Common Pitfalls in Implementing the Decorator Pattern
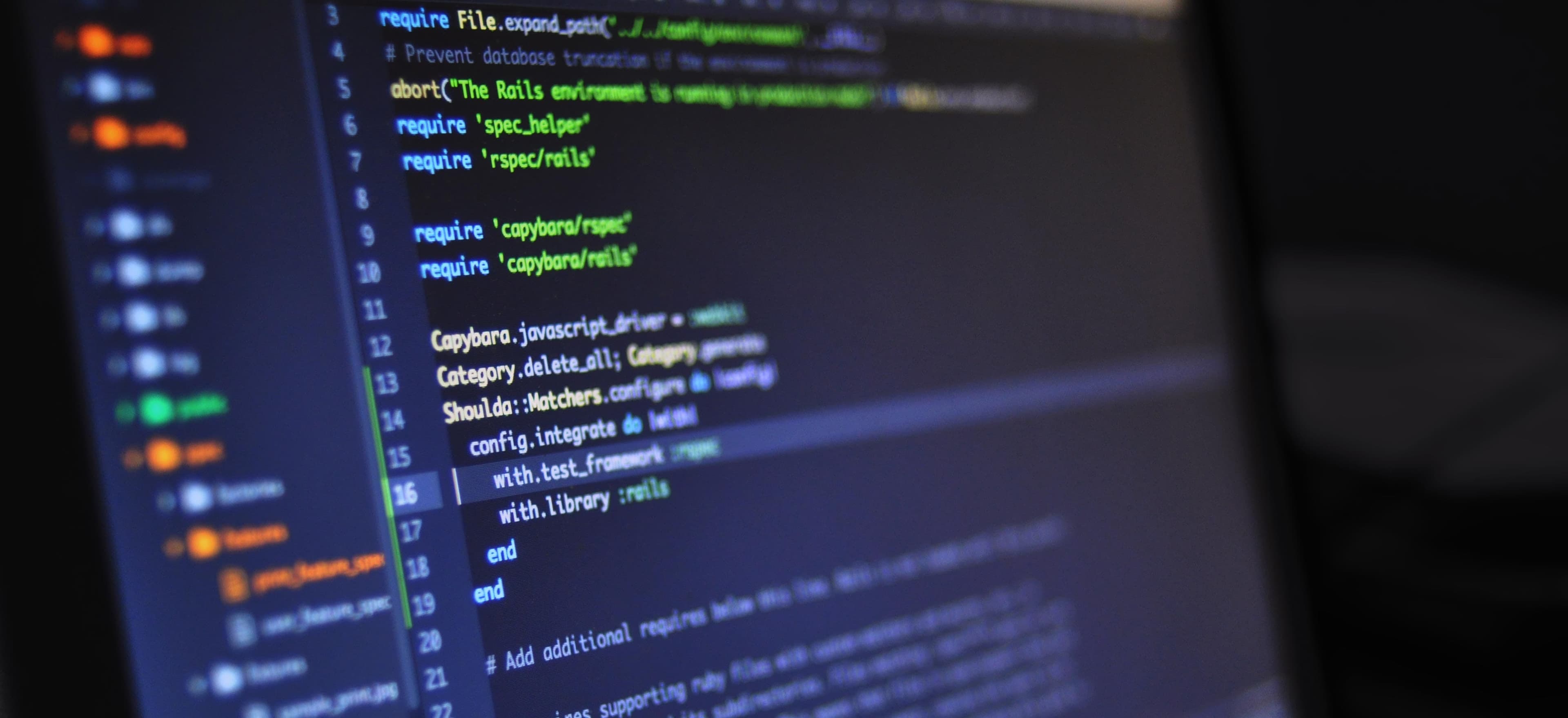
- Published on
Common Pitfalls in Implementing the Decorator Pattern
The Decorator Pattern is a powerful design pattern used in software engineering for adding new behaviors to objects at runtime without modifying their structure. In Java, it provides a flexible alternative to subclassing for extending functionality. However, like any tool in programming, it is not without its pitfalls. In this blog post, we will explore common mistakes made while implementing the Decorator Pattern in Java and how to avoid them.
What is the Decorator Pattern?
Before we delve into pitfalls, let’s quickly revisit what the Decorator Pattern is. The pattern allows for the dynamic addition of responsibilities to an object, enabling behaviors to be mixed and matched at runtime.
Key Components
- Component: An interface that defines the operations that can be dynamically added to concrete components.
- Concrete Component: An implementation of the Component interface. This is the object being decorated.
- Decorator: An abstract class that holds a reference to a concrete component and defines the same interface as the component.
- Concrete Decorators: These extend the Decorator class to add additional behavior.
Here is a simple implementation:
// The Component interface
public interface Coffee {
String getDescription();
double cost();
}
// The Concrete Component
public class SimpleCoffee implements Coffee {
@Override
public String getDescription() {
return "Simple Coffee";
}
@Override
public double cost() {
return 2.00;
}
}
This code defines a Coffee
interface and a simple implementation, SimpleCoffee
.
Decorating the Coffee
Now, let’s look at a simple decorator:
// The Decorator abstract class
public abstract class CoffeeDecorator implements Coffee {
protected Coffee coffee;
public CoffeeDecorator(Coffee coffee) {
this.coffee = coffee;
}
@Override
public String getDescription() {
return coffee.getDescription();
}
@Override
public double cost() {
return coffee.cost();
}
}
// Concrete Decorator
public class MilkDecorator extends CoffeeDecorator {
public MilkDecorator(Coffee coffee) {
super(coffee);
}
@Override
public String getDescription() {
return coffee.getDescription() + ", Milk";
}
@Override
public double cost() {
return coffee.cost() + 0.50; // Adding milk cost
}
}
Usage Example
public class CoffeeShop {
public static void main(String[] args) {
Coffee myCoffee = new SimpleCoffee();
System.out.println(myCoffee.getDescription() + " $" + myCoffee.cost());
myCoffee = new MilkDecorator(myCoffee);
System.out.println(myCoffee.getDescription() + " $" + myCoffee.cost());
}
}
This example demonstrates how the Decorator Pattern allows us to dynamically add milk to our coffee.
Common Pitfalls
1. Overusing Decorators
The Issue:
One common pitfall is the overuse of decorators which can lead to code that is difficult to read and maintain. Over-decorating can lead to a chain of decorators that is long and convoluted.
How to Avoid:
Limit the use of decorators to scenarios where they genuinely enhance functionality. Clearly document the chain and, if possible, use a builder pattern or a factory to encapsulate decorator creation.
2. Ignoring Interface Segregation
The Issue:
When creating decorators, developers may fail to properly adhere to the Interface Segregation Principle. This can result in decorators that expose methods not relevant to all decorators.
How to Avoid:
Ensure that each decorator adheres strictly to the component interface. Create dedicated interfaces for specific functionalities to avoid mixing unrelated methods.
public interface Milk {
String addMilk();
}
public class MilkDecorator extends CoffeeDecorator implements Milk {
// Implementation...
}
In this modified example, we create a dedicated Milk
interface.
3. Deep Inheritance Trees
The Issue:
Sometimes, developers will create a hierarchy of decorators, leading to deep inheritance trees that can increase complexity and reduce flexibility.
How to Avoid:
Consider composition over inheritance. While decorators inherently utilize inheritance, keep your design simple and avoid deep hierarchies. Favor a design where decorators can be created in a flat structure that allows for easier modification.
4. Not Considering Performance
The Issue:
A common mistake is ignoring the performance implications of using numerous decorators. Each decorator wraps the original object, which may incur additional overhead.
How to Avoid:
Profile your application to identify performance bottlenecks. Use decorators judiciously, and if performance is affected, consider alternatives like using a more efficient pattern.
5. Lack of Tests
The Issue:
Without appropriate tests, bugs can sneak into the decorator code when making changes or adding new decorators. This is particularly crucial as the number of decorators increases.
How to Avoid:
Implement unit tests for each decorator. Ensure that each addition is tested to verify that the entire chain of decorators interacts as expected.
public class MilkDecoratorTest {
@Test
public void testGetDescription() {
Coffee coffee = new SimpleCoffee();
Coffee milkCoffee = new MilkDecorator(coffee);
assertEquals("Simple Coffee, Milk", milkCoffee.getDescription());
}
@Test
public void testCost() {
Coffee coffee = new SimpleCoffee();
Coffee milkCoffee = new MilkDecorator(coffee);
assertEquals(2.50, milkCoffee.cost(), 0.01);
}
}
6. Failing to Maintain Immutability
The Issue:
When decorators modify the state of the underlying object, it can lead to unexpected behaviors, particularly in multi-threaded environments.
How to Avoid:
Aim to keep decorated objects immutable. Instead of modifying existing objects, return new decorated instances that encapsulate the changes.
7. Ignoring Composition
The Issue:
Poorly designed decorators may lead developers to forget about the potential benefits of composition over decoration. This can make it hard to scale.
How to Avoid:
Use decorators in conjunction with other patterns. For instance, Strategy and Command patterns can work well alongside decorators to build flexible architectures.
Lessons Learned
The Decorator Pattern is a cornerstone of flexible, object-oriented design, but it is fraught with potential pitfalls. By being aware of common issues such as overusing decorators, creating deep inheritance trees, or ignoring performance implications, developers can avoid these traps and create clean, maintainable code. Always remember to substantiate your decorations with proper testing and documentation.
As you implement the Decorator Pattern in your Java projects, keep these pitfalls in mind, and you'll be well on your way to mastering this powerful design technique. For more design patterns, check out the Java Design Patterns documentation. Happy coding!
Additional Resources
- UML for Design Patterns
- Effective Java by Joshua Bloch
- Design Patterns: Elements of Reusable Object-Oriented Software
Feel free to comment below your experiences or patterns you've developed using the Decorator Pattern!