Overcoming CI/CD Challenges with Private Repositories
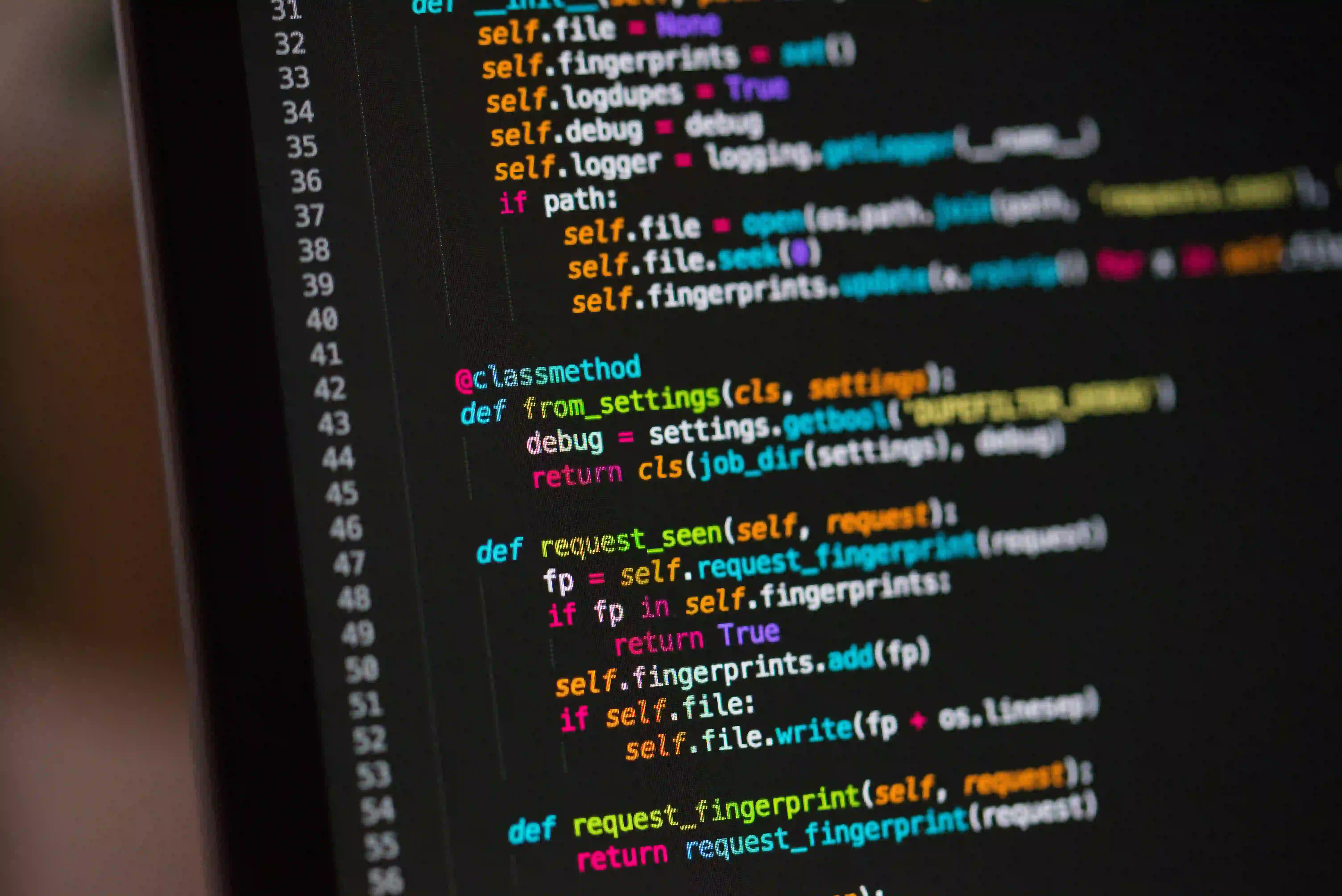
Overcoming CI/CD Challenges with Private Repositories
In the ever-evolving landscape of software development, Continuous Integration and Continuous Deployment (CI/CD) play a pivotal role in ensuring high-quality code delivery. However, when paired with private repositories, developers often face unique challenges. This post will explore these challenges and provide actionable insights to harness the strengths of private repositories effectively.
What is CI/CD?
Before delving into challenges, let's clarify CI/CD.
-
Continuous Integration (CI) is a practice where developers frequently merge their code changes into a central repository. Each integration is verified by an automated build and tests, allowing teams to detect problems early.
-
Continuous Deployment (CD) automates the release process. When code is verified and tested, it goes live automatically without manual intervention.
Why Use Private Repositories?
Private repositories, like those offered by platforms such as GitHub, GitLab, or Bitbucket, allow teams to keep their code secure from unauthorized access. They provide enhanced control over who can view and contribute to the codebase.
However, private repositories come with their own set of challenges, especially when implementing CI/CD pipelines.
Common CI/CD Challenges with Private Repositories
-
Access Management
Managing access permissions can become cumbersome. You need to ensure that only authorized developers have access to specific parts of your codebase. -
Integration Complexity
Integrating CI/CD tools with private repositories requires installing and configuring webhooks, which can lead to misconfigurations. -
Environment Variability
Different environments for testing and production can introduce inconsistencies. Code behavior might change based on configuration settings that are hard to replicate. -
Increased Setup Time
Setting up a CI/CD pipeline for a new project in a private repo often takes longer compared to public repos due to the need for access credentials and environment configurations. -
Dependency Management
Using libraries from private repositories can lead to complications in dependency resolution, making it difficult for CI/CD pipelines to fetch necessary packages for builds. -
Cost Considerations
Many CI/CD providers charge extra for private repositories, which can increase software development costs.
Strategies to Overcome CI/CD Challenges
Now that we understand the challenges, let’s look at practical strategies to mitigate them.
1. Leverage Token-Based Authentication
Instead of traditional username-password combinations, use token-based authentication for accessing private repositories. Tokens are easier to manage and can be scoped for specific permissions.
# To create a personal access token on GitHub, follow these steps:
# 1. Go to Settings > Developer settings > Personal access tokens.
# 2. Generate a new token, selecting the scopes you need (repo, workflow, etc.).
Why?
This approach enhances security and simplifies the authentication mechanism in CI/CD pipelines.
2. Use Environment Variables
Instead of hardcoding sensitive information like API keys or access tokens in your code, use environment variables. Most CI/CD platforms support this feature.
# Sample CI configuration using GitHub Actions
env:
API_TOKEN: ${{ secrets.API_TOKEN }} # Store API Token securely in GitHub secrets
Why?
Environment variables enable a secure method for handling sensitive data, preventing potential leaks.
3. Create Robust Webhook Configurations
Ensure that your webhooks are properly configured to trigger CI/CD processes. Proper testing of webhooks can prevent common pitfalls.
# Sample command to test webhook delivery
curl -X POST -H "Content-Type: application/json" -d '{"ref": "refs/heads/main"}' <WEBHOOK_URL>
Why?
Testing helps confirm that your webhooks are set up correctly and responsive to changes in the repository, reducing the chances of missed triggers.
4. Consistent Environment Setup
Use containers to ensure consistency across different environments. Docker can help encapsulate your application, along with all its dependencies.
# Sample Dockerfile for a Java application
FROM openjdk:11
COPY target/myapp.jar /usr/app/myapp.jar
CMD ["java", "-jar", "/usr/app/myapp.jar"]
Why?
Containers allow developers to run the same application in different environments without worrying about discrepancies, enhancing stability and predictability.
5. Establish Clear Dependency Management Practices
Utilize a dependency management tool like Maven or Gradle in Java projects to streamline handling libraries, including those hosted in private repositories.
Maven Example:
<dependency>
<groupId>com.example</groupId>
<artifactId>my-private-library</artifactId>
<version>1.0.0</version>
<repository>
<id>private-repo</id>
<url>https://your.private.repo/repo</url>
</repository>
</dependency>
Why?
With clear dependency management, you can easily identify and resolve dependencies, making CI/CD processes smoother and more efficient.
6. Automate and Monitor CI/CD Processes
Use CI/CD tools like Jenkins or GitHub Actions to automate processes and monitor their performance. Setting up alerts for failures can help mitigate issues proactively.
# Sample GitHub Actions CI workflow
name: CI
on:
push:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Check out repo
uses: actions/checkout@v2
- name: Build with Maven
run: mvn clean install
- name: Fix permissions
run: chmod +x ./your_script.sh
Why?
Automation saves time and reduces human error. Monitoring ensures that you catch issues early before they affect your production environment.
A Final Look
While private repositories present unique challenges for CI/CD, employing strategic solutions can make the process smoother and more secure. By leveraging tokens, managing dependencies effectively, and automating workflows, teams can ensure that they maintain high standards of code quality and security.
For further reading on CI/CD best practices, you might find these resources helpful:
- Continuous Integration: Improving Software Quality
- Why Use Docker for CI/CD?
By embracing these strategies, teams working with private repositories can overcome obstacles and establish robust, efficient CI/CD pipelines.
This post aims to provide deep insights while still being straightforward and practical. Hopefully, these strategies will assist you in overcoming CI/CD challenges efficiently while working with private repositories.