Mastering Java: Tackle NullPointerExceptions Like a Pro
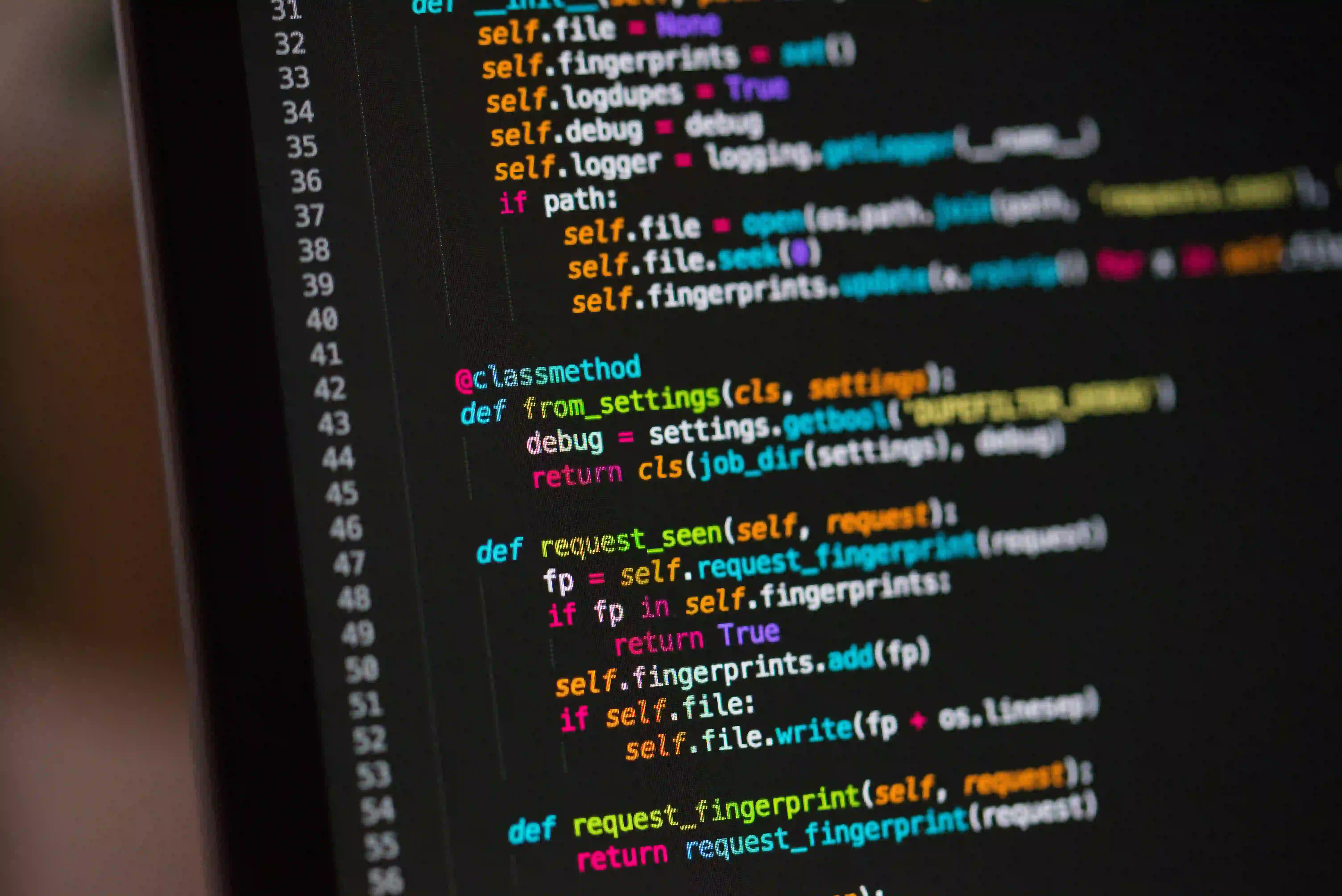
Mastering Java: Tackle NullPointerExceptions Like a Pro
Java developers know that one of the most common hurdles they face is the notorious NullPointerException
(NPE). These exceptions can be frustrating and often lead to hours of debugging, which can derail your development process. In this blog post, we will explore the underlying causes of NullPointerExceptions
, provide actionable strategies to prevent them, and demonstrate best practices through code snippets.
What Is a NullPointerException?
A NullPointerException
occurs in Java when you attempt to use an object reference that has not been initialized or has been set to null
. This situation arises when you try to invoke a method, access a field, or perform an operation on a null object.
Why Are NullPointerExceptions a Pain Point?
- Debugging Difficulty: Debugging a
NullPointerException
can be arduous, especially if the stack trace isn’t clear about where the null reference originated from. - Runtime Errors: They are not caught at compile time, making them more challenging to manage.
- Frequent Occurrences: They are one of the most common exceptions developers encounter.
Let's dive into some effective strategies for handling this headache.
Strategies to Avoid NullPointerExceptions
1. Utilize Java 8 Optional
Java 8 introduced Optional
, a container that can either contain a reference or be empty. Using Optional
helps to express clearly when a value can be absent, reducing the chances of NPE.
Example Code
import java.util.Optional;
public class UserService {
public Optional<User> findUserById(String userId) {
// Simulating data retrieval; it could return null
User user = getUserFromDatabase(userId);
return Optional.ofNullable(user);
}
public void displayUser(String userId) {
Optional<User> user = findUserById(userId);
user.ifPresent(u -> System.out.println("Username: " + u.getUsername()));
}
private User getUserFromDatabase(String userId) {
// Simulate data retrieval that could potentially return null
return null; // For demonstration purposes
}
}
Why Use Optional?
Using Optional
prevents the need for null checks explicitly. The ifPresent()
method executes the specified action if the value is present, thus eliminating the risk of encountering an NPE at this point.
2. Incorporate Null Checks
Always perform null checks before accessing methods or properties of an object.
Example Code
public class OrderService {
public void processOrder(Order order) {
// Check if the order is null
if (order == null) {
throw new IllegalArgumentException("Order cannot be null");
}
// Process the order
System.out.println("Processing order for " + order.getCustomerName());
}
}
Why Perform Null Checks?
A manual null check allows you to handle the situation gracefully, either by throwing an IllegalArgumentException or by providing an alternative behavior.
3. Use Annotations for Clarity
Leverage annotations such as @NonNull
and @Nullable
to clearly document your intentions regarding nullability.
Example Code
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
public class PaymentService {
public void processPayment(@Nonnull Payment payment) {
// This method expects payment to be non-null
System.out.println("Processing payment for " + payment.getAmount());
}
public String getReceipt(@Nullable Order order) {
if (order == null) {
return "No order found";
}
return "Receipt for Order: " + order.getId();
}
}
Why Use Annotations?
Annotations serve as documentation for other developers. Tools like IDEs can also utilize these annotations to give warnings or suggestions, helping prevent NPEs before runtime.
4. Adopt Builder Pattern
Use the Builder Pattern to ensure that all required fields of an object are initialized before it's used.
Example Code
public class User {
private final String username;
private final String email;
private User(UserBuilder builder) {
this.username = builder.username;
this.email = builder.email;
}
public static class UserBuilder {
private String username;
private String email;
public UserBuilder(String username) {
this.username = username;
}
public UserBuilder email(String email) {
this.email = email;
return this;
}
public User build() {
return new User(this);
}
}
}
// Usage:
User user = new User.UserBuilder("john_doe")
.email("john@example.com")
.build();
Why Use Builder Pattern?
The Builder Pattern allows you to create immutable objects. By requiring mandatory fields to be passed during the construction process, you eliminate the possibility of creating an object in an invalid state.
5. Java Null Object Pattern
The Null Object Pattern allows you to represent a default behavior for objects that are essentially "null," thus avoiding useful checks altogether.
Example Code
public interface User {
String getUsername();
}
public class RealUser implements User {
private final String username;
public RealUser(String username) {
this.username = username;
}
@Override
public String getUsername() {
return username;
}
}
public class NullUser implements User {
@Override
public String getUsername() {
return "Guest";
}
}
public class UserFactory {
public static User getUser(String username) {
if (username == null || username.isEmpty()) {
return new NullUser();
}
return new RealUser(username);
}
}
Why Use Null Object Pattern?
The Null Object Pattern provides a default implementation, eliminating null checks and making the code cleaner and easier to maintain.
Closing the Chapter
NullPointerExceptions
can be a common source of stress for developers, but with proper strategies and coding best practices, they can be effectively avoided. By using Java 8's Optional
, performing null checks, utilizing annotations, adopting patterns like the Builder Pattern, and leveraging the Null Object Pattern, you can build robust Java applications that are highly resistant to null-related issues.
For more insights into Java best practices, consider checking out Baeldung's Spring & Java tutorials and Oracle's Java documentation.
Armed with these strategies, you are now better equipped to tackle NullPointerExceptions
like a pro! Happy coding!