiguration of WildFly can lead to performance issues, security vulnerabilities, or even application downtime. This may arise from:
- Incorrect JVM parameters.
- Misconfigured data sources.
- Improper resource allocation for EJB pools.
Solution
Start by defining the right Java Virtual Machine (JVM) options and configuring the standalone.xml
correctly. Here’s an example of how to configure the maximum heap size:
<system-properties>
<property name="java.rmi.server.hostname" value="YOUR_IP"/>
<property name="java.util.logging.manager" value="org.jboss.logmanager.LogManager"/>
</system-properties>
Why? Setting the appropriate network settings in standalone.xml
ensures that RMI calls can be accessed correctly, enhancing the communication between distributed components.
Additionally, don’t forget to set data sources appropriately within the management console or configuration file:
<datasource jndi-name="java:/jdbc/MyDS" pool-name="MyDS" enabled="true">
<connection-url>jdbc:mysql://localhost:3306/mydb</connection-url>
<driver>mysql</driver>
<security>
<user-name>user</user-name>
<password>password</password>
</security>
</datasource>
Why? Properly configuring data sources ensures that the application can connect to the database without delays and security issues.
Resources for Further Reading
- WildFly Documentation
- Java EE 7 Specifications
Pitfall #2: Ignoring Dependency Management
The Dependency Management Challenge
Problems usually arise when developers overlook dependency management, especially in large applications. Dependencies can conflict with each other, leading to RuntimeExceptions or ClassNotFoundExceptions.
Solution
Always use a build management tool like Maven or Gradle to manage dependencies. For Maven, ensure the pom.xml
file is appropriately configured:
<dependency>
<groupId>org.jboss.wildfly.plugins</groupId>
<artifactId>wildfly-maven-plugin</artifactId>
<version>${wildfly.version}</version>
</dependency>
Why? This setup provides version control and ensures that all necessary dependencies are downloaded automatically, reducing the risk of errors during the build process.
Pitfall #3: Not Leveraging Docker Correctly
Docker Misunderstandings
While Docker brings numerous benefits, misunderstandings around its usage can lead to significant issues. Common mistakes include:
- Not using a
.dockerignore
file to exclude unnecessary files. - Running multiple services in a single container, violating the Docker philosophy of one service per container.
Solution
Make use of a .dockerignore
file to reduce the size of your Docker images and ensure that unnecessary files are not copied.
Here's a simple example:
# .dockerignore
.target
.git
.DS_Store
*.log
Why? This practice helps maintain cleaner images and reduces build times, ensuring faster deployment and easier navigation.
When writing your Dockerfile, be sure to divide services into separate containers. Here's how a simple Dockerfile looks for a WildFly application:
FROM jboss/wildfly:latest
COPY target/myapp.war /opt/jboss/wildfly/standalone/deployments/
Why? Keeping services separate allows for easier scaling and management of resources.
Pitfall #4: Neglecting Testing
The Testing Oversight
Many developers prioritize deployment over testing, and while it may seem faster in the short term, it leads to greater issues later. Uncovered bugs can be a nightmare to debug and fix.
Solution
Emphasize a solid testing strategy using frameworks like JUnit or Arquillian. Here is a simple JUnit test example that checks a REST endpoint:
@WebService
@Stateless
public class MyService {
public String getResponse() {
return "Hello, World";
}
}
And the corresponding test case would be:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MyServiceTest {
MyService service = new MyService();
@Test
public void testGetResponse() {
assertEquals("Hello, World", service.getResponse());
}
}
Why? Testing not only verifies the functionality of components but also ensures that they work together as expected.
Pitfall #5: Overlooking Monitoring and Logging
The Importance of Observability
Skipping logging and monitoring can lead to a lack of insights into application performance or issues. If bottlenecks occur or errors arise, knowing where to look can save significant time.
Solution
Implement a logging strategy using tools like Log4j or the Java Logging API. Here’s how to configure a basic logger:
import java.util.logging.Logger;
public class MyApplication {
private static final Logger logger = Logger.getLogger(MyApplication.class.getName());
public void doSomething() {
logger.info("Something is being done.");
}
}
Why? Proper logging allows developers to trace the application's behavior over time, making it easier to debug issues when they arise.
Consider integrating monitoring tools like Prometheus or Grafana to visualize and analyze performance metrics over time.
Wrapping Up
While Java EE 7, WildFly, and Docker present fantastic opportunities for enterprise application development, understanding and avoiding common pitfalls is crucial for success. Proper configuration, dependency management, leveraging Docker correctly, thorough testing, and effective logging and monitoring can make the difference between a thriving application and a maintenance nightmare.
By adopting these best practices, you can enhance your development workflow and pave the way for robust Java EE applications that stand the test of time. Happy coding!