Choosing the Right Testing: Unit vs. Integration
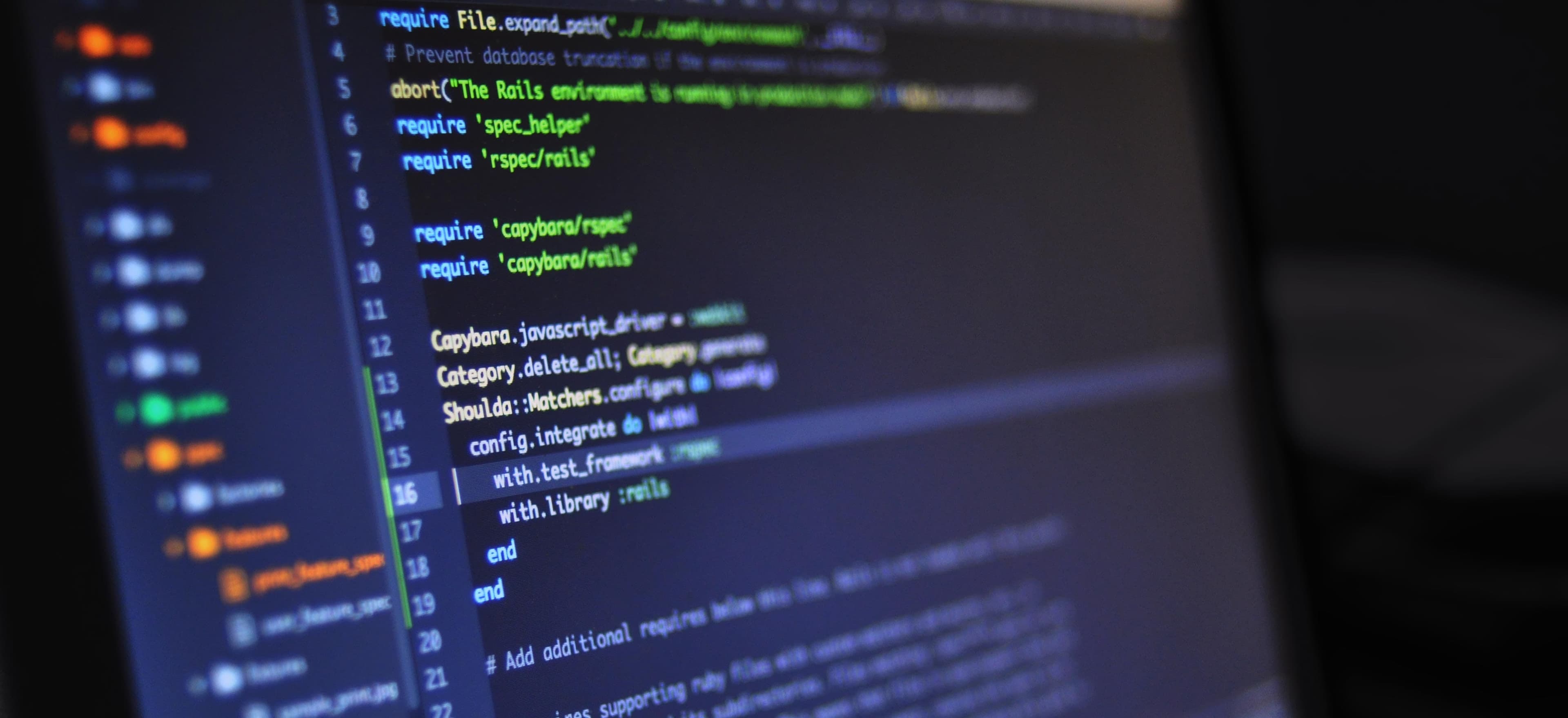
- Published on
When it comes to testing in software development, two types of tests play a crucial role in ensuring the quality and reliability of the codebase: unit tests and integration tests. While both are essential, understanding the differences between them and knowing when to use each is key to building robust and maintainable applications. In this article, we will delve into the specifics of unit tests and integration tests, explore their unique qualities, and discuss when it is appropriate to use each type.
What are Unit Tests?
Unit tests are focused, small-scale tests that verify the behavior of individual components in isolation. These components could be methods, functions, or classes, and the purpose of unit testing is to validate that each unit of code performs as expected. Mock objects or stubs are often used to isolate the unit under test from its dependencies, ensuring that the test focuses solely on the logic within the unit.
Advantages of Unit Tests
- Early Bug Detection: Unit tests can catch bugs early in the development cycle, making it easier and more cost-effective to fix them.
- Independent Verification: Since unit tests are designed to be independent of one another, they can be run in any order, facilitating parallel execution and helping to identify faulty units quickly.
- Improved Code Quality: Writing unit tests encourages modular and loosely coupled code, leading to better design and maintainability.
Example of a Unit Test
Consider a simple method that calculates the factorial of a given number:
public class MathUtils {
public int factorial(int number) {
if (number < 0) {
throw new IllegalArgumentException("Number should not be negative");
}
if (number == 0) {
return 1;
}
return number * factorial(number - 1);
}
}
A corresponding unit test for the factorial
method can be written as follows:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class MathUtilsTest {
@Test
public void testFactorial() {
MathUtils mathUtils = new MathUtils();
int result = mathUtils.factorial(5);
assertEquals(120, result);
}
}
In this example, the unit test ensures that the factorial
method produces the correct result for a given input. It focuses solely on the behavior of the factorial
method without considering its interactions with other code.
What are Integration Tests?
While unit tests validate individual units of code, integration tests verify the interaction and integration of multiple components or modules within a system. Integration tests aim to uncover defects arising from the interoperation of integrated components, including issues such as data flow, APIs, and database interactions.
Advantages of Integration Tests
- End-to-End Verification: Integration tests validate the interactions between various parts of the system, ensuring that the integrated components work as expected when combined.
- Real-world Scenarios: Integration tests often simulate real-world scenarios, such as interactions with databases, network requests, and external services, providing confidence in the system's behavior in a production environment.
- Comprehensive Testing: Integration tests can uncover issues that are not evident in unit tests, especially related to the integration points between components.
Example of an Integration Test
Consider a simple application that interacts with a database to retrieve and process data. An integration test for this scenario might involve setting up a test database, populating it with sample data, running the application, and verifying that the expected results are obtained.
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class DatabaseIntegrationTest {
@Test
public void testDatabaseInteraction() {
// Set up test database
// Populate test data
// Execute application functionality
// Verify expected results
assertEquals(expectedResult, actualResult);
}
}
In this example, the integration test ensures that the application integrates correctly with the database and produces the expected output, simulating a real-world scenario involving database interactions.
Choosing the Right Testing Approach
So, when should you use unit tests, and when should you opt for integration tests? The answer lies in understanding the specific purpose and scope of each testing approach, as well as the trade-offs associated with each.
Use Unit Tests When:
- Testing Isolated Components: Use unit tests when you need to verify the behavior of isolated units of code, such as methods or functions, without considering their interactions with other parts of the system.
- Fast Feedback Loop: Unit tests are typically fast to execute, making them suitable for providing quick feedback during the development process.
- Testing Edge Cases and Logic: Unit tests are well-suited for testing edge cases, error conditions, and intricate logic within individual units of code.
Use Integration Tests When:
- Validating System Interactions: Integration tests should be used to validate interactions between integrated components, such as database operations, network communications, and API integrations.
- End-to-End Scenarios: When you want to test end-to-end scenarios involving multiple components working together, integration tests are the most appropriate choice.
- Real-world Scenario Validation: Use integration tests to verify the behavior of the system in a more realistic environment, including interactions with external dependencies and services.
It's important to note that a balanced testing strategy often involves a combination of both unit tests and integration tests, with each serving a distinct purpose in ensuring the quality and reliability of the software. While unit tests focus on isolating and validating individual units of code, integration tests complement this by verifying the integrated behavior of multiple components.
In conclusion, understanding the differences between unit tests and integration tests, as well as knowing when to apply each type of testing, is crucial for establishing a comprehensive testing strategy. By leveraging the strengths of both unit tests and integration tests, developers can achieve a robust and reliable codebase that meets the demands of modern software development.
Final Thoughts
In this article, we have explored the distinct characteristics of unit tests and integration tests, highlighting their respective advantages and best use cases. By understanding the role of each type of testing and knowing when to apply them, developers can build resilient and high-quality software that meets the expectations of users and stakeholders.
In the constantly evolving landscape of software development, testing remains an indispensable practice for ensuring the functionality, reliability, and maintainability of code. As such, mastering the art of choosing the right testing approach—whether unit tests or integration tests—will undoubtedly contribute to the success of software projects, empowering teams to deliver exceptional solutions in today's dynamic marketplace.