Optimizing Performance: Removing Recursion in ConcurrentHashMap
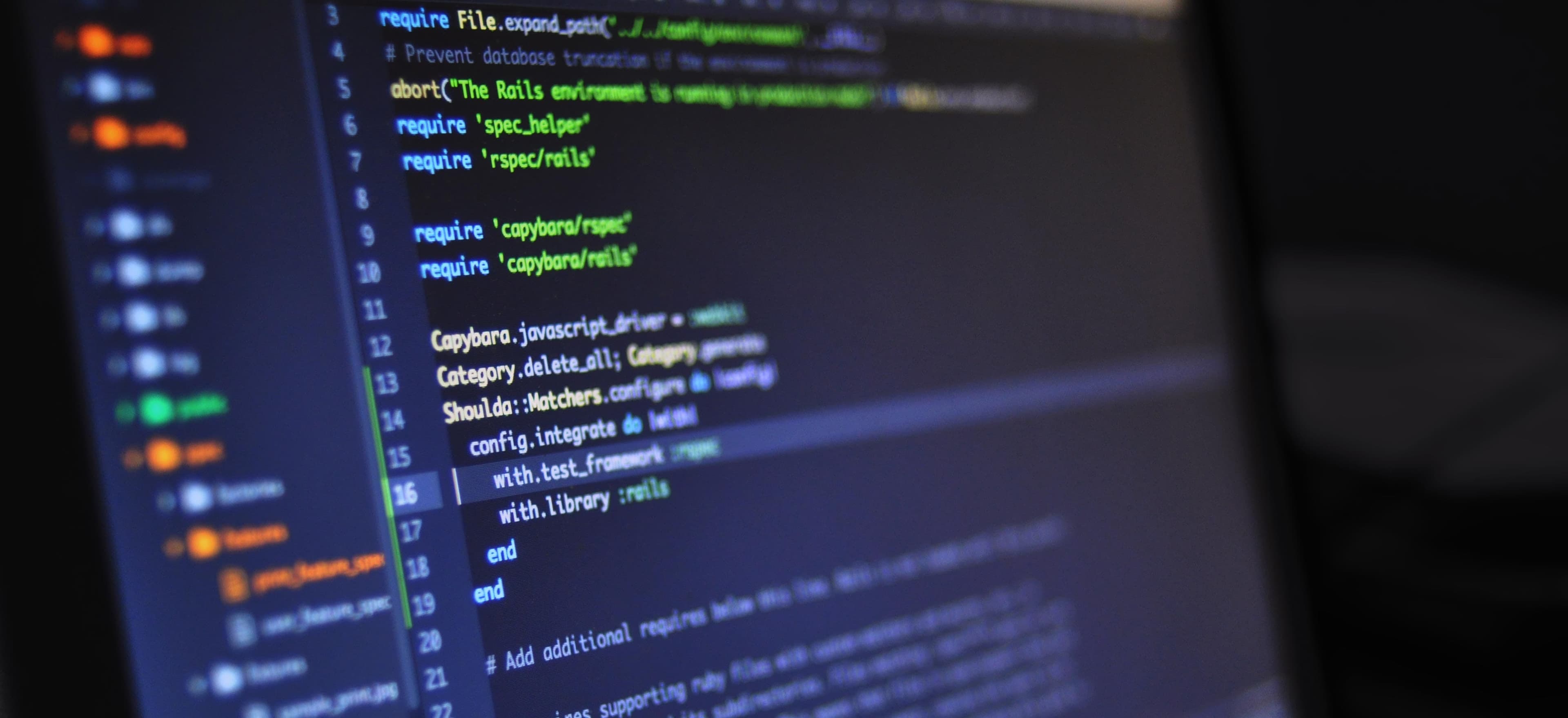
- Published on
Optimizing Performance: Removing Recursion in ConcurrentHashMap
ConcurrentHashMap is a powerful data structure in Java used for managing concurrent access to a map. It allows for high concurrency while maintaining the integrity of the data. However, in certain scenarios, the use of recursion can impact the performance of ConcurrentHashMap. In this post, we will explore the impact of recursion and how to optimize performance by removing recursion in ConcurrentHashMap operations.
Understanding Recursion in ConcurrentHashMap
Recursion in ConcurrentHashMap occurs when an operation, such as get
, put
, or remove
, invokes itself to handle collisions or map resizing. While recursion is a powerful concept, it can lead to performance issues in highly concurrent environments. This is particularly true when dealing with a large number of elements and high contention for resources.
Recursion in ConcurrentHashMap can lead to excessive thread stack usage and increased contention, ultimately affecting the overall performance of the application.
Impact of Recursion on Performance
The impact of recursion on ConcurrentHashMap operations becomes apparent when the number of elements in the map grows and the level of concurrency increases. Excessive recursion can lead to:
- Increased memory consumption due to stack frames
- Higher contention for resources
- Degraded performance under heavy load
When faced with these performance issues, it becomes imperative to optimize ConcurrentHashMap to reduce or eliminate recursion in its operations.
Optimizing ConcurrentHashMap Performance
The removal of recursion in ConcurrentHashMap can be achieved through various techniques such as using iteration instead of recursion for handling collisions and resizing. Let's explore some effective strategies for optimizing performance by eliminating recursion in ConcurrentHashMap operations.
Strategy 1: Iterative Collision Handling
One of the key areas where recursion can impact performance in ConcurrentHashMap is during collision resolution. By default, ConcurrentHashMap uses a linked list to handle collisions. When a collision occurs, the entry is added to the linked list. However, if the linked list becomes too long, it gets transformed into a binary tree for better performance. This transformation can involve recursion.
To optimize performance in this scenario, we can use an iterative approach instead of recursion for handling collisions. This can be achieved by iteratively traversing the linked list or the binary tree to find the appropriate entry.
// Iterative collision handling
Node<K,V> findNode(Object key, int hash) {
for (Node<K,V> e = tab[indexFor(hash, table.length)];
e != null;
e = e.next) {
// Perform operations
}
return null;
}
By using an iterative approach, we avoid the overhead of recursive method calls, leading to improved performance and reduced contention.
Strategy 2: Non-Recursive Resizing
ConcurrentHashMap dynamically resizes its internal data structure to accommodate the addition of elements. However, the resizing process can involve recursion, especially when transferring entries from the old table to the new table.
To optimize performance, we can implement a non-recursive approach for resizing the ConcurrentHashMap. This involves iteratively transferring the entries from the old table to the new table without the use of recursion.
// Non-recursive resizing
void resize() {
if (transferIndex >= 0) {
// Perform resizing operations
}
}
By utilizing a non-recursive approach for resizing, we eliminate the overhead of recursive calls, resulting in improved performance and reduced resource contention during resizing operations.
Strategy 3: Performance Testing and Profiling
Optimizing the performance of ConcurrentHashMap by removing recursion requires thorough testing and profiling to measure the impact of the applied optimizations. It is essential to design comprehensive performance tests to evaluate the effectiveness of the optimization strategies.
Profiling tools such as Java Mission Control, JProfiler, or YourKit can be utilized to analyze the performance characteristics and identify hotspots where recursion impacts ConcurrentHashMap operations.
By thoroughly testing and profiling the optimized ConcurrentHashMap, we can ensure that the performance improvements are substantial and validate the effectiveness of the applied strategies.
Key Takeaways
In conclusion, the removal of recursion in ConcurrentHashMap operations is crucial for optimizing performance in highly concurrent environments. By utilizing iterative collision handling, non-recursive resizing, and comprehensive performance testing, we can effectively mitigate the impact of recursion and enhance the overall performance of ConcurrentHashMap.
For highly concurrent applications where the performance of ConcurrentHashMap is critical, optimizing its operations by removing recursion becomes a vital aspect of application design and development.
With a clear understanding of the impact of recursion on ConcurrentHashMap and effective optimization strategies at hand, developers can create high-performance, concurrent applications leveraging the power of ConcurrentHashMap without being encumbered by the limitations imposed by recursion.
By adopting these optimization strategies, developers can ensure that ConcurrentHashMap continues to be a reliable and performant concurrent data structure in Java.
So, when working with ConcurrentHashMap in your Java applications, always remember the significance of optimizing performance by removing recursion to attain the highest levels of concurrency and efficiency.
Let's ensure that the performance of ConcurrentHashMap remains at its peak, even in the most demanding concurrent environments. Happy optimizing!