Mastering Web Tables: Tips to Handle Selenium Challenges
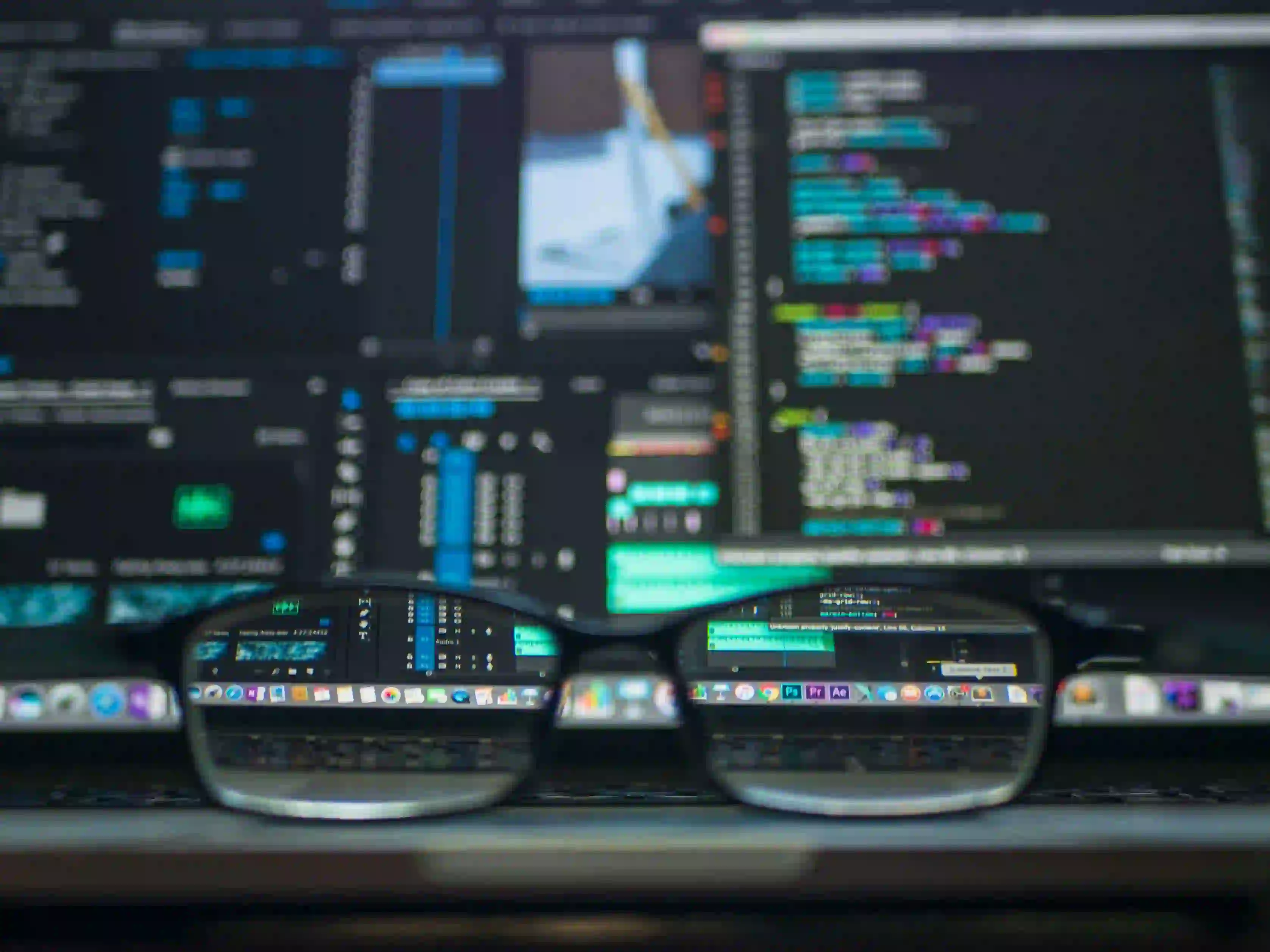
Mastering Web Tables: Tips to Handle Selenium Challenges
Web automation has become a crucial component of modern software development and testing. Among various web elements that testers and developers encounter, web tables tend to be a significant challenge due to their dynamic nature and intricate structure. In this blog post, we will explore effective techniques for handling web tables using Selenium, cover essential tips and tricks, and provide sample code snippets to help you master this skill.
Understanding Web Tables
Web tables are HTML tables that are used to display data in rows and columns. They can be found in various scenarios such as reports, data displays, and product listings. Browsers render these tables using the <table>
tag, which includes other tags like <tr>
for rows, <th>
for headers, and <td>
for data cells.
Key Challenges with Web Tables
- Dynamic Content: Web tables often update dynamically, making it difficult to locate elements.
- Variable Structures: Different tables may have varying structures, complicating the selection of elements.
- Complex Row Operations: Tables may include nested tables or checkboxes, which can complicate element interactions.
Setting Up Selenium
Before we dive into handling web tables, we should ensure we have a working Selenium setup. Here’s a quick guide for setting up Selenium with Java.
Dependencies
To start, make sure to include the Selenium Java library in your Maven project. Update your pom.xml
file:
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.0.0</version> <!-- Check for the latest version -->
</dependency>
WebDriver Initialization
Here’s a simple example of how to initialize the WebDriver:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class WebDriverSetup {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
// Your code goes here
driver.quit();
}
}
This setup initializes a Chrome browser window and directs it to a URL (replace https://example.com
with your actual target URL).
Navigating Web Tables
To effectively handle web tables, we must be able to interact with their elements programmatically. Below are strategies you can implement.
Locating Table Elements
To locate elements within a web table, make use of Selenium's capabilities to fetch rows and cells.
Sample Code for Retrieving Table Rows
Consider a sample table with this structure:
<table id="data-table">
<tr>
<th>Name</th>
<th>Age</th>
</tr>
<tr>
<td>John Doe</td>
<td>28</td>
</tr>
<tr>
<td>Jane Doe</td>
<td>30</td>
</tr>
</table>
You can retrieve table rows with the following code:
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import java.util.List;
public void fetchRows(WebDriver driver) {
WebElement table = driver.findElement(By.id("data-table"));
List<WebElement> rows = table.findElements(By.tagName("tr"));
for (WebElement row : rows) {
// Print the row text contents for observation
System.out.println(row.getText());
}
}
Accessing Specific Cells
When you need to access specific cells, you can do so by iterating over the rows and then the cells:
public void fetchCells(WebDriver driver) {
WebElement table = driver.findElement(By.id("data-table"));
List<WebElement> rows = table.findElements(By.tagName("tr"));
for (int i = 1; i < rows.size(); i++) { // Starting from 1 to skip headers
List<WebElement> cells = rows.get(i).findElements(By.tagName("td"));
// Accessing specific cell data (for example, Name and Age)
String name = cells.get(0).getText();
String age = cells.get(1).getText();
System.out.println("Name: " + name + ", Age: " + age);
}
}
Dynamic Web Tables
For tables that update dynamically, you might need efficient waits to ensure elements are present before interacting with them. Here is an example of a common wait strategy:
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
// Inside your method
WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("data-table")));
This will wait for up to 10 seconds until the table is visible before continuing.
Handling Complex Web Table Interactions
Selecting a Row Based on Content
Often, you might want to interact with a specific row based on its content. For example, deleting a user row based on their name can be done as follows:
public void deleteUserByName(WebDriver driver, String userName) {
WebElement table = driver.findElement(By.id("data-table"));
List<WebElement> rows = table.findElements(By.tagName("tr"));
for (WebElement row : rows) {
List<WebElement> cells = row.findElements(By.tagName("td"));
if (!cells.isEmpty() && cells.get(0).getText().equals(userName)) {
// Your delete logic goes here
System.out.println("Found user: " + userName + ". Deleting...");
// For example, clicking a delete button in the row (if it exists)
}
}
}
Dealing with Pagination
When dealing with web tables that have pagination, you typically want to loop through pages to find the information you need. A generic method might look like the following:
public void paginationHandler(WebDriver driver) {
boolean hasNextPage = true;
while (hasNextPage) {
fetchRows(driver); // Fetch data from the current page
// Check if there's a next page button and click it if it exists
try {
WebElement nextPageButton = driver.findElement(By.cssSelector(".next-page"));
if (nextPageButton.isDisplayed()) {
nextPageButton.click();
// Wait for the table to load on the next page
WebDriverWait wait = new WebDriverWait(driver, 10);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("data-table")));
} else {
hasNextPage = false;
}
} catch (NoSuchElementException e) {
hasNextPage = false; // No more pages
}
}
}
Additional Resources
For further reading on handling web tables in Selenium, you may find the following resources helpful:
- SeleniumHQ Documentation - Comprehensive guide to using Selenium.
- Web Tables Handling: A Practical Approach - A complete guide to mastering web tables.
Key Takeaways
Mastering web tables in Selenium can significantly improve your automation skills and efficiency. By using the right techniques, such as locating elements accurately, handling dynamic changes, and managing complex interactions, you can ensure your automation scripts are robust and reliable. As you continue to practice and refine your skills, you’ll find that tackling web tables, no matter how complex, becomes a manageable task.
Remember, practice sets apart great developers from good ones, so keep experimenting and optimizing your approach. Happy automating!