Mastering Object Creation: Common Mistakes to Avoid
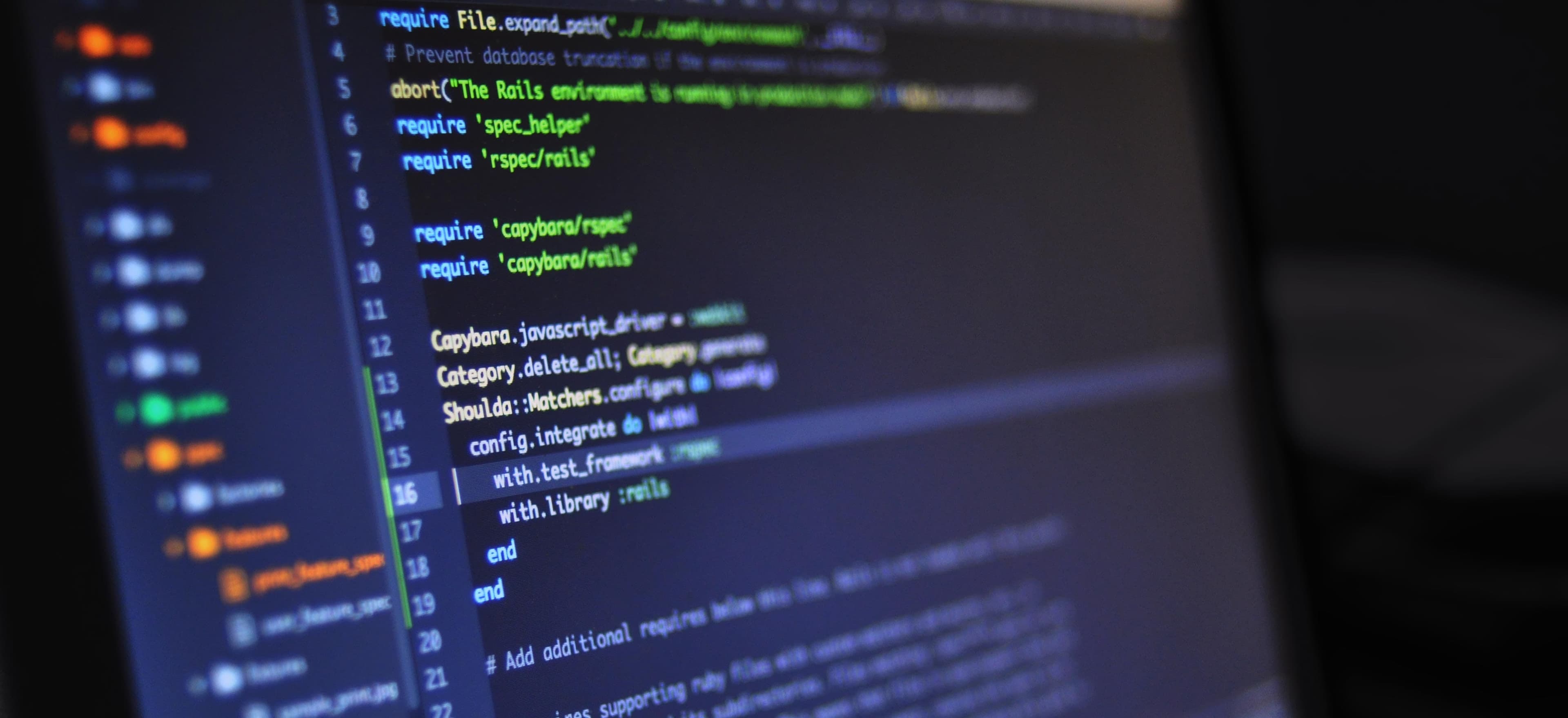
- Published on
Mastering Object Creation in Java: Common Mistakes to Avoid
Java, known for its object-oriented paradigms, offers powerful mechanisms for creating and managing objects. However, many developers, especially those new to Java, fall into common pitfalls that can lead to code that is difficult to read, maintain, and debug. This blog post will walk you through the common mistakes in object creation in Java and how to avoid them, ensuring your code is efficient and elegant.
Why Object Creation Matters
In Java, everything revolves around objects. They are fundamental to the language's structure, representing real-world entities. Understanding the nuances of object creation can help you manage memory effectively, improve performance, and ensure maintainability.
Common Mistakes and How to Avoid Them
1. Not Using Constructors Properly
Constructors in Java are special methods called when an object is instantiated. Failing to use them properly can lead to confusing code.
Example Mistake: Default Constructors Misuse
class Car {
private String model;
public Car() {
this.model = "Unknown"; // Default value
}
public void displayModel() {
System.out.println("Car model: " + model);
}
}
Why This is a Mistake:
While the default constructor sets a default value, it can lead to ambiguity, especially if multiple constructors are involved. New developers may assume the model is set without realizing it defaults to "Unknown".
Best Practice: Use Parameterized Constructors
class Car {
private String model;
public Car(String model) {
this.model = model; // Explicitly set during instantiation
}
public void displayModel() {
System.out.println("Car model: " + model);
}
}
Using parameterized constructors keeps your object initialization clear and explicit.
2. Uncontrolled Object Creation
Creating too many objects in a short amount of time can lead to performance issues due to excessive memory allocation.
Example Mistake: Excessive Object Creation in a Loop
for (int i = 0; i < 100000; i++) {
Car car = new Car("Car " + i);
// Do something with car
}
Why This is a Mistake:
Creating a new object for every iteration can lead to memory bloat and decreased performance due to garbage collection overhead.
Best Practice: Object Pooling
Consider utilizing an object pool to reuse existing objects, especially for expensive resources:
class CarPool {
private List<Car> availableCars = new ArrayList<>();
public Car getCar() {
if (availableCars.isEmpty()) {
return new Car("New Car");
} else {
return availableCars.remove(availableCars.size() - 1);
}
}
public void returnCar(Car car) {
availableCars.add(car);
}
}
Implementing an object pool can improve performance significantly by minimizing object churn.
3. Ignoring Immutability
Java objects can be mutable or immutable. Ignoring the benefits of immutability can lead to bugs.
Example Mistake: Mutable Object State
class User {
private String username;
public User(String username) {
this.username = username;
}
public void setUsername(String username) {
this.username = username; // Mutable state
}
}
Why This is a Mistake:
Mutating the state of objects can introduce unintended side effects, especially in multi-threaded environments.
Best Practice: Enforce Immutability
class User {
private final String username;
public User(String username) {
this.username = username;
}
public String getUsername() {
return username;
}
}
Making the fields final
and providing no setters help ensure immutability, which is especially useful in concurrent programming.
4. Failing to Override toString
Method
When debugging, having a human-readable string representation of an object can be invaluable. Many developers forget this step.
Example Mistake: Missing toString
Override
class Book {
private String title;
private String author;
// Constructors omitted
// No toString method
}
Why This is a Mistake:
Attempting to print a Book
object will provide an uninformative string like Book@5b2133c
, which is not useful for debugging.
Best Practice: Override toString
@Override
public String toString() {
return "Book{title='" + title + "', author='" + author + "'}";
}
By overriding the toString
method, you provide meaningful output that can enhance debugging and logging.
5. Neglecting the Use of Factory Methods
Developers often miss the opportunity to use factory methods to create objects, which can encapsulate complex creation logic.
Example Mistake: Direct Initialization
class Vehicle {
private String type;
public Vehicle(String type) {
this.type = type;
}
}
// User code
Vehicle car = new Vehicle("Car");
Why This is a Mistake:
The above code is fine for simple objects but can get cumbersome if the initialization process is complex.
Best Practice: Implement Factory Methods
class VehicleFactory {
public static Vehicle createCar() {
return new Vehicle("Car");
}
public static Vehicle createTruck() {
return new Vehicle("Truck");
}
}
// User code
Vehicle car = VehicleFactory.createCar();
Factory methods can encapsulate construction logic, making it easier to manage and modify.
Additional Resources
For more in-depth knowledge about object-oriented programming in Java, check out the Java Documentation or explore Effective Java by Joshua Bloch, which provides comprehensive insights on best practices.
The Last Word
In conclusion, mastering object creation in Java is essential for writing clean, efficient, and maintainable code. By avoiding the common mistakes discussed in this article and adhering to best practices, you can ensure that your code remains robust and easy to work with. Keep learning, keep coding, and embrace the power of Java's object-oriented features. Happy coding!
Checkout our other articles