Common Pitfalls in Java Agents for Beginners
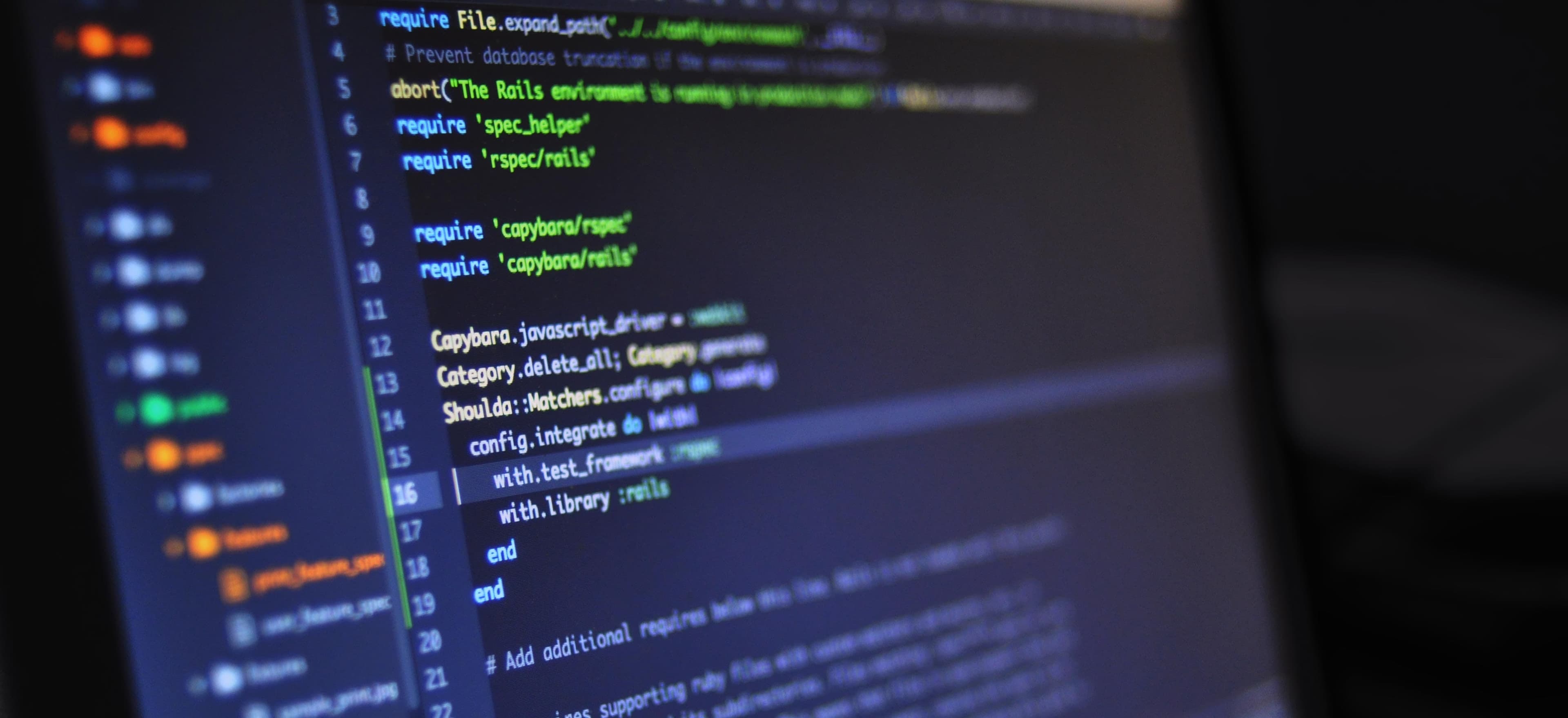
- Published on
Common Pitfalls in Java Agents for Beginners
Java agents provide a powerful mechanism for bytecode manipulation, allowing developers to enhance their applications with capabilities like monitoring, profiling, and instrumentation. However, despite their utility, beginners often encounter several common pitfalls. In this blog post, we will explore these pitfalls, providing clear explanations, examples, and recommended practices to help you effectively navigate the world of Java agents.
What is a Java Agent?
Before diving into pitfalls, it’s essential to understand what a Java agent is. A Java agent is a special type of JAR file that can modify the behavior of a Java application at runtime. While you can apply Java agents in various contexts, they are commonly used for monitoring runtime performance, adding metrics, or implementing security features.
Java agents employ the java.lang.instrument
package, which allows developers to define premain
and agentmain
methods for actions to perform when the agent is loaded.
import java.lang.instrument.Instrumentation;
public class MyJavaAgent {
public static void premain(String agentArgs, Instrumentation inst) {
// Code to be executed before the main application starts
}
public static void agentmain(String agentArgs, Instrumentation inst) {
// Code to be executed when the agent is loaded into a running JVM
}
}
Pitfall 1: Ignoring Class Loading Implications
A common pitfall encountered by beginners is failing to consider how class loading works in Java. Java agents can modify classes at various points in the lifecycle of a Java application. If you attempt to redefine a class that hasn't been loaded yet, you’ll encounter problems.
Best Practice: Always ensure that the class you want to redefine has been loaded. You can track this by using Instrumentation.getAllLoadedClasses()
and checking if the class you want to redefine is present.
Class<?>[] loadedClasses = inst.getAllLoadedClasses();
for (Class<?> loadedClass : loadedClasses) {
if (loadedClass.getName().equals("com.example.MyClass")) {
// Safe to redefine the class
}
}
Pitfall 2: Overusing Bytecode Manipulation
While bytecode manipulation is powerful, overusing it can lead to hard-to-track errors and performance issues. Think carefully about whether you need to instrument a class extensively.
Best Practice: Only apply changes where necessary. Use tools like AspectJ for cross-cutting concerns instead of modifying bytecode directly unless you have specific reasons.
Pitfall 3: Forgetting to Handle Exceptions
Failures in instrumentation can throw exceptions; however, beginners often overlook this by not implementing try-catch blocks. Failing to handle exceptions can lead to the entire application crashing unexpectedly.
Example: Catching Runtime Exceptions
try {
inst.redefineClasses(...);
} catch (ClassNotFoundException | UnmodifiableClassException e) {
// Handle the exception gracefully
System.err.println("Failed to redefine class: " + e.getMessage());
}
Best Practice: Always handle checked exceptions in your instrumentation code to maintain application stability.
Pitfall 4: Misunderstanding the Java Security Model
Java has a robust security model that restricts how classes can interact with one another. When developing agents, security policies can sometimes frustrate beginners.
For instance, attempting to access private fields or methods without the appropriate permission can lead to runtime exceptions.
Best Practice: Familiarize yourself with the Java Security Manager. If necessary, run your JVM with appropriate permissions to allow your agent to function as intended.
java -javaagent:myagent.jar -Djava.security.policy=my.policy
Pitfall 5: Unwinding at the Correct Context
Often, beginners don’t realize that you need to maintain the correct context when working with Java agents. In particular, when redefining classes or modifying object states, context is key.
Best Practice: Always keep track of which contexts (such as thread states or class loaders) will affect your instrumentation. Documenting these interactions will also help in debugging later.
Pitfall 6: Not Testing in a Controlled Environment
Testing your Java agent in the same environment as your production code can be tempting, but it’s a significant risk. Agents can alter application behavior in unpredictable ways.
Best Practice: Always test your agents in isolated environments. Consider using testing frameworks such as JUnit for integration tests and confirm that your changes are working as expected without impacting existing functionality.
Pitfall 7: Forgetting the JVM Version Compatibility
Java agents may behave differently based on the JVM version. Beginners may create agents that work seamlessly in one version but fail to function in another.
Best Practice: Always specify the JVM version compatibility in your project documentation, and run your tests across different environments if possible. Verify any features against the Java SE Documentation.
My Closing Thoughts on the Matter
Java agents can significantly enhance your applications' performance, monitoring, and security features. However, as we have explored, they come with several common pitfalls, especially for beginners. By understanding these pitfalls and adhering to best practices, you can avoid potential issues as you create more robust and efficient Java applications.
Additional Resources
For a deeper dive into Java agents and bytecode manipulation, consider checking the following resources:
- Java Instrumentation Guide
- AspectJ Documentation
Final Note
Java agents will continue to play an essential role in the Java ecosystem, especially with the growing trend toward monitoring and instrumentation. By equipping yourself with knowledge about common pitfalls and best practices, you will surely become a more effective developer. Happy coding!