Common Groovy XML Escaping Errors and How to Fix Them
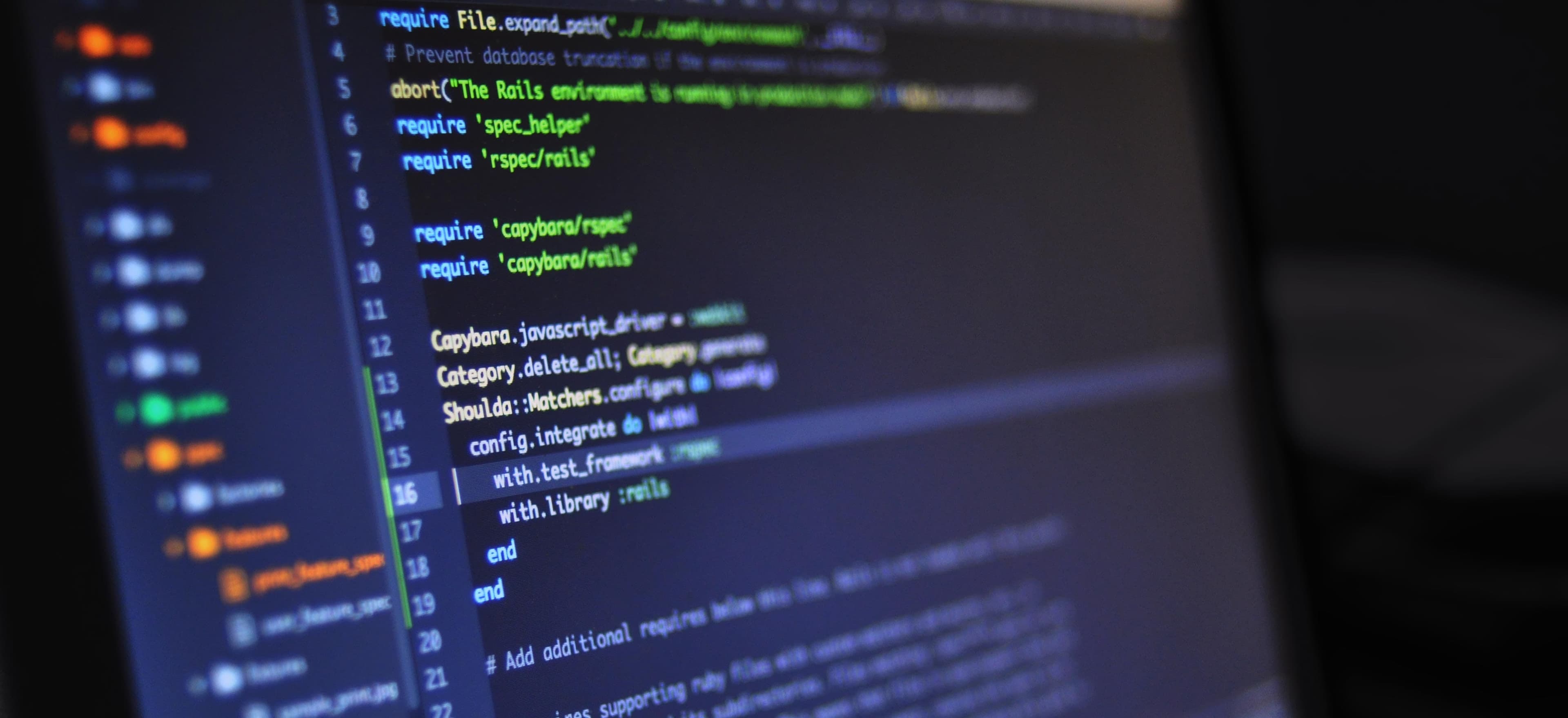
- Published on
Common Groovy XML Escaping Errors and How to Fix Them
When working with XML in Groovy, you may encounter various escaping errors that can lead to improper parsing or unexpected behavior in your applications. Understanding how to handle XML data correctly is crucial for creating robust and reliable software. In this article, we will explore common XML escaping errors in Groovy, provide examples to illustrate these issues, and discuss effective solutions.
Understanding XML Escaping
Before delving into common errors, it’s important to clarify what XML escaping involves. XML requires special characters to be escaped to ensure they are interpreted as data rather than markup. The characters that typically require escaping include:
&
(ampersand) should be escaped as&
<
(less than) should be escaped as<
>
(greater than) should be escaped as>
'
(single quote) should be escaped as'
"
(double quote) should be escaped as"
These characters must be properly escaped to prevent XML parser errors, which can lead to data corruption or application failures.
Common Errors in Groovy XML Escaping
1. Improperly Escaped Ampersands
One of the most common errors occurs when ampersands are not escaped correctly. For example, if you embed an URL, you may inadvertently include an ampersand without escaping it.
Example:
def xmlString = '<url>http://example.com?param1=value1¶m2=value2</url>'
Why This is a Problem:
The XML parser will throw an error when it encounters the ampersand. According to XML standards, it expects a character reference following &
.
Solution:
You should replace the &
with &
.
def escapedXmlString = '<url>http://example.com?param1=value1&param2=value2</url>'
2. Special Characters in Text Content
Another frequent issue occurs when text content within XML elements contains special characters that are not escaped appropriately.
Example:
def xmlString = '<message>This is a test with <, > and & characters.</message>'
Why This is a Problem:
The <
and >
characters will be misinterpreted as XML tags instead of text content, resulting in parsing errors.
Solution:
Make sure to escape both <
and >
.
def escapedXmlString = '<message>This is a test with <, > and & characters.</message>'
3. Escaping in Attributes
When specifying XML attributes, failing to escape quotes properly can lead to syntax errors.
Example:
def xmlString = '<element attribute="This is a "quoted" string"></element>'
Why This is a Problem:
The parser will be confused by the unescaped quotes, breaking the attribute value.
Solution:
Use "
for double quotes inside an attribute.
def escapedXmlString = '<element attribute="This is a "quoted" string"></element>'
4. Not Handling CDATA Properly
When your content includes characters that need to be escaped, using CDATA sections can be an option. However, errors often arise if developers forget to close the CDATA section appropriately.
Example:
def xmlString = '<data><![CDATA[This is a lot of data that includes <, >, and &]]></data>'
Why This is a Problem:
Forgetting to close CDATA can leave a portion of your XML malformed.
Solution:
Ensure that CDATA sections are properly opened and closed.
def validXmlString = '<data><![CDATA[This is a lot of data that includes <, >, and &]]></data>'
5. Mixed Content and Escaping
Mixed content (where text and child elements coexist) can introduce complexity with escaping requirements. This can lead to confusing errors if not handled properly.
Example:
def xmlString = '<note>Hello <to>World</to> & Welcome</note>'
Why This is a Problem:
The presence of &
would mislead the XML parser, creating parsing errors.
Solution:
Be vigilant about escaping all components:
def escapedXmlString = '<note>Hello <to>World</to> & Welcome</note>'
Best Practices for XML Escaping in Groovy
-
Use XML Builders: Groovy provides an easy way to create XML with the
XmlSlurper
orMarkupBuilder
. These builders handle escaping for you automatically, minimizing the risk of errors. -
Utility Functions: Consider creating utility functions that wrap escaping logic, making it reusable and ensuring consistency.
def escapeXml(text) {
return text.replaceAll('&', '&')
.replaceAll('<', '<')
.replaceAll('>', '>')
.replaceAll('\'', ''')
.replaceAll('"', '"')
}
-
Validations: Regularly validate your XML structure using appropriate libraries, such as
XmlParser
orXmlSlurper
, to catch errors early in the development process. -
Unit Tests: Write tests to ensure that your XML data structures render correctly and contain properly escaped content.
-
Be Cautious with User Input: User-generated content can introduce unique escaping issues. Always sanitize and escape external input before including it in your XML structure.
Lessons Learned
XML escaping issues in Groovy can hinder application functionality and lead to frustrating errors. By understanding common pitfalls and adhering to best practices, you can minimize these challenges. Utilize Groovy's XML builders and utility functions to manage escaping effortlessly, ensuring that your XML data remains intact and functional.
For further reading, you may explore the Groovy Documentation for deeper insights into XML handling and advanced use cases.
These strategies will keep your application running smoothly and your data safe from corruption caused by escaping errors. Happy coding!