Improving Performance: Identifying Hibernate Query Bottlenecks
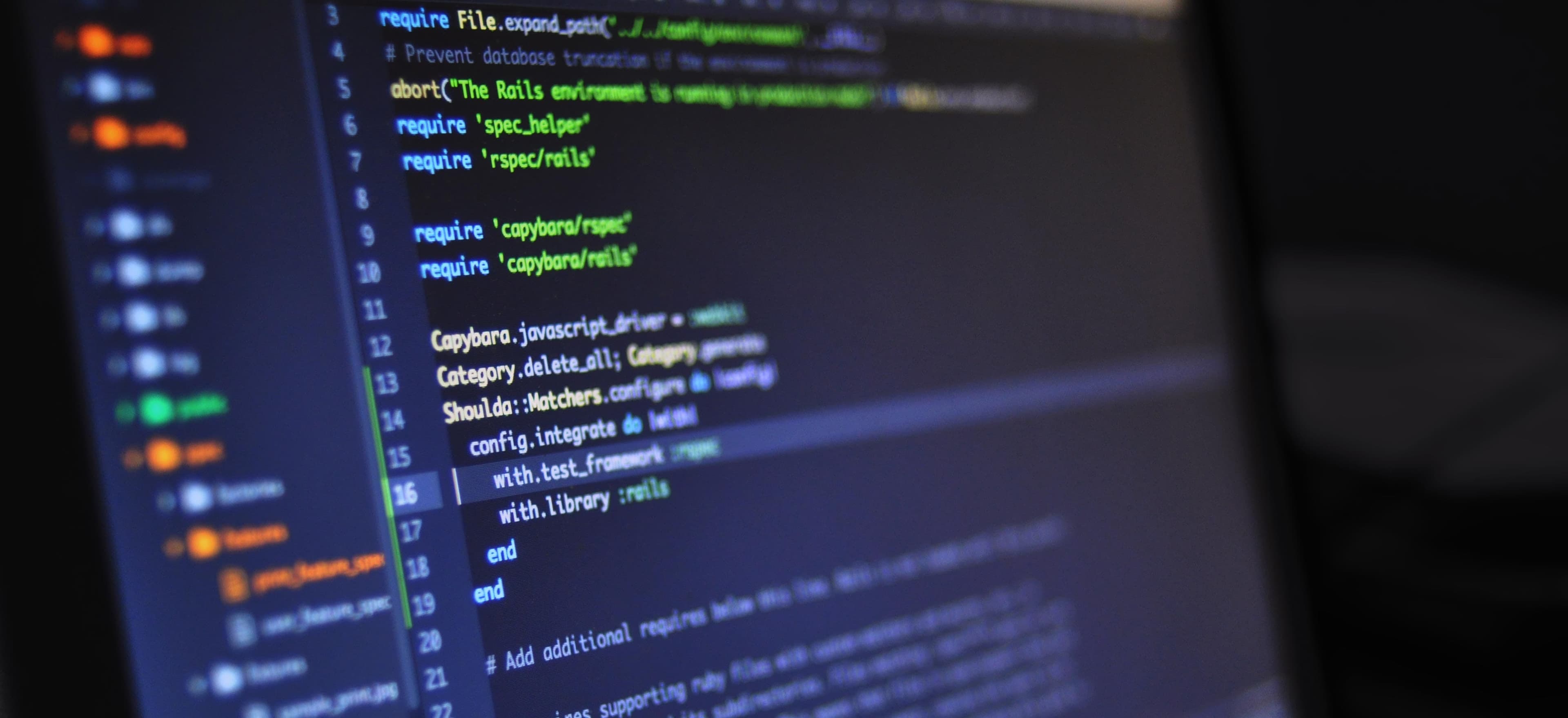
- Published on
Improving Performance: Identifying Hibernate Query Bottlenecks
In a Java application, Hibernate is a powerful tool for object-relational mapping, but like any framework, it comes with its own set of challenges. One common issue developers encounter is identifying and addressing performance bottlenecks in Hibernate queries. In this article, we'll explore some techniques to identify and resolve these bottlenecks, ultimately improving the overall performance of your Hibernate-based application.
Understanding Hibernate Query Performance
Hibernate provides a convenient way to perform database operations using object-oriented approaches, but this convenience can lead to performance issues if not used carefully. When dealing with large datasets or complex queries, it's crucial to understand the underlying SQL generated by Hibernate and how it interacts with the database.
Enable Hibernate SQL Logging
One of the first steps in identifying performance bottlenecks in Hibernate queries is to enable SQL logging. This allows you to see the actual SQL statements being executed by Hibernate, as well as any parameters being passed to these queries. By analyzing these logs, you can gain insights into the efficiency of your queries and identify any potential areas for improvement.
To enable SQL logging in Hibernate, add the following configuration to your application.properties
or hibernate.cfg.xml
:
# application.properties
spring.jpa.show-sql=true
logging.level.org.hibernate.SQL=DEBUG
With SQL logging enabled, you can now analyze the generated SQL statements and optimize them for better performance.
Use FetchType Carefully
In Hibernate, the FetchType
strategy determines how associated data is loaded from the database. The two main strategies are EAGER
and LAZY
. While EAGER
loading fetches the associated data immediately with the main entity, LAZY
loading defers the fetching until the associated data is explicitly accessed.
It's important to use FetchType
carefully, as excessive eager fetching can lead to performance issues by retrieving more data than necessary. On the other hand, lazy loading may result in a large number of database queries being executed, impacting performance.
When designing your entity mappings, consider the access patterns of your application and choose the appropriate FetchType
to balance between minimizing database roundtrips and avoiding unnecessary data retrieval.
Indexing for Performance
Just as in traditional SQL queries, indexing plays a crucial role in optimizing Hibernate query performance. By defining proper indexes on the columns used in your queries, you can significantly reduce the query execution time, especially for large datasets.
Identify the frequently queried columns in your entities and add indexes to those columns in the corresponding database tables. This can dramatically improve the execution time of Hibernate queries that rely on those columns.
Avoid N+1 Query Issues
The N+1 query problem is a common pitfall in Hibernate where the application executes N+1 SQL queries to fetch N entities and subsequently fetch associated entities for each of the N entities individually. This can lead to a large number of unnecessary database roundtrips, significantly impacting performance.
To mitigate the N+1 query issue, use explicit joins or batch fetching to retrieve the associated entities in a more efficient manner. Explicit joins can be achieved using the JOIN FETCH
clause in HQL or Criteria API, ensuring that associated entities are fetched in a single query rather than through multiple roundtrips.
Batch fetching, on the other hand, allows you to configure Hibernate to fetch associated entities in batches, reducing the number of database queries executed.
Leverage Hibernate Caching
Hibernate provides first and second-level caching to reduce the number of database hits and improve query performance. First-level caching is session-specific, meaning if the same entity is requested multiple times within a session, Hibernate retrieves it from the cache rather than hitting the database every time.
Second-level caching is shared across sessions and can be configured to cache entities, queries, or entire collections. By leveraging caching strategically, you can reduce the overhead of repeated database access and improve the overall performance of your Hibernate queries.
To Wrap Things Up
Identifying and addressing performance bottlenecks in Hibernate queries is crucial for building efficient and scalable Java applications. By enabling SQL logging, optimizing FetchType strategies, indexing columns, avoiding N+1 query issues, and leveraging caching, you can significantly improve the performance of your Hibernate-powered application.
To delve deeper into Hibernate performance optimization, consider exploring advanced techniques such as query plan analysis, database schema optimizations, and application profiling. Understanding the intricacies of Hibernate query performance and continuously optimizing your application will contribute to a smoother user experience and better resource utilization.
In conclusion, optimizing Hibernate query performance is an ongoing process that requires a combination of best practices, thorough analysis, and a deep understanding of both Hibernate and database interactions. With these techniques in place, you can ensure that your Hibernate-based application runs efficiently, even under high loads, providing a seamless experience for your users.
Remember, performance optimization is not a one-time task but an ongoing commitment to delivering a responsive and scalable application.
For more in-depth insights into Hibernate performance optimization, you can refer to the official Hibernate documentation. Additionally, exploring specific database optimization techniques, such as indexing best practices, can further enhance the performance of your Hibernate queries.
Keep optimizing, keep innovating, and keep delivering exceptional user experiences with your high-performance Hibernate-based Java applications!
Checkout our other articles