Uncovering Java's Hidden Features: What Most Developers Miss
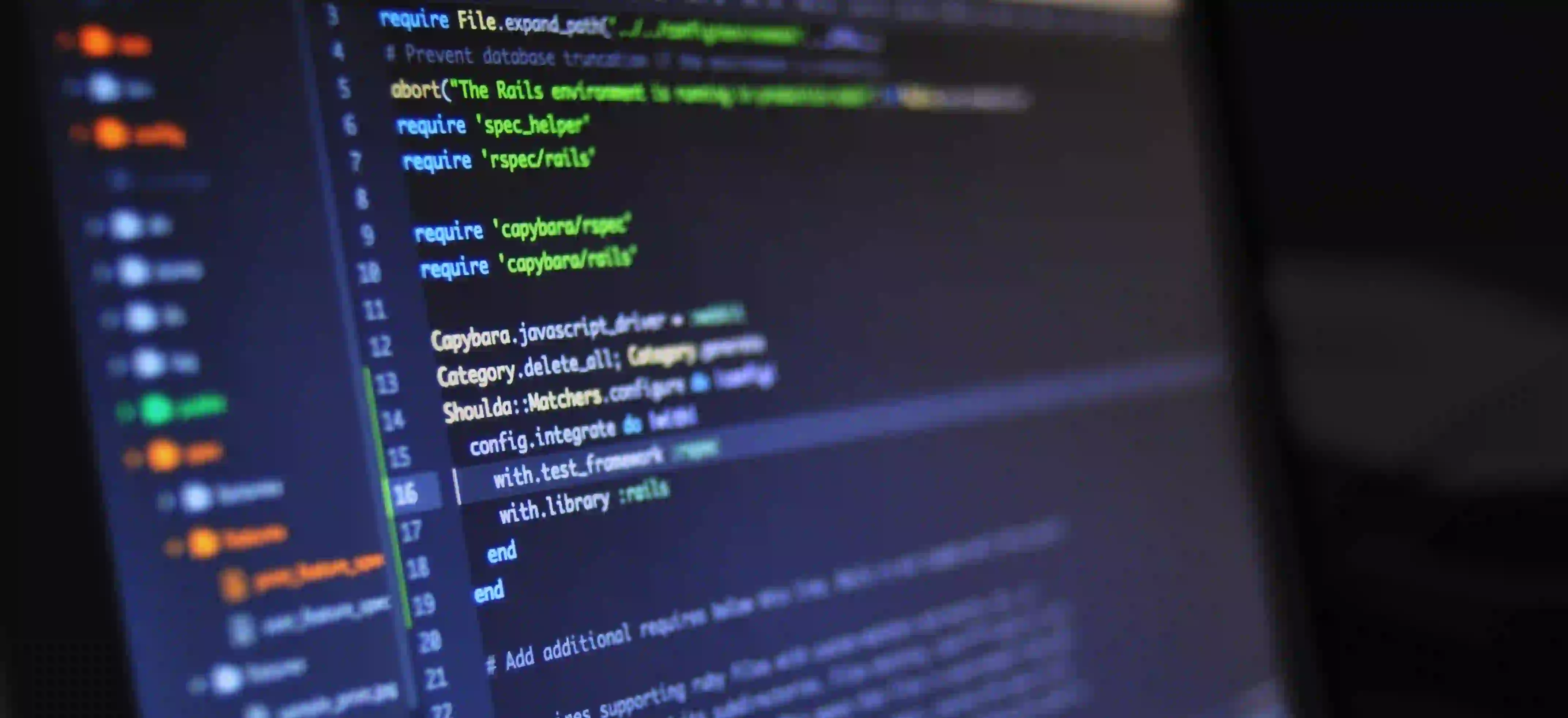
Uncovering Java's Hidden Features: What Most Developers Miss
Java is an omnipresent programming language, revered for its portability, robustness, and maturing ecosystem. Many developers have mastered its basic features, but the language is vast—packed with hidden gems that can significantly enhance productivity and code quality. In this blog post, we will explore some of Java’s lesser-known features and functionalities that developers often overlook.
1. Features of the var
Declaration
Since the introduction of Java 10, Java has permitted local variable type inference using var
. This feature allows you to declare a variable without explicitly stating its type.
Example
var number = 10; // Number is inferred as Integer
var message = "Hello, Java!"; // Message is inferred as String
Why Use var
?
- Simplification: It reduces verbosity.
- Clarity: When combined with meaningful variable names,
var
can make the code less cluttered.
However, use it judiciously. Overusing var
can make the code less readable when types are not obvious at a glance.
2. The switch
Expression
Introduced in Java 12, the switch
expression enhances the traditional switch
statement to return values and allows multiple labels on a single line.
Example
var day = 3;
String dayType = switch (day) {
case 1, 2, 3, 4, 5 -> "Weekday";
case 6, 7 -> "Weekend";
default -> throw new IllegalArgumentException("Invalid day: " + day);
};
System.out.println(dayType); // Weekday
Why Use This Feature?
- Conciseness: The
switch
expression reduces the boilerplate often found in traditionalswitch
statements. - Flexibility: It allows returning values directly and supports the use of lambda-like syntax.
3. Text Blocks
Text blocks, introduced in Java 13, facilitate multi-line string literals, making it easier to handle HTML, JSON, or SQL strings.
Example
String html = """
<html>
<body>
<h1>Hello, Java!</h1>
</body>
</html>
""";
System.out.println(html);
Why Use Text Blocks?
- Readability: They improve the readability and maintainability of code that includes multi-line strings.
- Formatting: Indentation is preserved, making it easier to visualize the structure.
4. Streams for Data Manipulation
Java 8 introduced the Stream API, an immensely powerful feature for processing collections of data in a functional style. Although many know its basic use for filtering and mapping, its full potential often remains untapped.
Example
List<String> names = Arrays.asList("John", "Jane", "Jake", "Jill");
List<String> result = names.stream()
.filter(name -> name.startsWith("J"))
.map(String::toUpperCase)
.collect(Collectors.toList());
System.out.println(result); // [JOHN, JANE, JAKE, JILL]
Why Use Streams?
- Chaining Operations: Multiple operations can be chained together in a concise manner.
- Parallel Processing: Streams can easily be converted to support parallel execution, enhancing performance on large datasets.
5. Optional for Null Safety
The Optional
class, introduced in Java 8, provides a way to express that a variable may or may not hold a value, thus reducing the risk of NullPointerExceptions
.
Example
Optional<String> name = Optional.of("John");
name.ifPresent(n -> System.out.println("Hello, " + n)); // Hello, John
String result = name.orElse("Guest");
System.out.println(result); // John
Why Use Optional?
- Intent Expressiveness: Using
Optional
conveys that the presence of a value is uncertain. - Method Chaining: It encourages functional programming practices through method chaining.
6. Default Methods in Interfaces
Default methods allow you to add new methods to interfaces without breaking existing implementations. This is particularly handy when evolving interfaces.
Example
interface Animal {
void makeSound();
default void sleep() {
System.out.println("Sleeping...");
}
}
class Dog implements Animal {
public void makeSound() {
System.out.println("Bark");
}
}
public static void main(String[] args) {
Animal dog = new Dog();
dog.makeSound(); // Bark
dog.sleep(); // Sleeping...
}
Why Use Default Methods?
- Backward Compatibility: They enable you to evolve interfaces while maintaining existing implementations.
- Code Reusability: Common functionality can be defined once and reused across various implementations.
7. The @FunctionalInterface
Annotation
This annotation marks an interface as a functional interface—an interface with only one abstract method. It enforces clarity and correctness.
Example
@FunctionalInterface
interface Greeting {
void sayHello(String name);
}
public static void main(String[] args) {
Greeting greet = name -> System.out.println("Hello, " + name);
greet.sayHello("Java"); // Hello, Java
}
Why Use @FunctionalInterface?
- Design Clarity: Enforces the definition of functional interfaces, making the intention clearer.
- Compiler Enforcement: Prevents the addition of multiple abstract methods, ensuring the interface remains functional.
8. Local-Variable Syntax for Lambda Parameters
Java 11 introduced the concept of using var
in lambda expressions, which allows local variable type inference for lambda parameters.
Example
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach((var name) -> System.out.println(name));
Why Use Local-Variable Syntax in Lambdas?
- Clarity: This improves readability, particularly when dealing with generics.
- Consistency: Aligns with the broader use of
var
throughout Java.
The Closing Argument
Java's evolution has introduced several features and techniques that many developers have yet to fully harness. By integrating these hidden gems into your coding repertoire, you can streamline your development process, enhance code quality, and prepare your applications for future scalability.
Further Reading
- Java SE Documentation
- Understanding Streams in Java
- Functional Programming in Java
By continually exploring Java’s offerings, you can become a more proficient and confident developer, setting yourself apart in this ever-evolving landscape. Happy coding!