Boost Your Java Project Efficiency: SBT Integration Tips
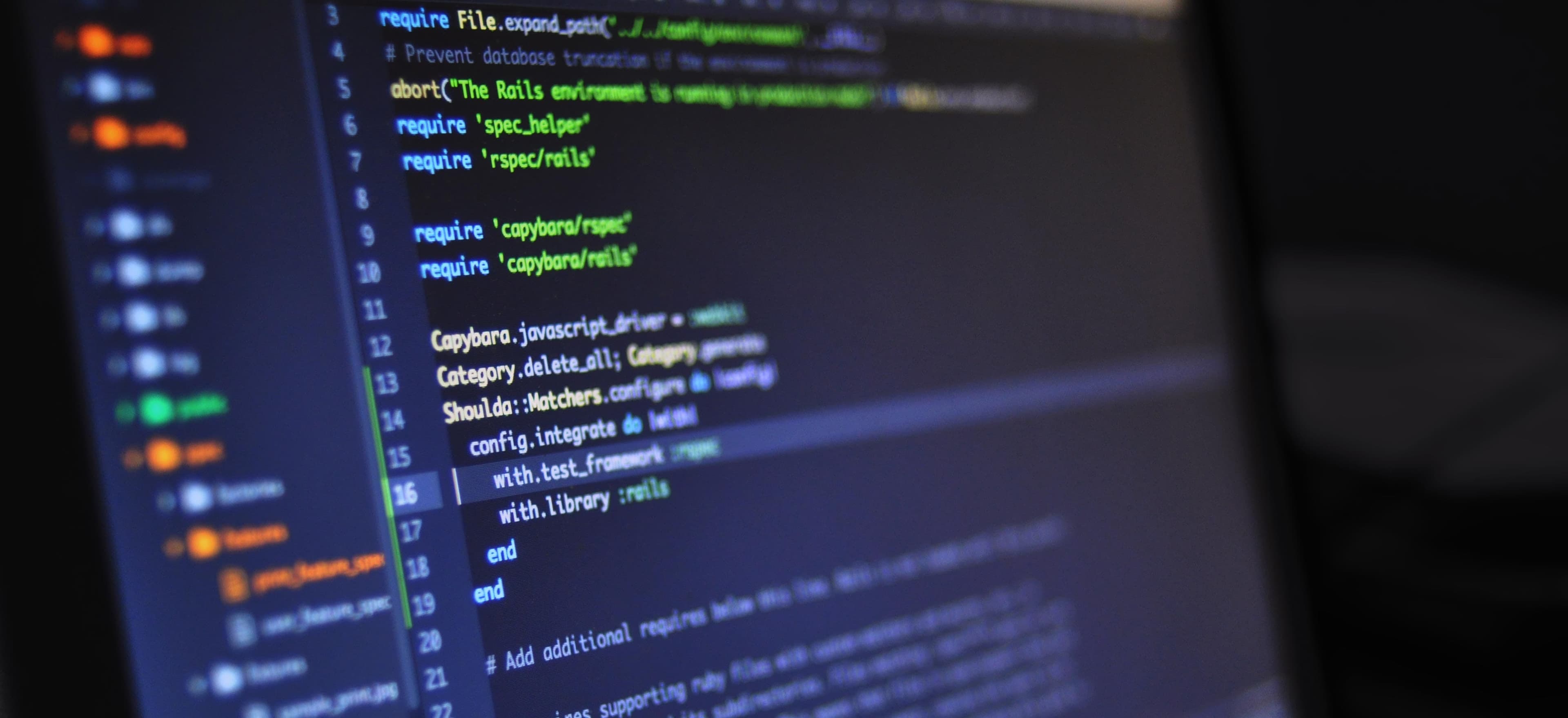
- Published on
Boost Your Java Project Efficiency: SBT Integration Tips
In the ever-evolving world of software development, efficiency is key. For Java developers, leveraging tools that streamline builds, dependencies management, and project configurations is paramount. One such tool is SBT (Simple Build Tool), which is renowned in the Scala community but can significantly enhance Java projects too. In this post, we will delve into the intricacies of SBT, its integration with Java projects, and tips to boost your development efficiency.
What is SBT?
SBT is a build tool primarily geared towards Scala projects but boasts strong support for Java. With its incremental compilation, dependency resolution, and interactive shell, it simplifies the building process and enhances productivity. Notably, SBT provides a concise method for specifying project configurations using a flexible build definition syntax based on Scala.
Why Choose SBT for Java Projects?
- Incremental Compilation: SBT only recompiles the code that changed, leading to faster build times.
- Dependency Management: With its powerful resolver, SBT can manage complex dependency trees effortlessly.
- Support for Multi-project Builds: Handling multiple modules within a single build is seamless.
- Integration with IDEs: SBT works well with popular IDEs like IntelliJ IDEA and Eclipse, streamlining the development workflow.
Getting Started with SBT
Before delving deeper, let’s get some basics down. Here’s how you can integrate SBT into a Java project.
Step 1: Install SBT
To install SBT, follow the official installation guide. You can install it via Homebrew on macOS, apt on Ubuntu, or download a standalone version directly.
Step 2: Create a New SBT Project
You can create a new project easily. Open a terminal and run the following commands:
mkdir my-java-project
cd my-java-project
sbt new sbt/scala-seed.g8
This command sets up a new project structure. Next, transition to a Java-centric directory structure.
Step 3: Modify Project Structure for Java
mkdir -p src/main/java
mkdir -p src/test/java
In this case, we've created folders for both the main Java source files and test files.
Your project will look like this:
my-java-project
├── build.sbt
└── src
└── main
└── java
Step 4: Configure build.sbt
Edit the build.sbt
file to include your project settings:
name := "MyJavaProject"
version := "0.1"
scalaVersion := "2.13.12" // Optional, for Scala interop
libraryDependencies += "org.slf4j" % "slf4j-api" % "1.7.30" // Example dependency
The libraryDependencies
line adds an example library that might be useful for your project.
Step 5: Add Java Code
Create a simple Java class inside src/main/java
. For example:
package com.example;
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, SBT and Java!");
}
}
Step 6: Running Your Application
To compile and run the application, execute the following command in your project root:
sbt run
You should see:
[info] Running com.example.HelloWorld
Hello, SBT and Java!
Essential SBT Tips for Java Developers
1. Leverage Multi-Project Builds
If you have multiple modules, SBT's multi-project capability allows you to organize your modules efficiently. In your build.sbt
:
lazy val root = (project in file("."))
.aggregate(moduleA, moduleB)
lazy val moduleA = (project in file("moduleA"))
lazy val moduleB = (project in file("moduleB"))
This approach creates a root project consisting of multiple modules, allowing for shared dependencies and streamlined builds.
2. Utilize Custom Tasks
SBT allows you to define custom tasks using Scala. This feature can automate repetitive tasks.
lazy val printHello = taskKey[Unit]("Prints Hello, World")
printHello := {
println("Hello, SBT!")
}
Run this task using:
sbt printHello
3. Incremental Compilation
If you're constantly editing your code, enable incremental compilation by leveraging the SBT shell. When you start SBT, it automatically watches for changes and recompiles only the edited files.
4. Test Framework Integration
SBT integrates seamlessly with testing frameworks like JUnit and ScalaTest. You can specify dependencies in your build.sbt
, enabling you to run and manage tests effectively:
libraryDependencies += "junit" % "junit" % "4.12" % Test
Now, place your test classes in the src/test/java
directory and run them using:
sbt test
5. Managing Dependencies
To manage dependencies effectively, use the libraryDependencies
field in your build.sbt
. Here’s an example:
libraryDependencies ++= Seq(
"org.apache.commons" % "commons-lang3" % "3.12.0",
"com.google.guava" % "guava" % "30.1-jre"
)
This approach keeps your dependencies organized and enhances collaboration within teams.
6. Using SBT Plugins
There are numerous plugins available for SBT that can enhance functionality. For instance, to monitor and visualize project dependencies, add the following plugin to project/plugins.sbt
:
addSbtPlugin("org.johnrengelman" % "shadow" % "7.1.2")
This plugin can be helpful for creating a fat jar, which bundles all the required libraries together.
A Final Look
Integrating SBT into your Java projects can lead to substantial efficiency improvements. From incremental builds to seamless dependency management, SBT offers a sophisticated and flexible solution for modern Java development. As your projects scale, these capabilities become invaluable.
By leveraging the tips outlined in this post, you can maximize your productivity and streamline your workflows. For further learning about SBT, consider following the official documentation at SBT Documentation.
Happy coding!