Speed Up Maven: Tackling Slow Compile and Packaging Times
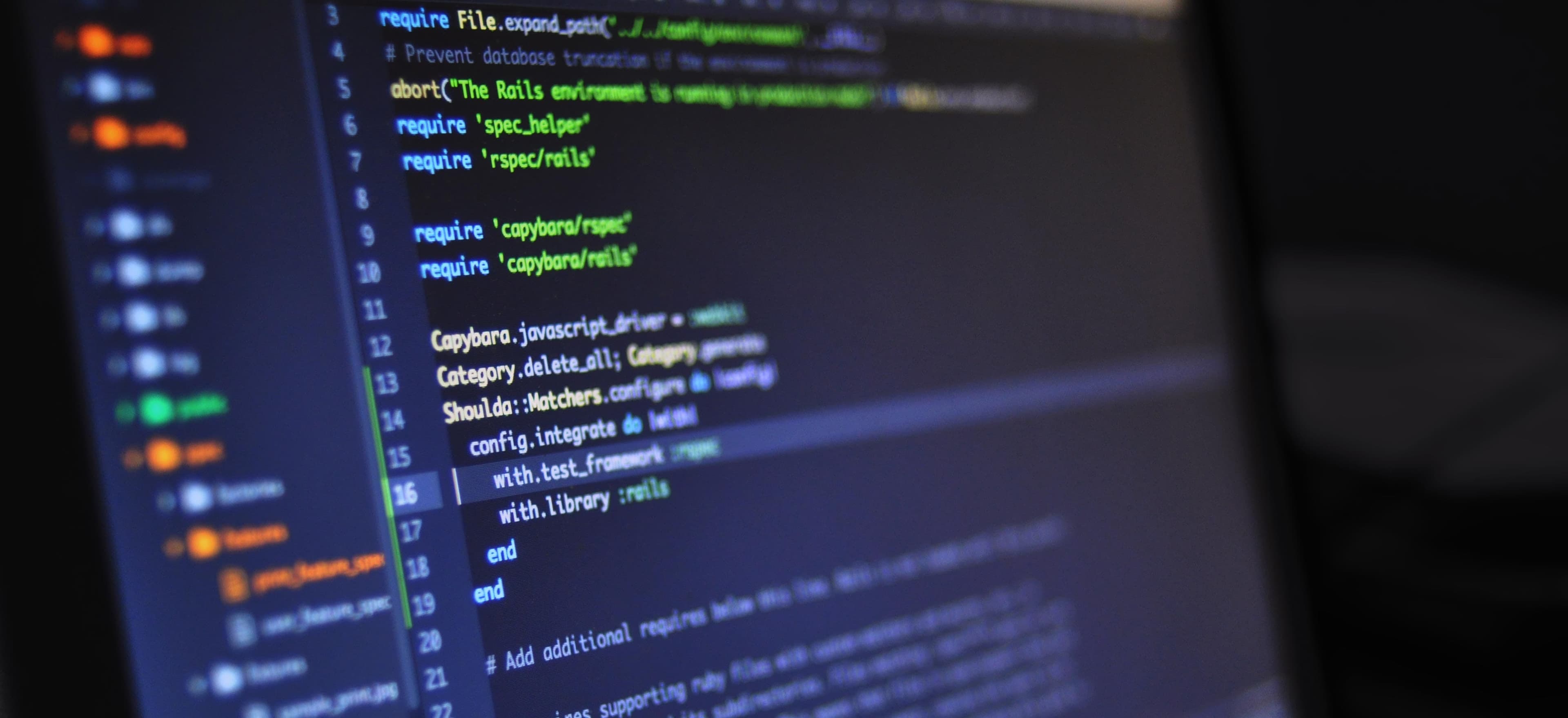
- Published on
Speed Up Maven: Tackling Slow Compile and Packaging Times
Maven is a powerful build automation tool used primarily for Java projects. However, developers frequently encounter the frustrating issue of slow compile and packaging times. In this blog post, we'll discuss effective strategies to optimize Maven's performance, enhance your development workflow, and ultimately make your builds faster.
Understanding Maven Build Lifecycle
Before diving into optimization techniques, it’s essential to understand Maven’s build lifecycle. Maven follows a sequence of phases and goals that dictate how your project is built. The three primary phases are:
- Validate: Check if the project is correct and all necessary information is available.
- Compile: Compile the source code of the project.
- Package: Assemble the compiled code into distributable formats like JAR or WAR.
By knowing these phases, you can identify which areas of the build process can be optimized.
Common Culprits for Slow Builds
- Large Project Size: Projects with numerous dependencies and modules can take longer to compile and package.
- Frequent Dependency Updates: Constantly downloading dependencies can significantly slow down a build.
- Insufficient Hardware: Memory and CPU limitations can bottleneck the compiling process.
- Inefficient Configuration: Improper pom.xml configurations can lead to longer build times.
Strategies for Speeding Up Maven
1. Use a Local Repository Cache
Maven downloads dependencies every time you build a project anew unless they are in the local repository. To ensure that your local repository is fully utilized:
<settings>
<localRepository>/path/to/local/repo</localRepository>
</settings>
This tells Maven where to look for dependencies locally before downloading from the remote repository.
2. Reduce Plugin Overhead
Plugins add functionality to Maven, but they can also introduce overhead. Analyze your pom.xml file to determine if you really need all the plugins currently being used.
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
<!-- Remove unnecessary plugins or update versions -->
</plugins>
</build>
Only keep essential plugins to keep your build speed optimal.
3. Parallel Builds
Maven supports parallel builds, which can significantly speed up your compile and package times. To enable this:
mvn -T 1C clean install
This command instructs Maven to use one thread per available CPU core, thus speeding up the build.
Why this works: Parallel builds utilize your system’s processing power more efficiently, especially in projects with multiple modules that can be built simultaneously.
4. Dependency Management
Analyzing and managing dependencies is crucial in optimizing builds. Use the dependency:analyze
goal:
mvn dependency:analyze
This command analyzes your project to find unused dependencies and dependencies that could be added for better performance. Reducing unnecessary dependencies can lead to much faster builds.
5. Incremental Builds
Maven can perform incremental builds by detecting changes in your source files and compiling only what has changed. To utilize this feature effectively, run:
mvn clean
This prepares the project for a fresh build. Although this may not seem like an optimization, it prevents Maven from always recompiling the entire codebase, thus speeding things up when only small changes are made.
6. Use of Profiles
Maven supports build profiles, allowing you to have different configurations for different scenarios. For instance, you might want one profile for development that skips tests:
<profiles>
<profile>
<id>dev</id>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<skipTests>true</skipTests>
</configuration>
</plugin>
</plugins>
</build>
</profile>
</profiles>
Why profiles are beneficial: This allows you to skip resource-intensive processes and, thus, shorten build time when you are actively developing.
7. Optimize JVM Configuration
Maven runs on the Java Virtual Machine (JVM), and tuning the JVM can enhance build performance. Set the following in your .mvn/maven.config
file:
-Xmx2048m
-XX:MaxPermSize=512m
This configures Maven to use more memory, reducing the likelihood of garbage collection stalling the build process.
8. Use Maven Wrapper
The Maven Wrapper (mvnw
) allows you to ensure consistent Maven environments across different systems. It can save time on configuring a Maven installation for new developers or CI systems by providing automatic setup.
To set up the Maven Wrapper, use the following command:
mvn -N io.takari:maven:wrapper
This helps to keep builds consistent, avoiding slowdowns due to version mismatches across varying environments.
9. CI/CD Pipeline Optimization
If your project is part of a CI/CD pipeline, take the time to optimize the pipeline itself. You can cache dependencies, only run tests that affect modified code, and isolate build environments. Tools like Jenkins or GitHub Actions can significantly improve the efficiency of your builds when configured correctly.
10. Consider Maven Alternatives
Lastly, if slow builds remain a thorn in your side, consider alternatives like Gradle. Gradle offers incremental builds natively and uses a different execution model that may better suit your needs. You can check out Gradle's official documentation for more information.
Key Takeaways
Sluggish compile and packaging times can frustrate even the most seasoned developers. By employing the strategies outlined above, you can significantly enhance your Maven experience. From managing dependencies to leveraging parallel builds, each tip contributes to a smoother, more efficient workflow.
Remember to continuously evaluate your build process and refine where necessary. Optimization is an ongoing journey. Start implementing these strategies today to see the difference in your build times—your future self will thank you!
For additional tips on Java build performance, you can read about Maven Builds Best Practices to stay updated with the latest methods.
Happy coding!