Why Agile Fails in Software Maintenance Projects
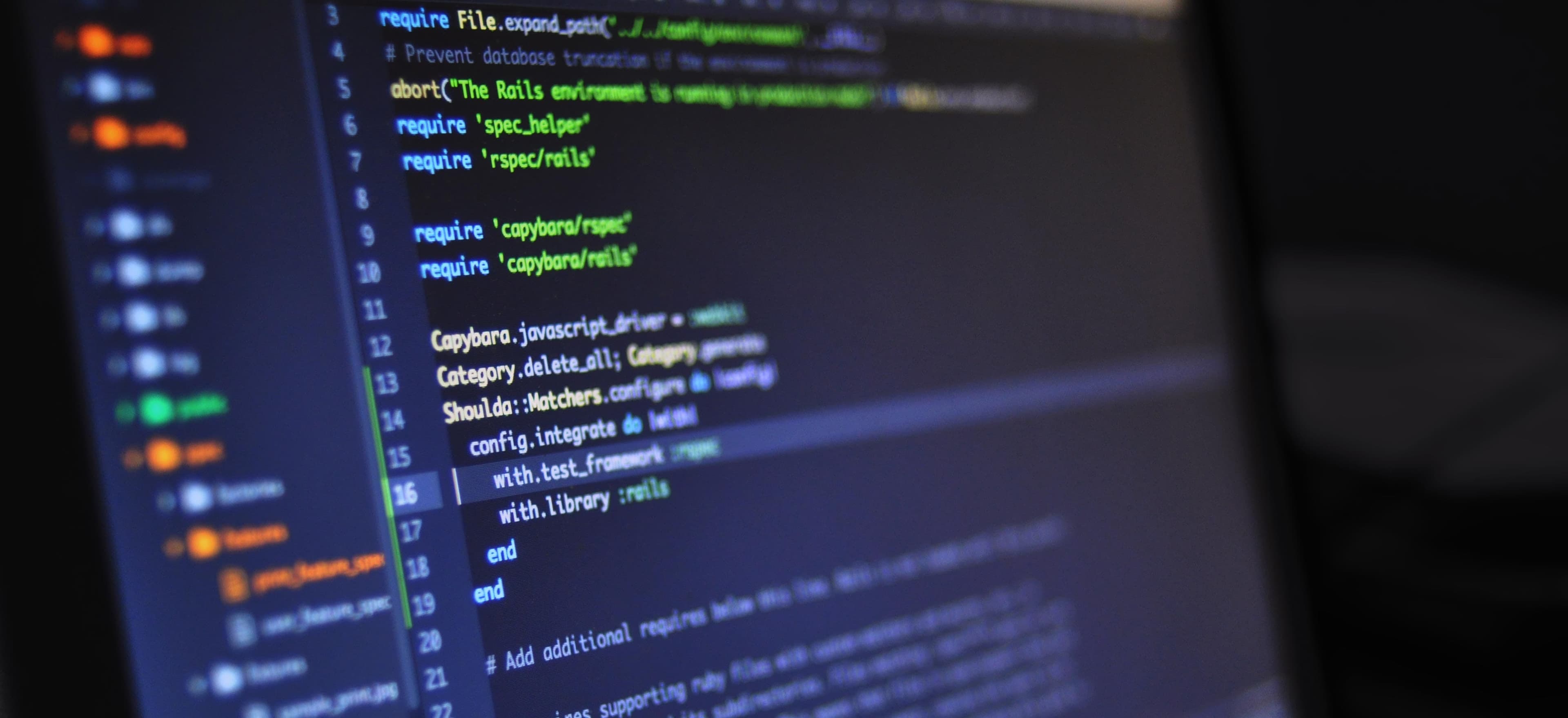
- Published on
Why Agile Fails in Software Maintenance Projects
Agile methodologies have been transformative in the way we approach software development. From Sprint planning to Continuous Integration, Agile frameworks like Scrum and Kanban strive to enhance efficiency and adaptability. However, as we delve into software maintenance projects, the effectiveness of Agile can become questionable. In this blog post, we will discuss why Agile may fail in these contexts and provide practical insights backed by code snippets and industry best practices.
Understanding Software Maintenance Projects
Before we dissect the reasons behind Agile's shortcomings in software maintenance, it's essential to understand what software maintenance entails. Software maintenance can be categorized into four types:
- Adaptive Maintenance: Modifying software to accommodate changes in the environment.
- Corrective Maintenance: Fixing bugs or issues that arise after the software is operational.
- Perfective Maintenance: Enhancing and improving features based on user needs.
- Preventive Maintenance: Modifications aimed at avoiding future problems.
While Agile thrives in dynamic development settings, the static nature of maintenance tasks can undermine its core principles.
Top Reasons Agile Fails in Software Maintenance
1. Lack of Clear Requirements
Agile methodologies emphasize user stories and active collaboration. However, in maintenance projects, requirements can be unclear or incomplete. As a result, teams may work on assumptions rather than clear objectives, leading to wasted effort and rework.
Example:
public class MaintenanceTask {
private String description;
private boolean isResolved;
public MaintenanceTask(String description) {
this.description = description;
this.isResolved = false;
}
public void resolve() {
// Assume we know how to resolve based on above assumptions
isResolved = true;
}
}
// In a maintenance project, a clear description is crucial for effective task resolution.
MaintenanceTask task = new MaintenanceTask("Fix null pointer exception in user profile.");
Why This Matters: This code snippet shows how unclear requirements can lead to unresolved issues in maintenance. If the requirements for fixing the bug are not clear, the developer will spend time guessing the resolution.
2. Resistance to Change
Agile encourages adaptability; however, maintenance teams are often resistant to change. Dealing with legacy code can make developers hesitant to alter existing systems, fearing a ripple effect of issues.
Example:
// Consider a legacy system
public class LegacyDatabase {
public void fetchData() {
// Outdated logic
System.out.println("Fetching data from Legacy DB.");
}
}
// Introducing new logic in a maintenance project can be risky.
public class NewDatabase {
public void fetchData() {
// New and improved logic
System.out.println("Fetching data from New DB.");
}
}
Why This Matters: Resistance to change hinders modernization efforts. The snippet contrasts the legacy system with a new approach. Abrupt changes can lead to system instability, complicating maintenance work.
3. Insufficient Testing
Agile methodologies stress the importance of testing, often through unit tests and continuous integration. However, in maintenance projects, the focus can skew towards fixing issues rapidly, sometimes at the expense of thorough testing.
Example:
public class IssueTracker {
private Map<String, Boolean> tickets = new HashMap<>();
public void addTicket(String ticket) {
tickets.put(ticket, false); // Not resolved
}
public void resolveTicket(String ticket) {
tickets.put(ticket, true);
// Here we fail to check if the ticket resolution was successful.
}
}
// In a maintenance context, verify if the issue was genuinely resolved.
Why This Matters: This snippet demonstrates insufficient testing. If the ticket resolution does not involve checks or validations, unresolved issues can linger, causing downtime or dissatisfaction.
4. Misalignment of Roles and Responsibilities
Roles in Agile must be clear. In maintenance projects, the responsibilities may become blurred, leading to overlaps or gaps in accountability. This can result in an unwieldy process and poor communication.
Example:
public class Developer {
public void code(String task) {
System.out.println("Coding task: " + task);
}
}
public class Tester {
public void test(String task) {
System.out.println("Testing task: " + task);
}
}
// Lack of clear communication can leave tasks unaddressed.
Why This Matters: As shown, if roles are unclear, critical tasks could fall through the cracks, affecting the project’s agility and overall effectiveness.
5. Short-Term Focus
Agile places a premium on short iterations and quick wins. This can lead to a focus on immediate fixes rather than long-term solutions in maintenance projects. The result is a snowball of tech debt that accumulates over time.
Example:
public class TemporaryFix {
private String solution;
public TemporaryFix(String detail) {
solution = detail;
}
public String getSolution() {
return solution;
}
}
// This may seem like an easy solution, but it's not sustainable.
Why This Matters: Here, the temporary fix can lead to recurrent problems. The approach prioritizes quick solutions that can complicate maintenance in the long run.
Solutions and Best Practices
While these challenges can seem daunting, there are solutions available to help mitigate the risks associated with Agile in maintenance projects:
-
Clarify Requirements: Employ techniques such as user feedback and constant definition of “done” to ensure a shared understanding of requirements.
-
Foster a Culture of Change: Encourage continuous learning and adaptation. Workshops and training can help teams become more proficient with modernizing legacy code.
-
Implement Robust Testing: Use automated tests to ensure that code adjustments do not introduce new issues.
-
Define Roles Clearly: Ensure everyone understands their responsibilities and accountabilities to foster better teamwork.
-
Focus on Long-term Solutions: Strive to balance short-term wins with strategies that reduce technical debt.
The Last Word
Agile principles are undeniably effective; however, their implementation in software maintenance projects requires careful consideration. By acknowledging the fundamental challenges such as unclear requirements, change resistance, and insufficient testing, teams can adapt Agile frameworks to better suit maintenance needs. The goal is not to abandon Agile but to evolve it, ensuring a clearer, sustainable, and smoother software maintenance process.
For further reading on Agile methodologies and their application, check out Scrum.org and Agile Alliance. Understanding these resources will expand your view on how to combine Agile with effective maintenance strategies.
Checkout our other articles