Boosting Scala Tuple Performance: Key Strategies Revealed
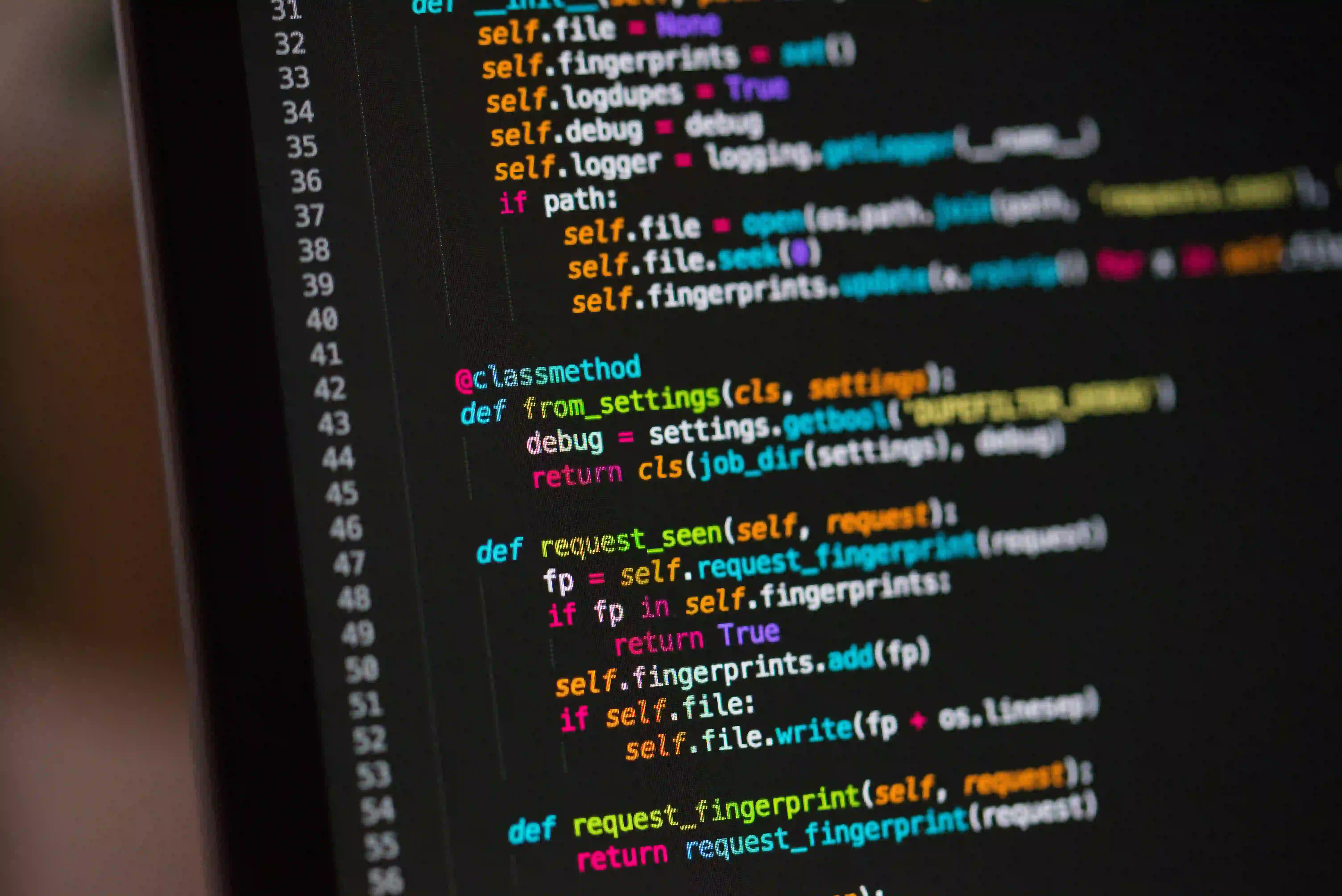
Boosting Scala Tuple Performance: Key Strategies Revealed
Scala is a powerful programming language that combines object-oriented and functional programming paradigms. One of its lightweight features is the use of Tuples, which allows developers to group multiple values together without creating an explicit class. While Tuples can simplify code and enhance readability, performance considerations can arise in large-scale applications. This blog post aims to explore the performance of Scala Tuples and offer strategies to help you boost their efficiency.
Understanding Scala Tuples
Before diving into performance strategies, it's important to understand what Tuples are and when to use them. A Tuple in Scala is a finite ordered list of elements, which can consist of any type and number of elements. For instance:
val tupleExample = (1, "Scala", 3.14)
In this example, the Tuple contains an integer, a string, and a double.
Why Use Tuples?
Tuples provide a convenient way to group data. Use them when you want to return multiple values from a function or when dealing with small collections of heterogeneous types. They help in reducing boilerplate code and enhance clarity.
However, while Tuples are handy, they can impact performance if not used wisely, especially in cases with a high frequency of creation and destruction, or when manipulating large datasets.
Performance Bottlenecks with Tuples
-
Immutable Nature: Tuples in Scala are immutable. Every time you create a new Tuple, a new instance is generated. This can lead to performance degradation if you're frequently modifying data.
-
Boxing and Unboxing: When tuples contain primitive types, they need to be boxed into reference types, which results in overhead, particularly in high-performance scenarios.
-
Pattern Matching: While Scala’s powerful pattern matching capabilities work seamlessly with Tuples, using it extensively can impact performance due to additional overhead.
Strategies to Boost Tuple Performance
Here are some strategies that you can implement in your Scala applications to enhance the performance of Tuples.
1. Use Case Classes Instead of Tuples
When dealing with complex data structures that require more than just storage, consider using case classes instead of Tuples. Case classes give you named parameters and can be more efficient in terms of garbage collection, especially in large collections.
case class Person(name: String, age: Int)
val person = Person("Alice", 30)
Using case classes not only clarifies the purpose of each value but also provides better performance when used in collections, particularly when the objects need to be processed multiple times.
2. Limit Tuple Size
Keep Tuples small. Scala allows you to create Tuples with up to 22 elements, but using Tuples of large sizes can lead to performance issues. If you find yourself needing larger Tuples, re-evaluate your data structure.
3. Use Efficient Collection Types
Instead of frequently creating new Tuples, consider using collections like Lists, Maps, or Sets, which are optimized for various operations. These collections can often offer better performance by reducing the overhead involved in creation and destruction:
val numbersList = List(1, 2, 3)
val numbersTuple = (1, 2, 3)
For instance, if you’re processing a series of records, a List of case classes may be easier to handle and more efficient than manipulating numerous Tuples.
4. Minimize Boxing
If your Tuple contains primitive types, consider alternatives that minimize boxing overhead. Use primitive collections like Array
or Vector
, where no boxing occurs:
val arrayExample = Array(1, 2, 3)
Working with primitive types directly can yield performance benefits, particularly in tight loops or high-frequency function calls.
5. Optimize Pattern Matching
Although pattern matching is a powerful feature in Scala, it might slow down your application if employed indiscriminately with Tuples. Limit its use to only when necessary and consider alternatives when performance is critical.
For example:
tupleExample match {
case (x, y, z) => // process values
}
This process can be optimized by leveraging methods directly on the Tuple, avoiding costly pattern matches where possible.
6. Use @specialized
Annotation
For performance-sensitive code, consider using the @specialized
annotation. It allows the compiler to generate optimized versions of classes for different types. This can reduce boxing overhead significantly:
class SpecializedTuple[@specialized T](val a: T, val b: T)
This can be particularly effective when Tuples contain frequently used types like Int, Double, or Boolean.
7. Benchmark and Profile
Prioritize benchmarking your code. Use tools like JMH (Java Microbenchmark Harness) to identify bottlenecks and ensure that your optimizations are yielding the desired performance improvements.
Here's a simple structure to benchmark Tuple performance:
import org.openjdk.jmh.annotations._
@State(Scope.Thread)
class TupleBenchmark {
val tuple = (1, 2, 3)
@Benchmark
def benchTupleAccess(): Int = {
tuple._1 + tuple._2 + tuple._3
}
}
Use JMH to run benchmarks on tuple access versus case class access to gather performance data.
To Wrap Things Up
While Scala Tuples are a powerful feature that can enhance code readability and flexibility, they can also introduce performance bottlenecks if not utilized carefully. By implementing the strategies discussed in this blog post—using case classes, minimizing Tuple sizes, avoiding boxing, optimizing pattern matching, and using profiling tools—you can significantly boost your application's performance.
In the world of coding, every optimization counts. As you strive for efficiency in Scala, remember that thoughtful usage of Tuples, along with these key strategies, will pave the way for smoother and faster applications.
For more information about improving Scala performance, consider checking the Scala documentation. Stay tuned for further articles as we delve deeper into Scala performance optimization techniques and best practices in future posts!