Common Pitfalls in Violating the Single Responsibility Principle
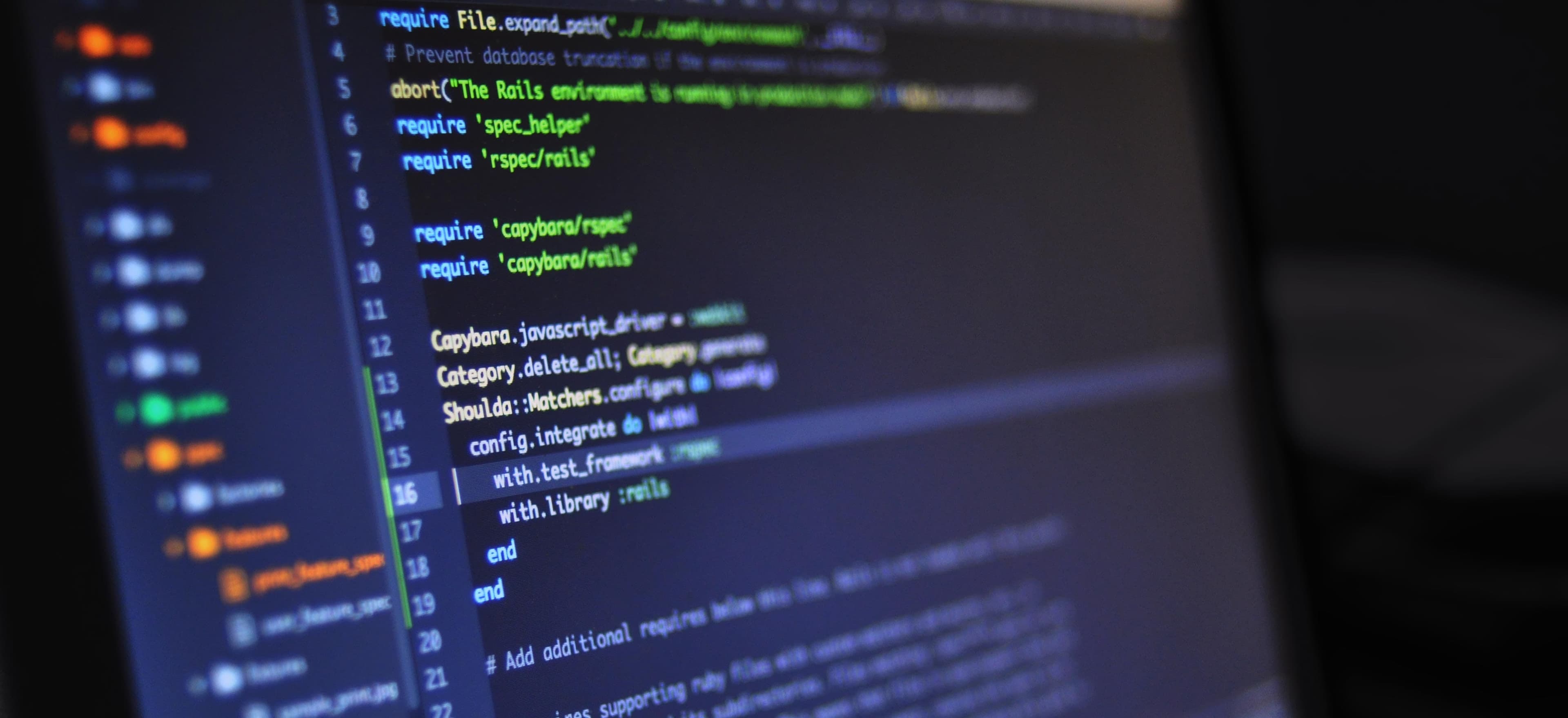
- Published on
Common Pitfalls in Violating the Single Responsibility Principle
The Single Responsibility Principle (SRP) is one of the five SOLID principles of object-oriented programming and design, which advocates that a class should only have one reason to change. In simpler terms, a class should have one job and one job only. This principle aims to make software designs easier to understand, test, and maintain.
This blog post will delve into common pitfalls associated with violating the Single Responsibility Principle, how they manifest in Java code, and strategies to avoid them. By the end, you should have a well-rounded understanding of SRP and its importance in maintaining clean and manageable codebases.
Understanding the Single Responsibility Principle
Before diving into the pitfalls, let’s clarify what SRP means in practical terms. Consider a Java class that handles a banking transaction. If this class both calculates interest and handles the persistence of transaction data (e.g., storing it in a database), it can be said to violate SRP. When changes are necessary—for instance, if the interest calculation logic updates or the data storage method shifts—this class must be modified for multiple reasons, increasing the likelihood of errors.
Here are the key reasons SRP matters:
- Easier to Test: A class that adheres to SRP is easier to test in isolation.
- Improved Readability: Fewer responsibilities make classes more straightforward, enhancing comprehension.
- Reduced Complexity: When classes focus on a single task, the overall complexity of the software architecture decreases.
Common Pitfalls
1. Merging Responsibilities
One of the most common pitfalls in Java programming is merging responsibilities in a single class. Developers may believe that combining various functions will lead to reduced redundancy. However, this often results in a class that has multiple reasons to change.
public class UserManager {
public void createUser(String username) {
// Logic to create a user
}
public void sendEmail(String email) {
// Logic to send email
}
public void logUserAction(String action) {
// Logic to log user action
}
}
Why is this problematic?
The UserManager
class is responsible for user creation, email communication, and logging actions. If a bug is found in the email-sending logic, you may inadvertently impact the user creation functionality when testing or modifying it. Each responsibility can evolve independently, thus violating the SRP.
2. Unnecessary Dependencies
Another common mistake is when classes depend on others to fulfill multiple responsibilities. This increases coupling and makes refactoring more challenging.
public class NotificationService {
private EmailService emailService;
private SMSService smsService;
public void notifyUser(User user, String message) {
emailService.sendEmail(user.getEmail(), message);
smsService.sendSMS(user.getPhoneNumber(), message);
}
}
Why is this problematic?
The NotificationService
class is in charge of handling notifications through both email and SMS. If you need to change how one notification is sent or what service is used, you'll be forced to modify a shared class, leading to the violation of SRP.
3. Large Classes
Large classes can be natural at times, especially when a developer has to add functionalities quickly. However, a large class often indicates that it is trying to do too much.
public class OrderProcessor {
public void processOrder(Order order) {
// Validate order
// Process payment
// Notify user
// Print receipt
// Update inventory
}
}
Why is this problematic?
The OrderProcessor
class handles multiple tasks from validation to notification. Such extensive responsibilities lead to low maintainability and high difficulty in testing. If you need to tweak how to notify users, you might inadvertently break payment processing.
4. Lack of Cohesion
When a class is expected to conduct various operations that seem unrelated, it becomes difficult to discern its primary responsibility, thus breaking SRP.
public class Utility {
public void printReport(String report) {
// Logic to print report
}
public void sendEmail(String emailContent, String recipient) {
// Logic to send email
}
public String createUser(String username) {
// Logic to create a new user
return "User Created";
}
}
Why is this problematic?
This Utility
class is a classic case of lack of cohesion. It offers multiple functionalities that do not relate to a single purpose. As a result, maintaining it becomes cumbersome and confusing.
Strategies to Adhere to SRP
Refactor Regularly
Regular refactoring is essential in preserving SRP in your codebase. Schedule periodic code reviews to identify code smells and potential SRP violations.
Use Interfaces
Consider defining interfaces that correspond to distinct responsibilities. This helps to enforce clear boundaries and cohesive behavior among components.
public interface UserCreation {
void createUser(String username);
}
public interface Notification {
void sendNotification(User user, String message);
}
Leverage Composition
Instead of creating large classes that encompass multiple responsibilities, use composition to combine smaller, focused classes. This promotes reusability and clearer responsibility assignment.
public class OrderProcessor {
private final OrderValidator validator;
private final PaymentProcessor paymentProcessor;
private final NotificationService notificationService;
public OrderProcessor(OrderValidator validator, PaymentProcessor paymentProcessor,
NotificationService notificationService) {
this.validator = validator;
this.paymentProcessor = paymentProcessor;
this.notificationService = notificationService;
}
public void processOrder(Order order) {
validator.validate(order);
paymentProcessor.processPayment(order);
notificationService.notifyUser(order.getUser(), "Your order has been processed.");
}
}
Implement Unit Tests
Writing unit tests for individual responsibilities can help ensure that these classes remain aligned with their single responsibility. It also offers confidence in maintaining and modifying the code in the future.
A Final Look
Violating the Single Responsibility Principle can lead to complex, unmanageable, and fragile code. Throughout this blog post, we navigated common pitfalls such as merging responsibilities, creating unnecessary dependencies, hosting large classes, and lacking cohesion. By recognizing these issues and implementing strategies like regular refactoring, defining interfaces, leveraging composition, and writing unit tests, you can keep your classes focused, maintainable, and easier to work with.
To learn more about design principles and best practices in Java, consider exploring resources like Java Design Patterns and Effective Java. By adhering to principles like SRP, your designs will not only be cleaner but also more robust against the inevitable changes that come with development.