Boosting Log4j 2 Performance: Tackling Latency Issues
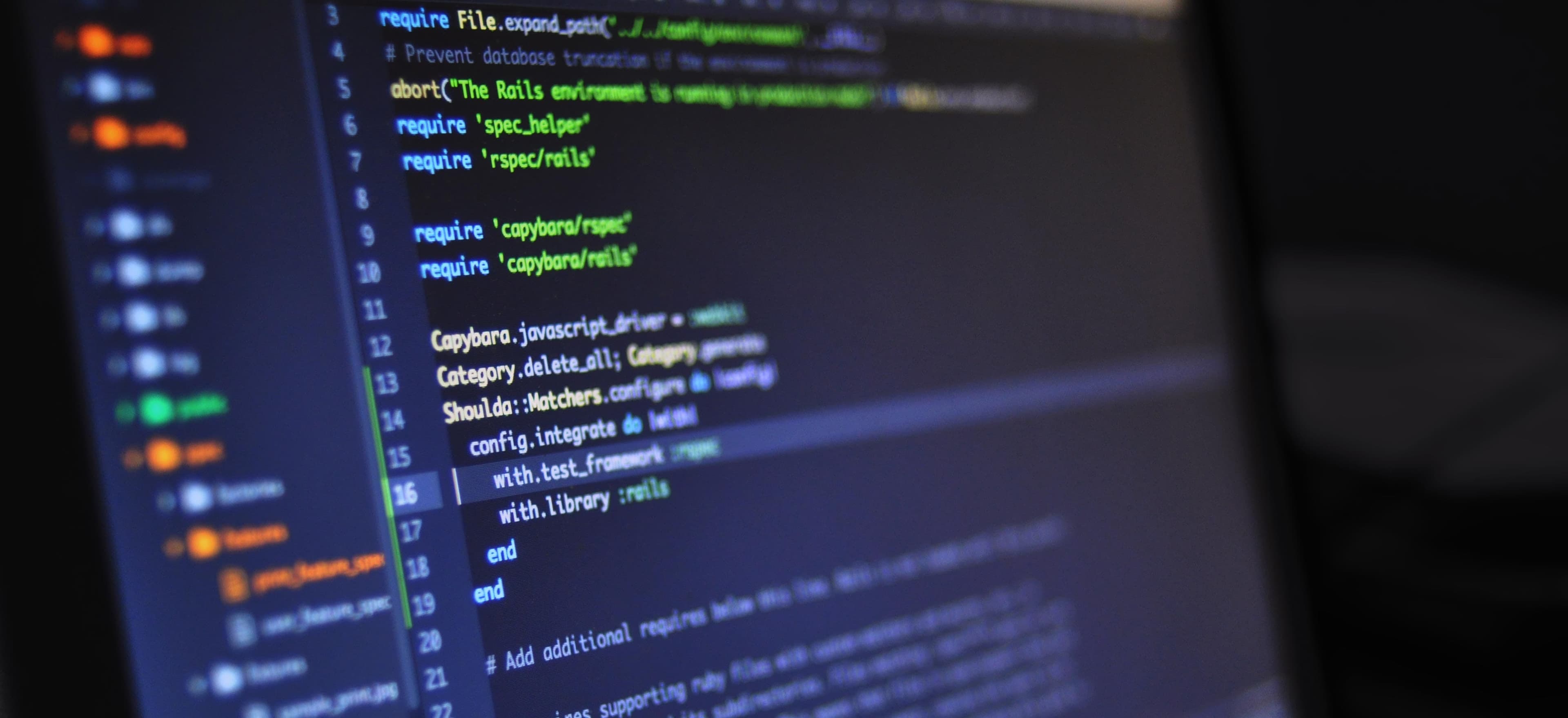
- Published on
Boosting Log4j 2 Performance: Tackling Latency Issues
In today's fast-paced software development environment, effective logging is crucial. As applications scale, ensuring that logging mechanisms do not become bottlenecks is essential. Log4j 2, one of the most popular logging frameworks for Java applications, offers several features that cater to high-performance logging. This blog post will explore methods to enhance Log4j 2 performance by tackling latency issues, providing code snippets, and discussing best practices to maximize efficiency.
Understanding Latency in Logging
Latency in logging refers to the delay encountered when writing log messages, especially in high-throughput applications. High latency can lead to performance degradation, as logging operations could slow down application response times.
To effectively mitigate this, we first need to understand what contributes to logging latency:
- I/O Operations: Writing logs to disk or sending them over the network can be time-consuming. Synchronous I/O operations block execution until complete, adding to latency.
- Log Level Filtering: Evaluating log levels can add overhead, especially if numerous logging statements are present in performance-critical code paths.
- Logging Formats: Complex log formats that require additional processing can increase latency.
Strategies to Reduce Log4j 2 Latency
1. Asynchronous Logging
One of the most effective ways to reduce latency is asynchronous logging. Log4j 2 supports asynchronous logging through the AsyncAppender
class, which allows log events to be queued and processed in a separate thread.
Example Code: Asynchronous Logging Configuration
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="WARN">
<Appenders>
<Async name="AsyncLogger">
<File name="FileLogger" fileName="logs/app.log" />
</Async>
</Appenders>
<Loggers>
<Root level="debug">
<AppenderRef ref="AsyncLogger"/>
</Root>
</Loggers>
</Configuration>
Why This Matters: Asynchronous logging decouples log writing from application execution, minimizing the impact of logging on performance. The events are processed in a separate thread, allowing the application to continue running without waiting for I/O operations.
2. Use of RollingFileAppender
In high-volume applications, log files can grow rapidly, affecting performance during write operations. The RollingFileAppender
can automatically manage log file sizes, keeping them from becoming bloated.
Example Code: RollingFileAppender Configuration
<Appenders>
<RollingFile name="RollingFileLogger" fileName="logs/app.log"
filePattern="logs/app-%d{yyyy-MM-dd}-%i.log.gz">
<PatternLayout>
<Pattern>%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1} - %m%n</Pattern>
</PatternLayout>
<Policies>
<SizeBasedTriggeringPolicy size="10MB"/>
<TimeBasedTriggeringPolicy interval="1" modulate="true"/>
</Policies>
<DefaultRolloverStrategy max="10"/>
</RollingFile>
</Appenders>
Why This Matters: Rolling file management helps prevent files from becoming excessively large, which can lead to slow read/write operations. By leveraging policies, you can automatically roll over logs based on size and time, simplifying log management.
3. Appropriate Log Level Configuration
Logging at the right level is essential. Using DEBUG or TRACE in production can lead to unnecessary log generation, increasing processing time. Restricting log levels to INFO or WARN will significantly reduce the overhead.
Example Code: Logger Configuration
<Loggers>
<Logger name="com.myapp" level="info" additivity="false">
<AppenderRef ref="RollingFileLogger"/>
</Logger>
<Root level="warn">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
Why This Matters: Setting the logger level appropriately ensures that only essential logs are recorded. This not only improves performance but also reduces noise, making it easier to identify important information.
4. Optimize Layouts
Log4j 2 supports various layout formats. The chosen layout can impact the processing overhead associated with log messages. Opt for simpler layouts when high performance is necessary.
Example Code: Using SimplePatternLayout
<PatternLayout pattern="%d{HH:mm:ss} [%t] %-5p %c - %m%n"/>
Why This Matters: A simpler log format reduces processing time when constructing log entries. Avoid unnecessary complexity in your layouts to maintain logging speed.
5. Buffering and Flushing
Buffering log messages can significantly reduce the number of I/O operations. Log4j 2 provides options for buffered writes through the BufferedIO
property in your configuration.
Example Code: Buffered File Appender
<Appenders>
<File name="BufferedFileLogger" fileName="logs/app.log" bufferedIO="true" bufferSize="8192">
<PatternLayout>
<Pattern>%d{yyyy-MM-dd HH:mm:ss} - %m%n</Pattern>
</PatternLayout>
</File>
</Appenders>
Why This Matters: Buffering allows multiple log writes to occur in a single I/O operation, thereby reducing latency. It significantly enhances performance in high-throughput scenarios by minimizing the context-switching caused by frequent I/O operations.
6. Monitor Performance
Finally, continuously monitoring performance provides insight into the effectiveness of your logging strategy. Utilize tools like JMX or application performance monitoring (APM) solutions to track logging impact on your application.
Why This Matters: Adapting logging strategies based on actual performance metrics can lead to ongoing improvements. Identify problematic areas and iterate to find the optimal configuration for your needs.
The Bottom Line
Optimizing Log4j 2 performance is a vital concern for developers, particularly in high-volume applications. By leveraging asynchronous logging, managing log file sizes with RollingFileAppender
, appropriately configuring log levels, simplifying layouts, optimizing buffering, and actively monitoring performance, you can significantly reduce logging latency.
For more information on Log4j 2 features and optimizations, consider checking out the official Log4j documentation and additional resources on performance tuning.
By implementing these strategies, you will ensure that log4j 2 serves as a beneficial tool in your development environment rather than a hindrance. Remember, effective logging leads to enhanced application reliability and better user experiences.
By following these steps and understanding the 'why' behind each configuration, developers can ensure that Log4j 2 enhances application performance rather than detracts from it. Be proactive, always assess how your logging practices align with your performance goals!