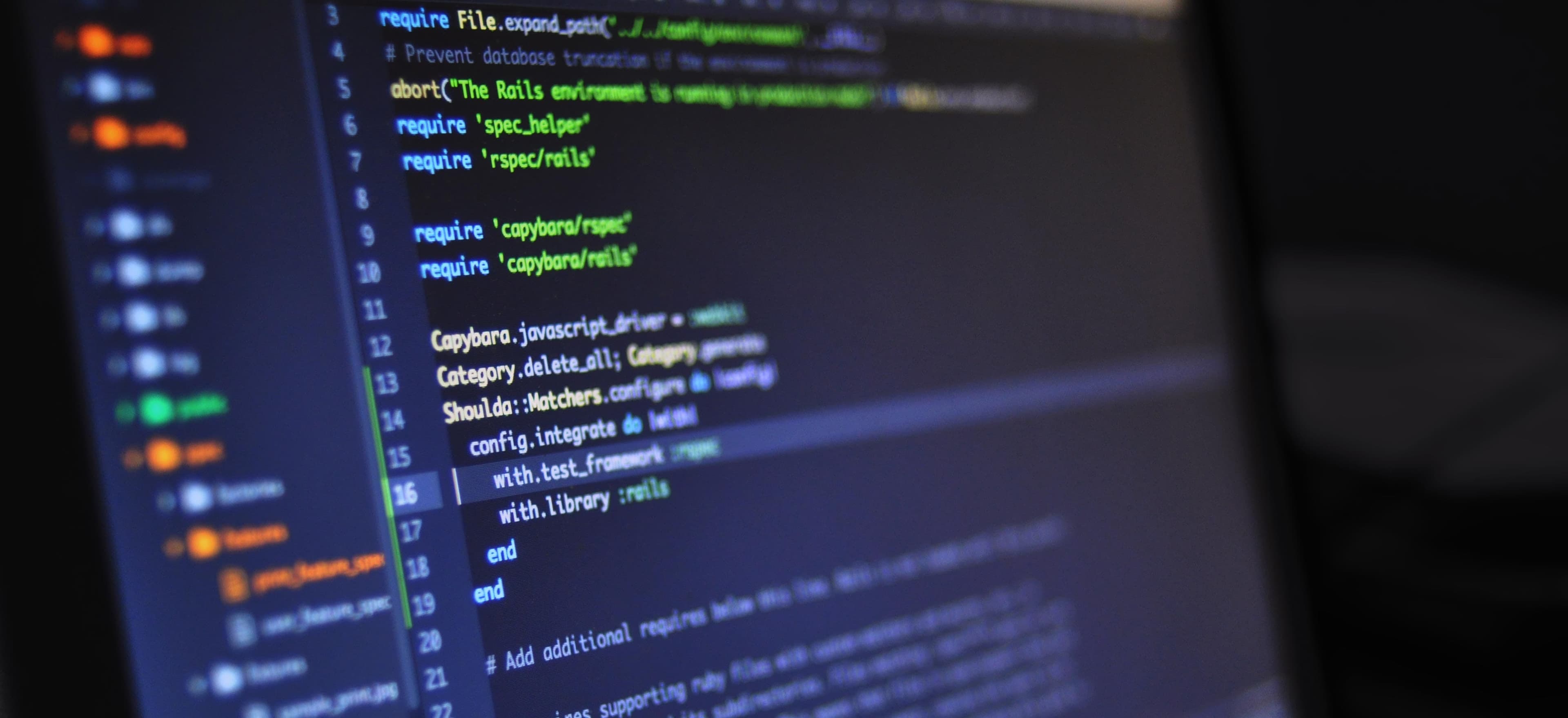
- Published on
Securing Your Java Application with Okta
In the modern age of technology, security is paramount. No matter what type of application you are building, ensuring the safety of user data and resources is critical. When it comes to Java applications, integrating secure authentication and authorization mechanisms is a top priority. One solution that stands out in this domain is Okta.
In this blog post, we will explore how you can secure your Java application using Okta. We will cover the basics of authentication and authorization, and then dive into implementing Okta's authentication and authorization mechanisms in a Java application.
Understanding the Basics
Before delving into the integration of Okta, let's quickly recap the fundamental concepts of authentication and authorization.
Authentication is the process of verifying the identity of a user. It ensures that the user is who they claim to be. Upon successful authentication, the user is granted access to the application.
Authorization, on the other hand, is the process of determining what actions a user is allowed to perform within the application. It involves checking whether the authenticated user has the necessary permissions to carry out specific operations.
Introducing Okta
Okta is an identity and access management (IAM) service that provides secure authentication and authorization for web and mobile applications. It offers a range of features such as user management, single sign-on (SSO), multi-factor authentication, and more.
By integrating Okta into your Java application, you can offload the complexities of user management and authentication to a reliable and trusted service, allowing you to focus on building the core features of your application.
Now, let's get our hands dirty and see how we can integrate Okta into a Java application.
Setting up Okta
First things first, you'll need to sign up for an Okta developer account if you don't already have one. Once you're logged into the Okta dashboard, you can create a new application to obtain the necessary configuration details for integrating Okta into your Java application.
Integrating Okta with Java
Step 1: Adding Okta SDK Dependency
To get started, add the Okta Java SDK dependency to your project. If you're using Maven, you can include the following dependency in your pom.xml
file:
<dependency>
<groupId>com.okta.sdk</groupId>
<artifactId>okta-sdk-api</artifactId>
<version>5.4.0</version>
</dependency>
Step 2: Configuring Okta Client
Next, you'll need to configure the Okta client in your Java application. This involves providing your Okta domain and API token. Here's a sample code snippet demonstrating how you can initialize the Okta client:
import com.okta.sdk.client.Client;
import com.okta.sdk.client.Clients;
public class OktaClientProvider {
private static final String OKTA_DOMAIN = "https://your-okta-domain.com";
private static final String OKTA_API_TOKEN = "your-okta-api-token";
public static Client createClient() {
return Clients.builder()
.setOrgUrl(OKTA_DOMAIN)
.setApiToken(OKTA_API_TOKEN)
.build();
}
}
In this snippet, we use the Okta SDK to create an instance of the Okta Client
by providing the Okta domain and API token.
Step 3: Implementing Authentication and Authorization
With the Okta client configured, you can now proceed to implement authentication and authorization in your Java application. Okta provides comprehensive documentation and libraries to facilitate these processes, including support for various protocols such as OpenID Connect and OAuth 2.0.
For instance, suppose you want to enable single sign-on (SSO) using Okta in your Java application. You can use the Okta Spring Boot starter to effortlessly integrate Okta SSO with your Spring Boot application. The starter provides auto-configuration and pre-built components to simplify the setup process.
Step 4: Adding User Management Features
In addition to authentication and authorization, Okta offers robust user management capabilities. You can leverage Okta's APIs and libraries to create, update, and manage user accounts within your Java application. This includes features such as user registration, password resets, and user profile management.
The Bottom Line
In conclusion, Okta provides a comprehensive set of tools and services to secure your Java application. By integrating Okta's authentication and authorization mechanisms, you can ensure that your application adheres to the highest security standards while offloading the complexities of identity management.
In this blog post, we've only scratched the surface of what Okta has to offer. I encourage you to explore Okta's extensive documentation and resources to discover the full potential of integrating Okta with your Java applications.
So, don't compromise on security – leverage Okta to fortify your Java application and provide your users with a secure and seamless experience.
Now, it's your turn to give it a try and fortify your Java application with Okta!