Common JAXB Log4j XML Configuration Pitfalls to Avoid
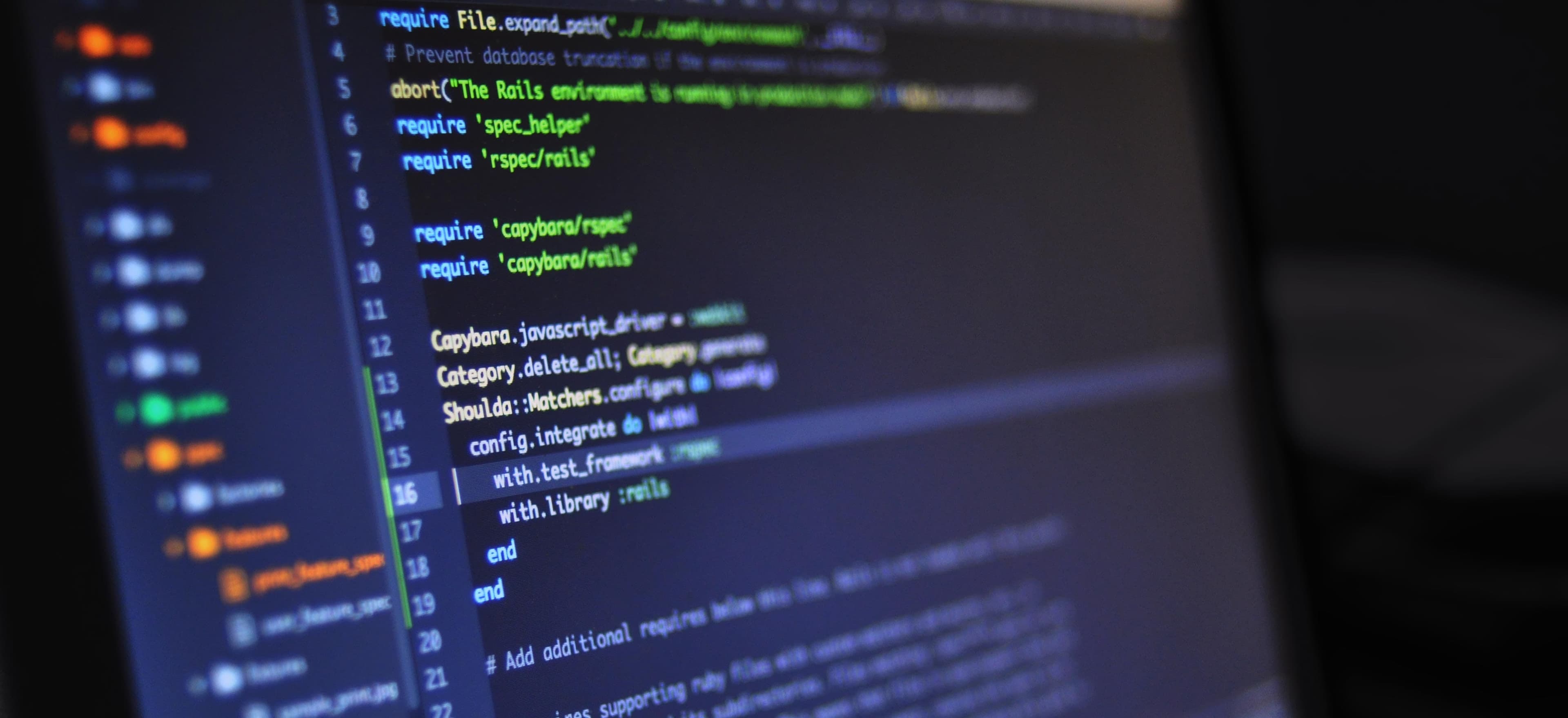
- Published on
Common JAXB Log4j XML Configuration Pitfalls to Avoid
Configuring logging in Java applications can often seem straightforward, yet when dealing with frameworks like Log4j and data binding with JAXB, it's easy to encounter pitfalls that can lead to perplexing runtime issues. This blog post explores common mistakes when working with JAXB and Log4j using XML configurations and provides strategies to avoid them.
Understanding JAXB and Log4j
Before diving into the pitfalls, it’s essential to establish what JAXB and Log4j are and why they are used.
What is JAXB?
Java Architecture for XML Binding (JAXB) is a Java standard for converting Java objects into XML and vice versa. JAXB makes working with XML easier and allows for seamless integration of XML data into Java applications.
What is Log4j?
Log4j is a popular logging library in Java, enabling developers to log messages in a manageable and configurable way. Log4j supports various output destinations (such as console and file) and log levels (such as DEBUG, INFO, WARN, ERROR).
Common Pitfalls and Solutions
1. XML Structure Errors
Pitfall: One of the most common pitfalls in JAXB configuration is an incorrect XML structure. JAXB is strict about the XML schema, and any discrepancy leads to binding exceptions.
Solution: Always validate your XML against the defined schema (XSD
). If you're binding Java objects to XML, ensure that your annotations (like @XmlRootElement
, @XmlElement
) match the XML document structure.
Example Code Snippet
@XmlRootElement(name = "appender")
public class FileAppender {
@XmlElement(name = "name")
private String name;
// getters and setters
}
Why: This annotation specifies that the root element in the XML is <appender>
. Discrepancies between the expected XML and this definition could lead to binding errors.
2. Improper Use of JAXB Annotations
Pitfall: Developers often misunderstand how JAXB annotations work, leading to unwanted behaviors or runtime exceptions.
Solution: Familiarize yourself with the most common JAXB annotations. Annotations like @XmlTransient
are used to skip fields during the XML binding process.
Example Code Snippet
@XmlRootElement(name = "logger")
public class LoggerConfig {
@XmlElement(name = "level")
private String level;
@XmlTransient
private String privateData;
// getters and setters
}
Why: The @XmlTransient
annotation ensures that the privateData
field is never included in the XML representation for logging configuration, thus keeping sensitive information hidden.
3. Classpath Issues
Pitfall: In a complex project structure, certain resources such as XML configuration files may not be on the classpath, leading to file not found exceptions.
Solution: Double-check your project structure. You can use Java’s ClassLoader
to verify that the XML files are accessible.
Example Code Snippet
InputStream inputStream = getClass().getClassLoader().getResourceAsStream("log4j.xml");
if (inputStream == null) {
throw new FileNotFoundException("log4j.xml not found");
}
Why: By ensuring the file is accessible, we mitigate runtime exceptions related to missing configuration files.
4. Ignoring Log4j Best Practices
Pitfall: Log4j has its best practices, including proper logging levels and avoiding excessive logging, which many developers ignore.
Solution: Always configure log levels appropriately. For example, during development, DEBUG may be appropriate, while in production, you often want INFO or WARN levels.
Example Log4j Configuration
<Configuration>
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{HH:mm:ss} %-5p %c{1} - %m%n"/>
</Console>
</Appenders>
<Loggers>
<Logger name="com.example" level="INFO"/>
<Root level="ERROR">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
Why: This configuration ensures that we log important events at the WARN level, avoiding clutter from DEBUG logs in a production environment.
5. Missing Dependencies
Pitfall: Failing to include necessary dependencies in your pom.xml
can lead to issues at runtime.
Solution: Ensure that the following dependencies are included:
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>2.3.0</version>
</dependency>
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
Why: Missing dependencies can lead to ClassNotFoundException
or NoClassDefFoundError
, which hampers functionality and increases debugging time.
6. Misunderstanding Log4j Configuration Loading
Pitfall: Developers assume that Log4j automatically fetches the configuration file without considering priority and loading mechanisms.
Solution: Specify the configuration file location explicitly if necessary.
Example Code Snippet
System.setProperty("log4j.configuration", "file:/path/to/log4j.xml");
Why: This ensures that Log4j uses the specified configuration file, avoiding defaults that may not fit your needs.
7. Failing to Address Performance Issues
Pitfall: Not paying attention to the performance of logging mechanisms can lead to degraded application performance, especially in high-load scenarios.
Solution: Consider asynchronous logging if your application demands high performance.
Example Log4j Configuration
<AsyncRoot level="DEBUG">
<AppenderRef ref="Console"/>
</AsyncRoot>
Why: Asynchronous logging helps offload the I/O operations on a separate thread, allowing the application to maintain responsiveness.
Bringing It All Together
By understanding common pitfalls in JAXB and Log4j XML configuration, you can significantly reduce debugging time and improve application stability. Always remember to validate configurations, adhere to best practices, and ensure that resources are accessible.
For further reading, check out the official JAXB Documentation, and explore Log4j’s official site to understand advanced features.
Avoiding these pitfalls will not only make your application more robust but will also enhance your proficiency in handling XML configurations effectively. Happy coding!
Checkout our other articles