Choosing Between Data-Driven and Logic-Based Algorithms
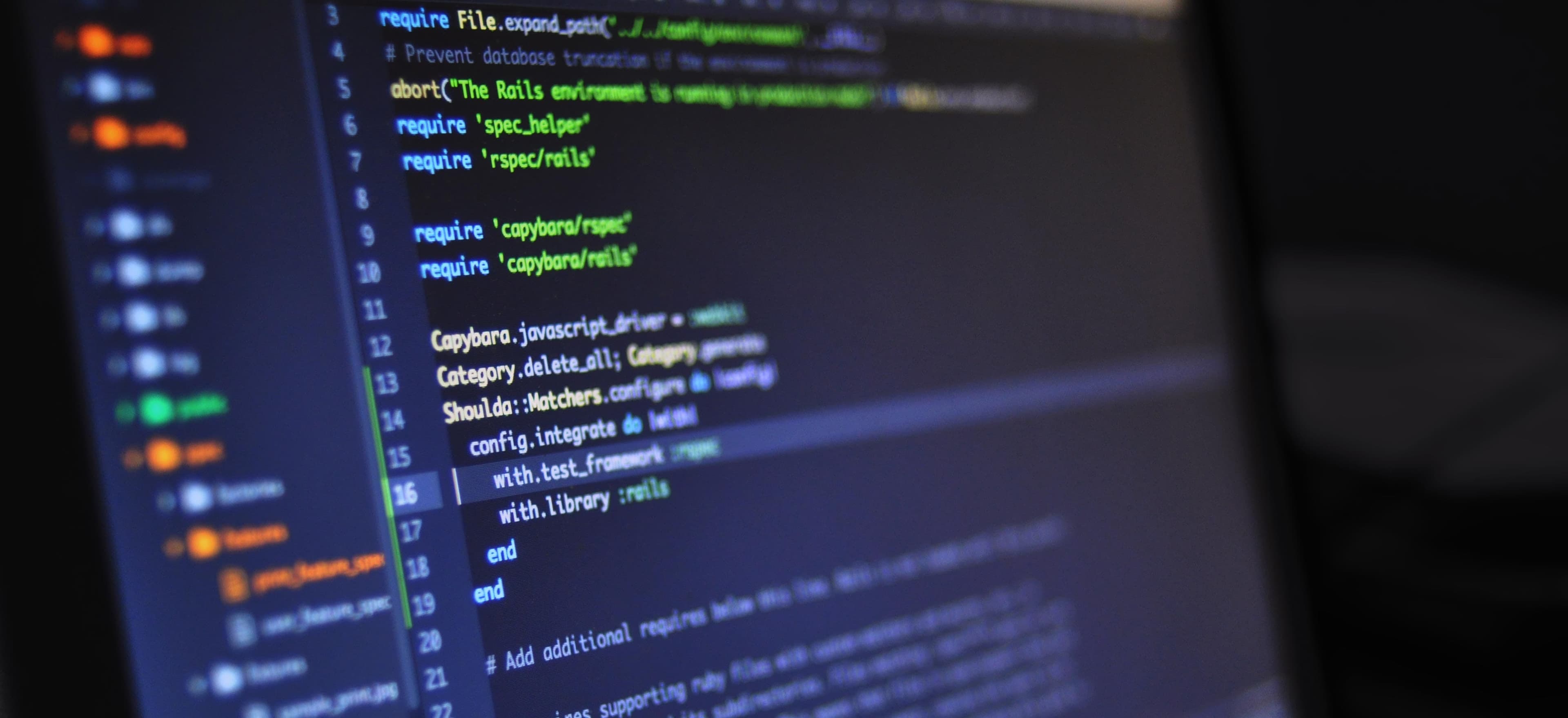
- Published on
Choosing Between Data-Driven and Logic-Based Algorithms
In the age of big data, algorithms serve as the backbone of countless applications. With options that span from data-driven techniques to logic-based reasoning, making the right choice can significantly impact the outcome of your project. This blog post will provide insights into these two distinct approaches, their advantages, disadvantages, and when to use each.
Understanding Data-Driven Algorithms
Data-driven algorithms rely primarily on data to learn patterns and make decisions. This approach is particularly useful in scenarios where one can leverage vast amounts of data without having explicit rules governing the outcome. They are often used in machine learning, where the algorithm adapts based on the input data.
How Data-Driven Algorithms Work
Consider a scenario where we are trying to predict housing prices based on various factors like location, size, and amenities. A data-driven algorithm might use historical price data to train itself on these features.
import java.util.List;
public class HousingPricePredictor {
// A simple class to represent a house with its features
class House {
double size;
int numBedrooms;
String location;
// Constructor
public House(double size, int numBedrooms, String location) {
this.size = size;
this.numBedrooms = numBedrooms;
this.location = location;
}
}
public double predictPrice(List<House> houses) {
// Placeholder logic for price prediction
double predictedPrice = 0;
for (House house : houses) {
predictedPrice += (house.size * 200) + (house.numBedrooms * 5000); // Simplistic formula
// More complex models can be substituted here, like regression
}
return predictedPrice / houses.size();
}
}
In this code snippet, we have created a class to predict housing prices based on size and the number of bedrooms. The predictPrice method is simplistic but serves as a placeholder for more complex algorithms, like regression or neural networks. The main takeaway here is that a data-driven approach leverages historical data to derive insights and inform predictions.
Advantages of Data-Driven Algorithms
- Flexibility: Adapt to new data without requiring a complete overhaul.
- Scalability: Handle large datasets effectively through training and optimization.
- Accuracy: Often achieve higher accuracy in prediction when trained properly.
Disadvantages of Data-Driven Algorithms
- Data Dependency: Performance is highly dependent on the quality and quantity of data.
- Overfitting: Risk of creating models that are too tailored to the training data, resulting in poor generalization.
- Black Box Nature: Often, the reasoning behind predictions can be opaque and hard to interpret.
Understanding Logic-Based Algorithms
Logic-based algorithms, on the other hand, rely on predefined rules and logical conditions to make decisions. They are built on the principles of formal logic and are widely used in rule-based systems, expert systems, and artificial intelligence applications.
Example: An Expert System
Suppose we are creating an expert system for diagnosing illnesses based on symptoms. Here, rules govern the decision-making process.
public class HealthDiagnosisSystem {
public String diagnose(String symptom) {
if ("fever".equals(symptom)) {
return "Possible flu or infection.";
} else if ("cough".equals(symptom)) {
return "Consider checking for respiratory issues.";
} else if ("headache".equals(symptom)) {
return "Could be tension headache or migraines.";
} else {
return "Symptom not recognized. Please consult a professional.";
}
}
}
In this code, the diagnose
method provides feedback based on the symptom input by the user. Logic-based algorithms focus on establishing clear rules that encapsulate domain knowledge.
Advantages of Logic-Based Algorithms
- Transparency: Rules can be easily understood and modified.
- No Need for Data: Can operate effectively even in situations with limited data.
- Reliability: Consistent and reproducible outputs based on the defined rules.
Disadvantages of Logic-Based Algorithms
- Rigid: Struggles to adapt to new situations not covered by predefined rules.
- Domain Limitations: Only as good as the rules specified; might miss critical insights outside its scope.
- Complexity: As the number of rules increases, managing them can become unwieldy.
When to Choose Which Approach
The choice between data-driven and logic-based algorithms depends greatly on the specific needs of your application.
Use Data-Driven Algorithms When:
- You have access to large datasets.
- You expect the data or problem domain to evolve.
- Improved accuracy is critical, and you can invest effort in tuning the model.
Use Logic-Based Algorithms When:
- You have limited or no data.
- You need transparency and explainability in decision-making.
- The problem is well-defined with established rules and constraints.
Key Takeaways
Choosing between data-driven and logic-based algorithms is not a straightforward task; each has its unique strengths and weaknesses. As a rule of thumb, assess your project’s requirements, the availability of data, and the importance of transparency in the decision-making process.
By adhering to fundamental principles and frameworks, you can select the most suitable algorithmic approach to drive the success of your project. As the technology landscape continues to evolve, remaining aware of these methodologies will empower you to make informed decisions that can advance your objectives.
For more on algorithm types and their implications, check out articles on machine learning and expert systems.
The landscape of algorithms is not static; it adapts with advancements in AI, machine learning, and data processing techniques. Whether it's data-driven or logic-based, understanding each approach's foundational elements is key to unlocking their potential. Remember to continuously evaluate and iterate upon your algorithms for optimal performance.
Happy coding!