Effective Selenium Test Automation with Maven
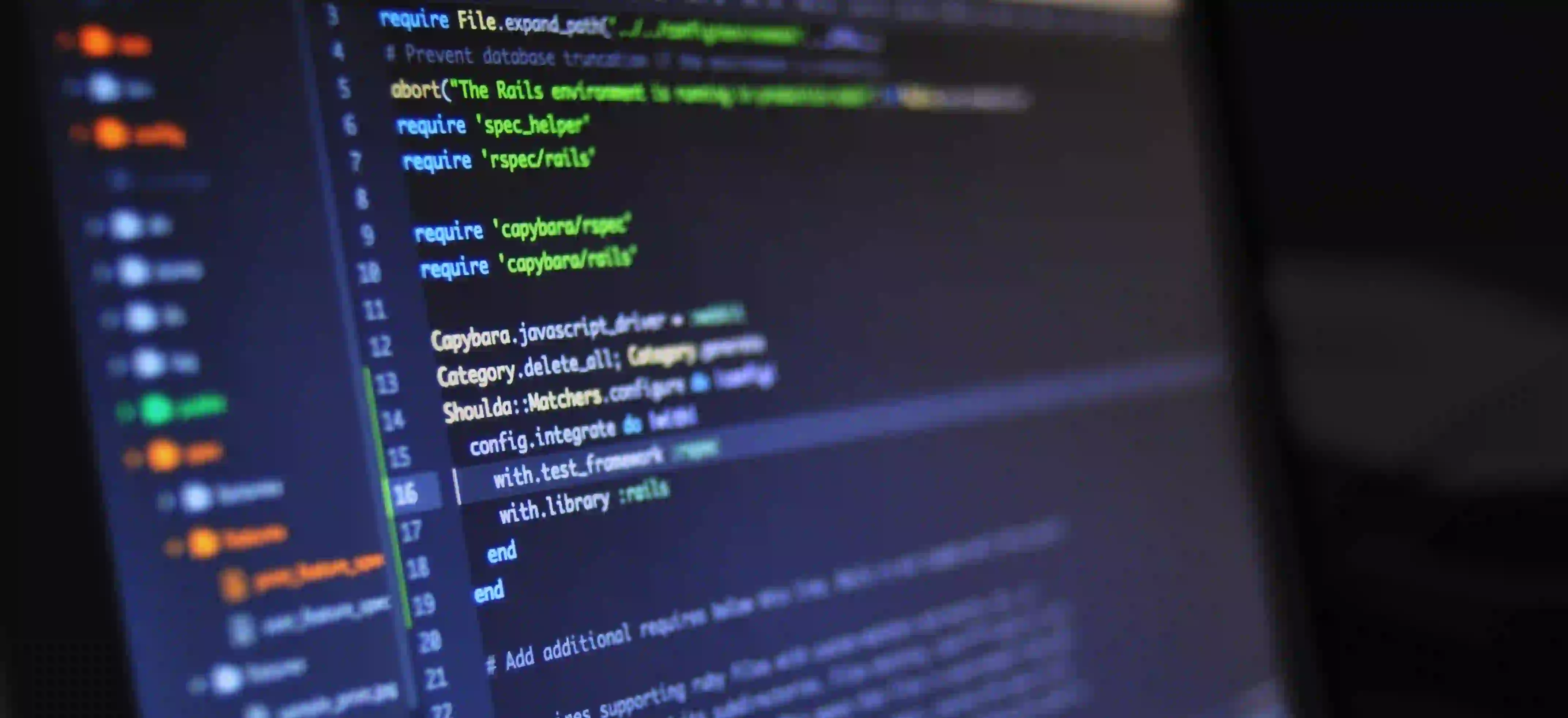
Effective Selenium Test Automation with Maven
In the world of software development, automation testing has become an integral part of the overall development process. Selenium, a powerful and versatile automation tool, has gained widespread popularity for automating web applications. When it comes to managing dependencies and building automation projects, Maven stands out as a robust project management and comprehension tool.
This blog post will delve into the seamless integration of Selenium with Maven for effective test automation. We will explore the benefits of using Maven for Selenium automation, discuss best practices, and provide a step-by-step guide to set up a Selenium test automation project using Maven.
Benefits of Using Maven for Selenium Automation
1. Dependency Management
Maven simplifies the process of managing dependencies in Selenium automation projects. It allows us to define and centralize project dependencies, making it easier to add, update, or remove libraries and drivers necessary for Selenium testing.
2. Build Lifecycle
Maven provides a well-defined build lifecycle, which allows for the execution of various phases such as compilation, testing, packaging, and deployment in a sequential manner. This ensures consistency and reliability in the build process of Selenium automation projects.
3. Plugin Support
Maven offers a wide range of plugins for tasks such as generating reports, executing tests, and integrating with CI/CD tools. This extensibility facilitates the seamless integration of Selenium automation with different tools and processes.
With these benefits in mind, let's dive into the process of setting up a Selenium test automation project with Maven.
Setting Up a Selenium Test Automation Project with Maven
Step 1: Prerequisites
Before starting, ensure that Java and Maven are installed on your system. Additionally, have a clear understanding of the Selenium WebDriver and its usage for automating web browsers.
Step 2: Create a Maven Project
Use the following Maven command to create a new Maven project. Replace groupId
, artifactId
, and version
with appropriate values.
mvn archetype:generate -DgroupId=com.example.selenium -DartifactId=selenium-automation -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Step 3: Add Selenium Dependencies
Open the pom.xml
file in the project directory and add the Selenium WebDriver dependency within the <dependencies>
section.
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version>
</dependency>
Step 4: Create a Selenium Test
Create a new Java class for your Selenium test under the src/test/java
directory. In this class, instantiate a WebDriver instance, write test scenarios, and perform necessary assertions.
public class SeleniumTest {
WebDriver driver;
@Before
public void setUp() {
// Initialize the WebDriver (e.g., ChromeDriver, FirefoxDriver)
driver = new ChromeDriver();
}
@Test
public void testTitle() {
driver.get("https://example.com");
String title = driver.getTitle();
Assert.assertEquals("Example Domain", title);
}
@After
public void tearDown() {
if (driver != null) {
driver.quit();
}
}
}
Step 5: Execute the Tests
Run the Selenium tests using Maven by executing the following command in the project directory:
mvn test
Best Practices for Selenium Test Automation with Maven
1. Organize Test Code
Maintain a clear and organized directory structure for test code, page objects, and resources within the Maven project. This improves code maintainability and readability.
2. Use Page Object Model (POM)
Implement the Page Object Model design pattern to create reusable and efficient Selenium tests. Separate the page-specific locators and interactions from test logic, promoting better code maintainability.
3. Leverage Maven Profiles
Utilize Maven profiles for managing environment-specific configurations, such as different browser configurations or test data sets. This allows for streamlined execution of tests across various environments.
4. Incorporate Reporting and Logging
Integrate reporting and logging plugins for generating comprehensive test reports and logging test execution details. This aids in tracking test results and identifying issues effectively.
Key Takeaways
In conclusion, the integration of Selenium with Maven significantly enhances the efficiency and manageability of test automation projects. By leveraging Maven's robust dependency management, build lifecycle, and plugin support, Selenium test automation becomes a streamlined and scalable process. Adhering to best practices such as organizing test code, implementing the Page Object Model, leveraging Maven profiles, and incorporating reporting and logging further elevates the effectiveness of Selenium test automation with Maven.
With the step-by-step guide and best practices outlined in this post, you are well-equipped to embark on your Selenium test automation journey with Maven and drive the quality and reliability of your web applications to new heights.
Start integrating Maven with Selenium today and witness the power of efficient test automation firsthand.
For more in-depth understanding of Maven, you can check out this Maven in 5 Minutes guide and explore further possibilities for your automation journey. Additionally, to gain deeper insights into Selenium WebDriver, the official Selenium Documentation serves as an invaluable resource.
Happy automating!