Optimizing Spark Streaming for Efficient Slack Integration
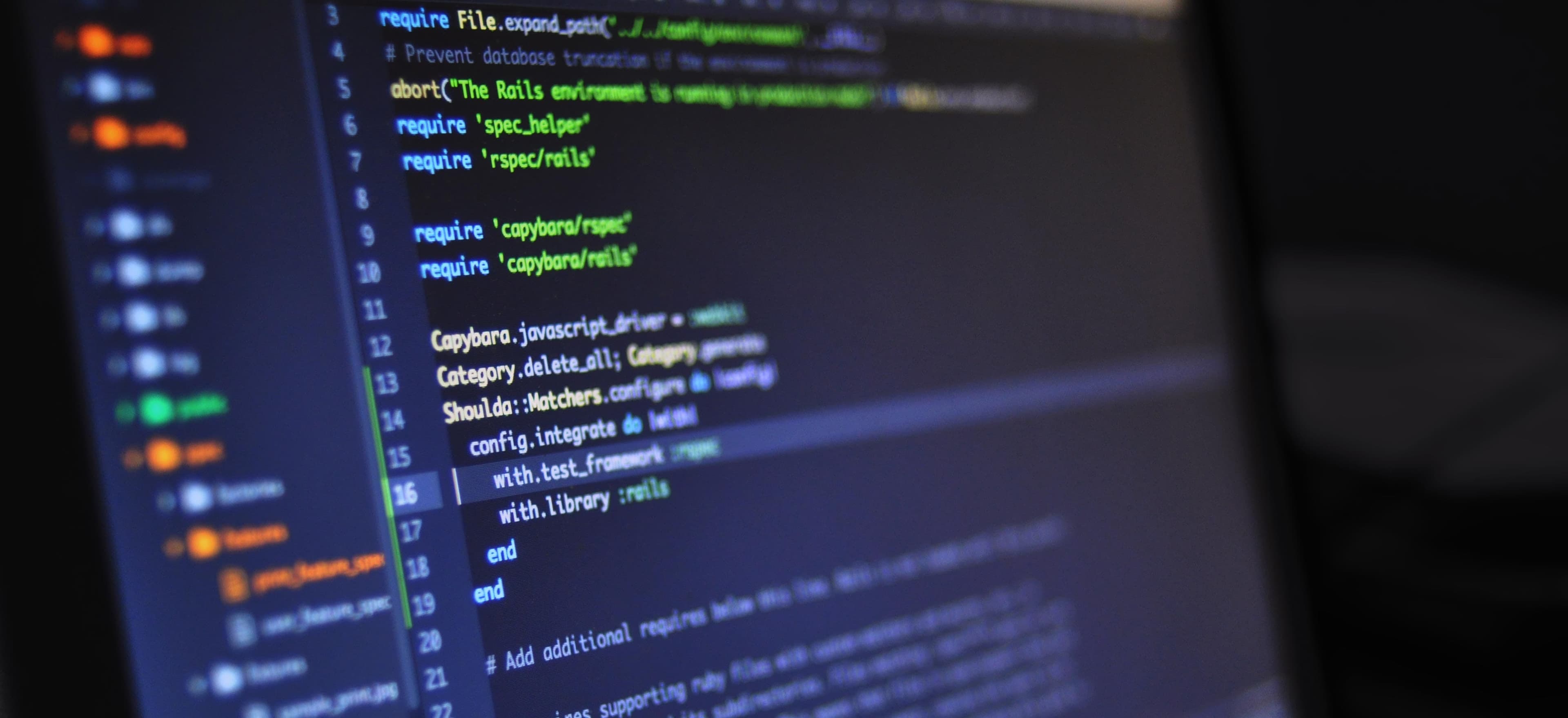
- Published on
Maximizing Efficiency in Slack Integration with Spark Streaming
In today's interconnected digital world, real-time data processing has become a critical component of many applications. Whether it's analyzing social media trends, monitoring server logs, or processing IoT data, the need to ingest and process data in real time is essential. Apache Spark's streaming capabilities provide a powerful framework for real-time data processing, and when combined with popular communication platforms like Slack, it opens up a wide array of possibilities for real-time monitoring, alerting, and decision-making.
This article will delve into maximizing efficiency in integrating Spark Streaming with Slack, ensuring that the entire workflow is optimized for performance and scalability.
Understanding the Integration
Before diving into the technical aspects, let's briefly understand the integration between Spark Streaming and Slack. In this scenario, Spark Streaming would be processing real-time data, and based on certain conditions or events, it would trigger notifications/alerts to be sent to Slack channels or users. This could range from notifying system administrators about critical errors to broadcasting real-time analytics insights to a dedicated channel.
Establishing a Connection to Slack
The first step in the integration process is to establish a connection from Spark Streaming to Slack. Slack provides a well-documented API for sending messages, which can be leveraged from Spark using HTTP libraries like Apache HttpClient or the popular OkHttp library. It's important to manage this connection efficiently, considering factors such as connection pooling, reconnection logic, and overall network efficiency.
An exemplary implementation using OkHttp for sending messages to a Slack channel could look like this:
OkHttpClient client = new OkHttpClient();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\"text\":\"Your message here\"}");
Request request = new Request.Builder()
.url("https://slack.com/api/chat.postMessage?channel={channel_id}")
.method("POST", body)
.addHeader("Authorization", "Bearer {your_token_here}")
.addHeader("Content-Type", "application/json")
.build();
Response response = client.newCall(request).execute();
By using a dedicated HTTP client like OkHttp and managing the request efficiently, we ensure that our connection to Slack is optimized for performance.
Processing Data Efficiently
As with any real-time processing system, the efficiency of data processing is paramount. In the context of Spark Streaming, this involves designing the processing logic to be as streamlined as possible, utilizing appropriate transformations, and considering factors like data partitioning and caching.
Suppose we have a streaming application that processes incoming data and determines when to send notifications to Slack based on certain conditions. It's crucial to design the processing logic with scalability and efficiency in mind. Utilizing appropriate Spark transformations and actions, such as filter
, map
, and foreachRDD
, ensures that the data processing pipeline is optimized for real-time execution.
JavaDStream<YourDataType> inputDataStream = ... ; // Input data stream from a source
inputDataStream.foreachRDD(rdd -> {
rdd.filter(yourCondition)
.foreachPartition(partition -> {
// Initialize the Slack client connection per partition
// Send messages to Slack within the partition
});
});
In the above snippet, we utilize foreachPartition
to process each partition of the RDD independently, thus optimizing resource utilization and parallelism.
Achieving Fault Tolerance
In any real-time system, ensuring fault tolerance is crucial. Spark Streaming provides built-in fault tolerance through mechanisms like checkpointing and managing stateful operations using technologies like Apache Kafka for offset management.
When integrating with Slack, it's important to handle potential failures in sending notifications. This includes retry mechanisms for sending messages, tracking message delivery status, and ensuring that no data loss occurs even in the event of failures.
Scale and Resource Management
Efficiently managing resources and scaling the Spark Streaming application based on workload and data volume is another essential aspect. Leveraging dynamic allocation, where Spark automatically adjusts the resources allocated to the application based on demand, is crucial for optimizing resource utilization.
Additionally, using cluster managers like Kubernetes with Spark can further enhance the scalability and resource efficiency of the Spark Streaming application.
Monitoring and Metrics
Finally, to ensure the efficiency of the entire workflow, it's vital to incorporate comprehensive monitoring and metrics. Tools like Prometheus and Grafana can be integrated with the Spark application to monitor various aspects such as processing throughput, Slack message sending rates, and overall system health. This provides essential insights for performance optimization and proactive issue resolution.
Lessons Learned
Integrating Spark Streaming with Slack for real-time notifications and insights opens up a realm of possibilities, but ensuring optimal efficiency is key to a successful implementation. By focusing on efficient connection management, streamlined data processing, fault tolerance, resource management, and comprehensive monitoring, organizations can harness the full potential of real-time interactions between Spark Streaming and Slack.
Optimizing this integration is a continuous process, evolving alongside the application and organizational needs. By staying mindful of best practices and adapting to changing requirements, the integration can become a robust and efficient mechanism for real-time communication and decision-making.
Incorporating Spark Streaming with Slack can significantly enhance a system's agility and responsiveness, making it an invaluable tool for modern real-time data-driven applications.
For further reference, you can explore more about Apache Spark and Slack API.