Common Pitfalls in POS System Architecture Design
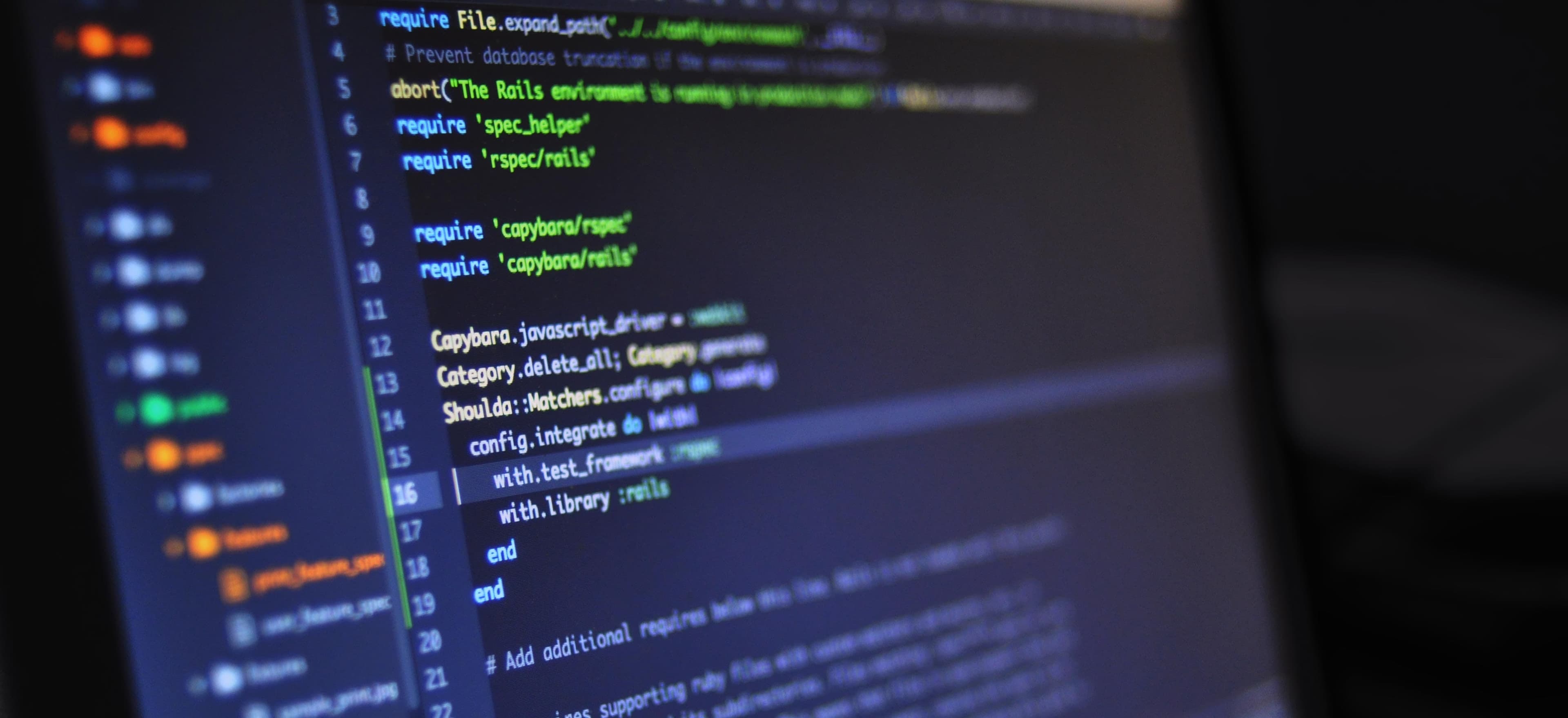
- Published on
Common Pitfalls in POS System Architecture Design
Designing a Point of Sale (POS) system can be a daunting task. It involves meticulous planning and keen attention to detail to ensure the system is efficient, scalable, secure, and user-friendly. In this blog post, we will explore some common pitfalls in POS system architecture design, highlighting why avoiding these mistakes is crucial for your project's success.
1. Underestimating Scalability Needs
The Importance of Scalability
When designing a POS system, one must anticipate future growth. Many tend to design for a limited user base, underestimating potential customer growth. This oversight leads to performance bottlenecks.
Example Code Snippet: Load Balancing
To prepare for scalability, consider implementing a load balancer. Below is a simple example of a load balancer in Java using the Spring Cloud Netflix Eureka framework:
@EnableEurekaClient
@SpringBootApplication
public class PosApplication {
public static void main(String[] args) {
SpringApplication.run(PosApplication.class, args);
}
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
Why This Matters
Using a load balancer distributes incoming requests across multiple servers, allowing your system to handle increased traffic. A lack of this foresight can lead to downtime during peak hours, severely affecting customer experience.
2. Neglecting Security Measures
Security Risks in POS Systems
Security is paramount in POS systems due to the sensitive nature of transaction data. A common pitfall is neglecting to incorporate robust security measures during architecture design.
Key Techniques for Security
Employ encryption and follow best practices in security:
- Use HTTPS: Ensure all web communications are secured.
- Data Encryption: Encrypt sensitive customer data both in transit and at rest.
Example Code Snippet: HTTPS Configuration
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.requiresChannel()
.anyRequest()
.requiresSecure();
}
}
Why This Matters
Using secure channels with HTTPS protects customer data from interception. A breach can lead to loss of reputation and financial penalties, making initial security investment indispensable.
3. Overlooking Integration Capabilities
Integration with Other Systems
A POS system should seamlessly integrate with other existing systems, such as inventory management, CRM, and accounting software. Failing to plan for these integrations can result in silos of information.
Example Code Snippet: RESTful API for Integration
A classic approach to build an integration point involves using RESTful services:
@RestController
@RequestMapping("/api/inventory")
public class InventoryController {
@Autowired
private InventoryService inventoryService;
@GetMapping("/{itemId}")
public ResponseEntity<Item> getItem(@PathVariable Long itemId) {
return ResponseEntity.ok(inventoryService.getItem(itemId));
}
}
Why This Matters
This RESTful API offers a structured way for your POS system to communicate with other software. Lack of integration can lead to delays, inconsistencies, and ultimately zaps your team's productivity.
4. Ignoring User Experience (UX)
Design User-Centric Interfaces
Strong emphasis on system performance and backend logic can overshadow essential frontend elements. A POS system that is difficult to use or slow will frustrate users.
Key UX Principles
- Simplicity: Intuitive layout and navigation.
- Feedback Mechanisms: Real-time feedback for user actions.
Example Code Snippet: A Simple User-Friendly UI
Using JavaFX for the UI layer could provide a responsive experience. For instance:
public class PosUI extends Application {
@Override
public void start(Stage primaryStage) {
Button btn = new Button();
btn.setText("Process Payment");
btn.setOnAction(event -> System.out.println("Payment processed!"));
StackPane root = new StackPane();
root.getChildren().add(btn);
primaryStage.setScene(new Scene(root, 300, 250));
primaryStage.show();
}
}
Why This Matters
A user-friendly interface ensures that employees can quickly learn to use the system, minimizing errors and increasing efficiency.
5. Poor Database Design
The Role of Database in POS
Databases are the backbone of any POS system, yet many developers undermine the importance of well-structured databases, leading to performance issues and data redundancy.
Key Principles of Database Normalization
- Eliminate redundant data.
- Ensure data dependencies make sense.
Example Code Snippet: Normalized Database Schema
Here’s how you might set up a Customers
and Orders
table using SQL:
CREATE TABLE Customers (
CustomerID INT PRIMARY KEY,
CustomerName VARCHAR(100),
ContactEmail VARCHAR(100)
);
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
CustomerID INT,
OrderDate DATETIME,
FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID)
);
Why This Matters
A normalized database prevents data anomalies and improves query performance. Poorly designed databases can lead to slower transactions, resulting in a frustrating customer experience.
6. Weak Testing Strategies
Importance of Robust Testing
Testing is often relegated to the last stage of development, but this can lead to overlooking critical bugs. Designing a testing strategy from the start is essential for a robust application.
Testing Frameworks
Leverage tools like JUnit for unit testing:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class PaymentTest {
@Test
void testCalculateTotal() {
Payment payment = new Payment();
assertEquals(100.0, payment.calculateTotal(100));
}
}
Why This Matters
Thorough testing ensures that your application behaves as expected in real-world scenarios. Otherwise, flaws can go unnoticed until a critical situation arises.
7. Failing to Document the Architecture
The Need for Documentation
Documentation is the unsung hero of successful software projects. Failing to document your architecture can lead to misunderstandings among development teams and result in a knowledge vacuum as team members leave.
Recommended Documentation Practices
- High-level architecture diagrams.
- Detailed user stories.
- API documentation.
Why This Matters
Well-documented systems facilitate easier onboarding for new developers and promote collaborative problem-solving. Not documenting the architecture can significantly hinder team efficiency.
The Bottom Line
Designing a POS system is no small feat. The common pitfalls discussed here—underestimating scalability, neglecting security, overlooking integrations, dismissing user experience, poor database design, weak testing strategies, and failing to document—are obstacles that can severely compromise your system’s effectiveness.
By addressing these challenges head-on with intelligent design principles and robust methodologies, you set yourself and your team up for success. For further reading, check out resources on architecture patterns, or consult documentation on Java security best practices.
In designing a POS system, it’s not merely about crafting code; it’s about creating a resilient and scalable solution that meets both business and customer needs. Stay informed, stay proactive, and your POS system will thrive.