Common Challenges When Using Spark with Apache Gora
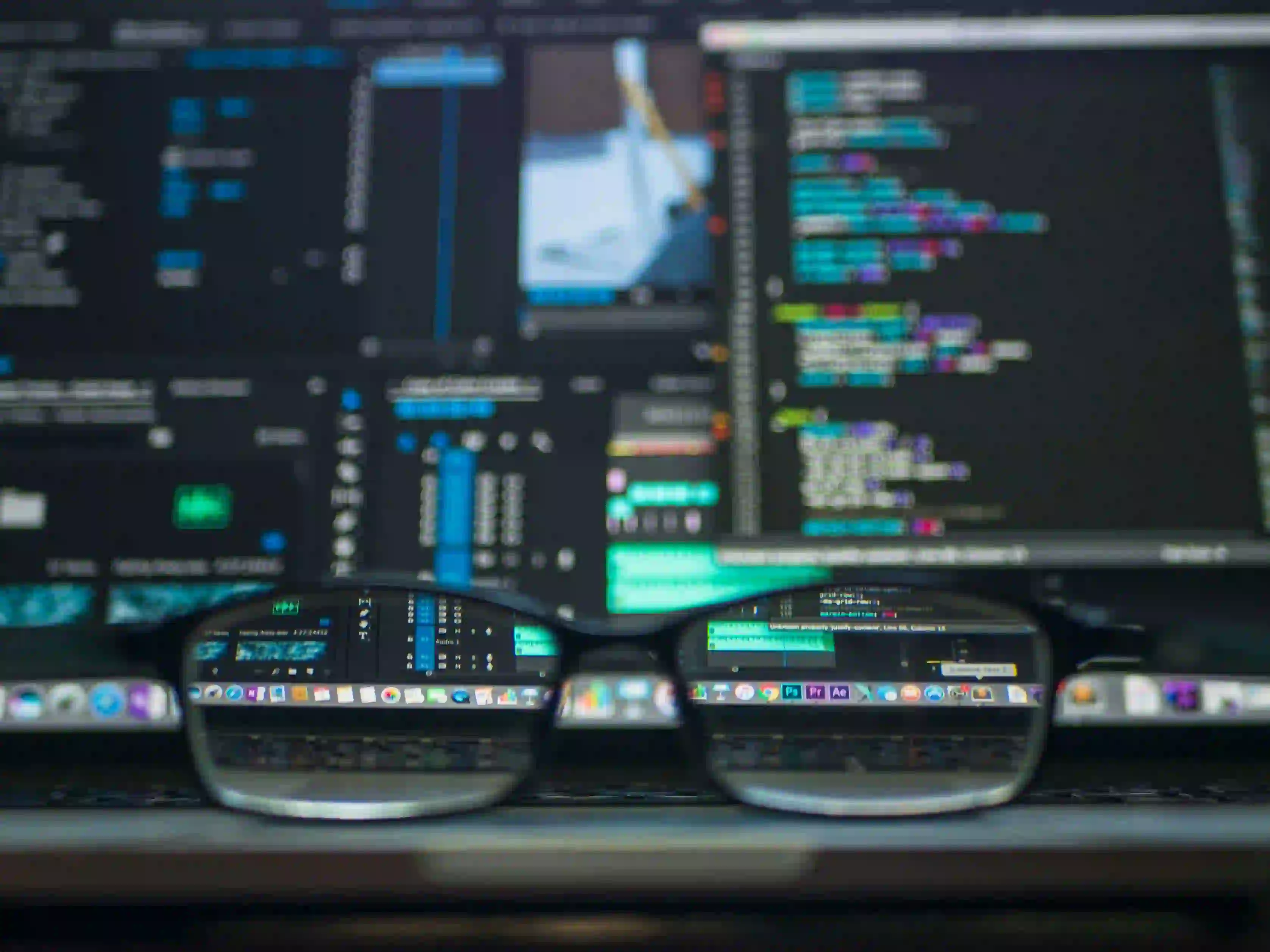
Common Challenges When Using Spark with Apache Gora
Apache Spark and Apache Gora are powerful tools that enable developers to process and manage large-scale data efficiently. However, integrating these two ecosystems can present unique challenges. In this blog post, we will explore some common challenges faced when using Spark with Apache Gora and offer insights on how to overcome them.
Understanding Apache Gora
Apache Gora is a data access framework that allows the handling of various data store backends, like HBase or Cassandra, with a unified API. Gora provides a wide range of features such as schema management and data retrieval which can significantly streamline data processing tasks.
Why Use Apache Spark?
Spark is renowned for its in-memory data processing capabilities. This framework allows for distributed data processing, making it ideal for handling large datasets quickly and efficiently. The combination of Spark and Gora is appealing for big data projects, but it does come with its own set of challenges.
1. Data Compatibility Issues
Challenge
One of the primary challenges is ensuring data compatibility between Spark and the data store managed by Apache Gora. When fetching data, differences in schema or types can lead to runtime errors.
Solution
To mitigate this, it is critical to define the schemas accurately in both Spark and Apache Gora. You can define your Gora schema in a way that it gracefully maps to Spark DataFrame types. Here’s an example of defining a Gora schema:
@SchemaEntity
public class User {
@Column(name = "username", nameType = NameType.String)
private String username;
@Column(name = "age", nameType = NameType.Int)
private int age;
// Getters and Setters
}
By ensuring that your Gora entities align with Spark’s expected data types, you can prevent compatibility issues.
2. Performance Tuning
Challenge
Performance can be a significant bottleneck when working with large datasets. The data retrieval from Apache Gora may not be optimized for Spark, leading to slow processing times.
Solution
To improve performance, start by configuring Apache Gora’s data retrieval parameters. Use batching for reads to reduce the overhead. Here’s a simple example of using batching in Gora:
GoraSQLTable<User> table = ...; // Initialize Gora table
List<User> users = table.getAllUsers(0, 100); // Fetches 100 users at once
This approach minimizes the number of read queries sent to the database, improving overall efficiency. You can also consider caching data in Spark's in-memory storage to speed up repeated access:
val usersDF = spark.read.format("gora").load("your_data_store_path")
usersDF.cache() // Caching the DataFrame
3. Connectivity Issues
Challenge
Connectivity issues can arise when integrating Spark with Gora, especially when dealing with various data backends. Each backend has its own set of configurations and connection protocols.
Solution
Ensure that your Spark application has the necessary dependencies in its build file (like Maven, SBT, etc.) to support Gora. Here’s how you can include Gora in a Maven project:
<dependency>
<groupId>org.apache.gora</groupId>
<artifactId>gora-core</artifactId>
<version>0.10.1</version>
</dependency>
Moreover, validate your connection settings for the Gora backend, as each one requires specific configurations. For example, if you are using HBase, it’s crucial to ensure that HBase is correctly configured to communicate with Spark.
4. Fault Tolerance and Data Loss
Challenge
In distributed systems, fault tolerance is key. There’s always a risk of data loss or corruption during processing, leading to inconsistencies.
Solution
Utilize Spark’s built-in fault-tolerant mechanisms, such as RDD lineage. By ensuring that you understand how data lineage works in Spark, you can effectively recover lost data through recomputation.
Consider the following example of transforming an RDD, which can be recomputed if a failure occurs:
val rdd = sparkContext.parallelize(Seq(1, 2, 3, 4))
val transformedRDD = rdd.map(x => x * 2) // Each operation is recorded in lineage
Should a failure happen during processing, Spark can recover the RDD as it knows how the data was transformed.
5. Complexity in Data Manipulation
Challenge
The complexity of data manipulation across Gora and Spark can be overwhelming for developers. Often, understanding the unique API of Gora while also using Spark's DataFrames can complicate data workflows.
Solution
Leverage Spark SQL capabilities to ease the data manipulation process. With Spark SQL, you can execute complex queries against your Gora-backed data which is presented as tables in Spark. Here’s an example:
usersDF.createOrReplaceTempView("users")
val adultsDF = spark.sql("SELECT * FROM users WHERE age >= 18")
By treating data as tables and utilizing SQL-like queries, you simplify the data manipulation process significantly.
6. Lack of Comprehensive Documentation
Challenge
Both Apache Spark and Apache Gora are evolving tools, but the documentation may not cover every integration aspect in detail. This can lead to confusion.
Solution
Stay up-to-date with the latest releases and refer to community forums or the official Apache Gora documentation for insights. Participating in community discussions can also provide helpful context and solutions from other users.
A Final Look
While combining Apache Gora with Apache Spark can present challenges, it also opens the door to a powerful data processing framework. By addressing data compatibility issues, optimizing performance, ensuring reliable connectivity, and leveraging Spark's resilience features, you can create a robust data processing system.
As you explore these technologies, remember that challenges are part of the learning process. Overcoming them will enhance your proficiency and empower you to build efficient big data solutions. Happy coding!