Securing Secrets for Tomcat Service in Amazon ECS
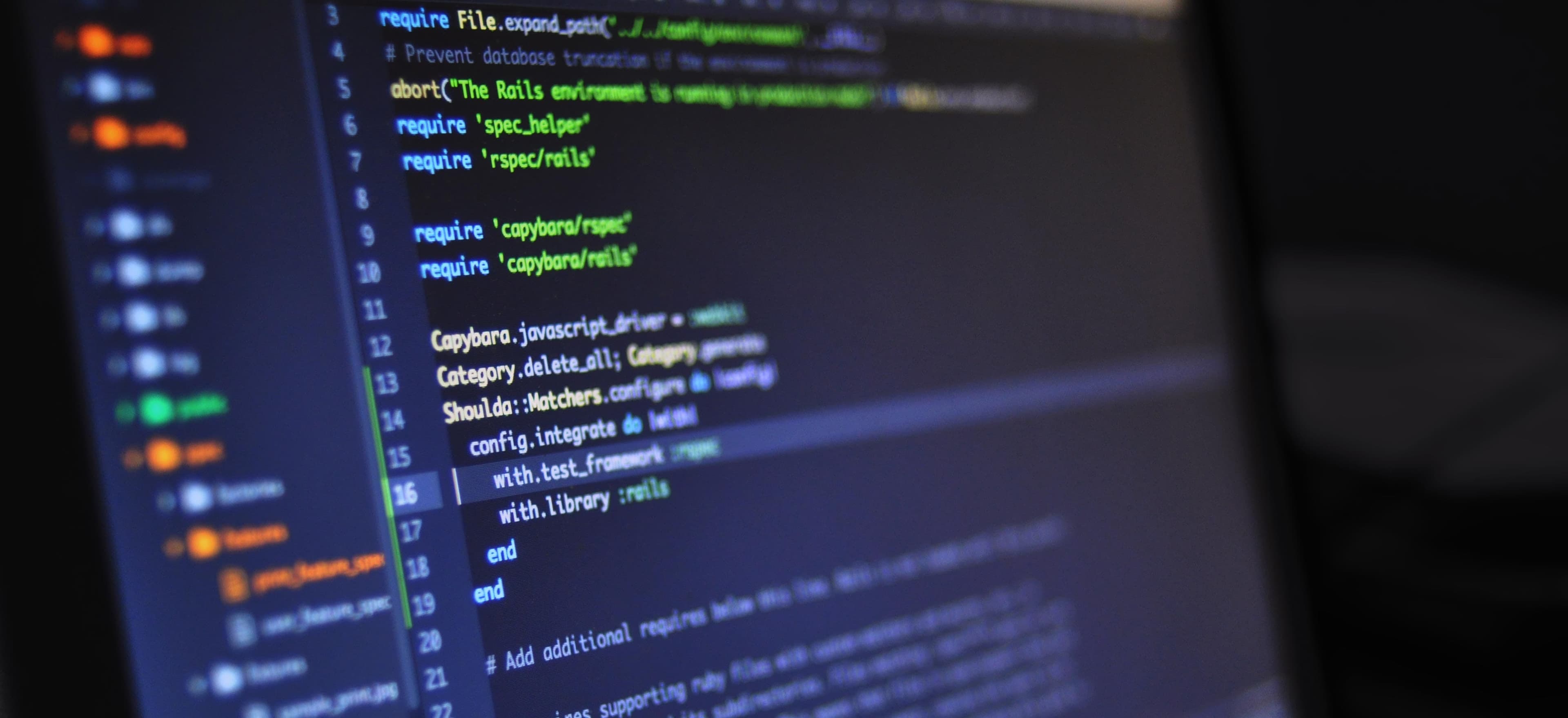
- Published on
Securing Secrets for Tomcat Service in Amazon ECS
When deploying a Java application on Amazon ECS, ensuring that sensitive data such as credentials are securely managed is crucial. In this blog post, we will explore how to secure secrets for a Tomcat service running in Amazon ECS.
Using Environment Variables
One common approach to managing secrets in Amazon ECS is through environment variables. When deploying a Tomcat service, sensitive information such as database credentials can be passed as environment variables.
public class DatabaseConfiguration {
private String username;
private String password;
public DatabaseConfiguration() {
this.username = System.getenv("DB_USERNAME");
this.password = System.getenv("DB_PASSWORD");
}
// Getters and setters
}
By reading the sensitive data from environment variables, the application can securely access the required credentials without hardcoding them within the codebase.
AWS Systems Manager Parameter Store
Another approach is to use AWS Systems Manager Parameter Store to securely store and retrieve sensitive data. This can be achieved by creating a SecureString parameter in Parameter Store and granting the ECS task execution role permission to access the parameter.
public class DatabaseConfiguration {
private String username;
private String password;
public DatabaseConfiguration() {
AWSSimpleSystemsManagement ssmClient = AWSSimpleSystemsManagementClient.builder().build();
GetParameterRequest parameterRequest = new GetParameterRequest()
.withName("/myapp/db/password")
.withDecryption(true);
GetParameterResult parameterResult = ssmClient.getParameter(parameterRequest);
this.username = "mydbuser";
this.password = parameterResult.getParameter().getValue();
}
// Getters and setters
}
By utilizing Parameter Store, the application can securely fetch the sensitive data without exposing it directly within the code or environment variables.
AWS Secrets Manager
For a more advanced and comprehensive way of managing secrets, AWS Secrets Manager can be used. This service provides built-in integration with Amazon ECS, making it easy to retrieve credentials securely at runtime.
public class DatabaseConfiguration {
private String username;
private String password;
public DatabaseConfiguration() {
AWSSecretsManager client = AWSSecretsManagerClient.builder().build();
GetSecretValueRequest secretRequest = new GetSecretValueRequest()
.withSecretId("myapp/db");
GetSecretValueResult secretResult = client.getSecretValue(secretRequest);
this.username = "mydbuser";
this.password = secretResult.getSecretString();
}
// Getters and setters
}
By leveraging AWS Secrets Manager, the application can securely retrieve the sensitive data, benefiting from its robust security features and seamless integration with Amazon ECS.
Wrapping Up
Securing secrets for a Tomcat service in Amazon ECS is paramount for maintaining the confidentiality and integrity of sensitive information. By utilizing approaches such as environment variables, AWS Systems Manager Parameter Store, and AWS Secrets Manager, Java applications can access credentials securely without compromising on best security practices.
For further information, refer to the following resources:
By integrating these secure practices, Java developers can ensure that their applications running in Amazon ECS are well-protected against unauthorized access to sensitive data.