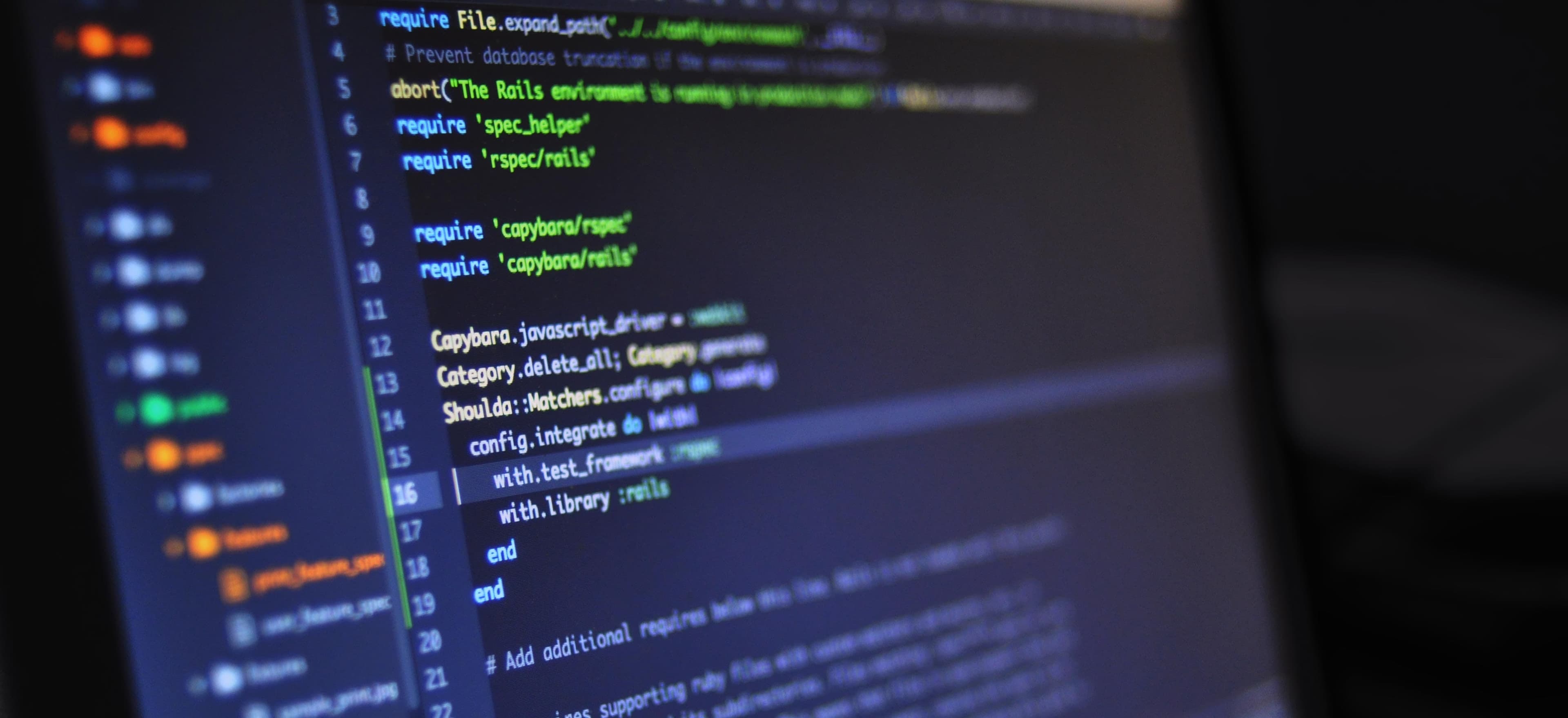
- Published on
Securely Invoking APIs in a Web App with OAuth2 and JWT
In the world of web development, integrating with third-party APIs is a common requirement. When it comes to securely invoking APIs in a web app, OAuth2 and JSON Web Tokens (JWT) are two prominent technologies that come to the forefront. In this blog post, we will explore the fundamentals of OAuth2 and JWT, and then delve into how to securely invoke APIs in a web app using these technologies.
Understanding OAuth2
OAuth2 is an authorization framework that enables a web app to access a protected resource on behalf of a user. It provides a secure and standardized way for web apps to obtain limited access to a user's data without exposing their credentials. OAuth2 involves multiple parties: the client (the web app), the resource server (the API), and the authorization server.
OAuth2 Flow
The OAuth2 flow typically involves the following steps:
- The client initiates the flow by redirecting the user to the authorization server.
- The user authenticates and grants permissions to the client.
- The authorization server issues an access token to the client.
- The client uses the access token to access the protected resources on the resource server.
JSON Web Tokens (JWT)
JSON Web Tokens (JWT) are compact, URL-safe, and self-contained tokens that can be used to transmit information between two parties. JWTs are commonly used for securely transmitting information between the client and the server. A JWT consists of three parts: the header, the payload, and the signature.
JWT Structure
A JWT has the following structure: xxxxx.yyyyy.zzzzz
- The header typically contains the type of the token and the signing algorithm being used.
- The payload contains the claims, which are statements about an entity and additional metadata.
- The signature is used to verify that the sender of the JWT is who it says it is and to ensure that the message wasn't changed along the way.
Securely Invoking APIs with OAuth2 and JWT
Now that we have an understanding of OAuth2 and JWT, let's explore how to securely invoke APIs in a web app using these technologies. We'll go through a step-by-step guide to integrate OAuth2 and JWT into a Java web app.
Step 1: Obtain OAuth2 Credentials
Before integrating OAuth2 into the web app, you'll need to obtain the OAuth2 credentials from the provider of the API you want to access. These credentials typically include a client ID, a client secret, and the authorization endpoint URL.
Step 2: Implement OAuth2 Authentication Flow
In your Java web app, you'll need to implement the OAuth2 authentication flow. This involves redirecting the user to the authorization server's login page, handling the callback after the user grants permissions, and exchanging the authorization code for an access token.
// Example implementation of OAuth2 authentication flow in Java
public class OAuth2Client {
private String clientId;
private String clientSecret;
private String authorizationEndpoint;
private String tokenEndpoint;
public OAuth2Client(String clientId, String clientSecret, String authorizationEndpoint, String tokenEndpoint) {
this.clientId = clientId;
this.clientSecret = clientSecret;
this.authorizationEndpoint = authorizationEndpoint;
this.tokenEndpoint = tokenEndpoint;
}
// Method to initiate the OAuth2 flow by redirecting the user to the authorization server
public String initiateAuthentication() {
// Construct the authorization URL and redirect the user
String authorizationUrl = authorizationEndpoint + "?client_id=" + clientId + "&redirect_uri=" + redirectUri + "&response_type=code";
return authorizationUrl;
}
// Method to exchange the authorization code for an access token
public String exchangeCodeForToken(String code) {
// Make a POST request to the token endpoint with the authorization code and client credentials
// Retrieve and return the access token from the response
}
}
Step 3: Securely Transmitting JWTs
Once you obtain the access token from the authorization server, you can use it to securely transmit JWTs to the resource server. The JWT should be included as a bearer token in the Authorization
header of the HTTP request.
// Example of securely transmitting JWTs in Java
public class APIClient {
private String apiUrl;
public APIClient(String apiUrl) {
this.apiUrl = apiUrl;
}
public String invokeAPI(String accessToken) {
// Make a GET request to the API with the access token in the Authorization header
// Process the response from the API
}
}
By securely transmitting JWTs with the access token included as a bearer token, you can securely invoke the APIs in your Java web app.
Final Thoughts
In conclusion, OAuth2 and JWT play crucial roles in securely invoking APIs in a web app. By understanding the OAuth2 flow and the structure of JWTs, and by implementing OAuth2 authentication flow and securely transmitting JWTs in your Java web app, you can ensure the security and integrity of API invocations.
Integrating OAuth2 and JWT into a Java web app empowers you to seamlessly and securely interact with third-party APIs, enhancing the functionality and value of your web app.
Now that you have a deeper understanding of securely invoking APIs with OAuth2 and JWT in a Java web app, it's time to put this knowledge into practice and elevate the security of your API integrations.
Remember, security is paramount in the world of web development, and leveraging technologies like OAuth2 and JWT is a significant step towards ensuring the protection of user data and the integrity of API communications.
For more in-depth insights into OAuth2 and JWT, check out the OAuth 2.0 Authorization Framework and the JWT.io websites.
Happy coding!
Checkout our other articles