Common Pitfalls in JavaFX Apps: Eizo Curator's Challenges
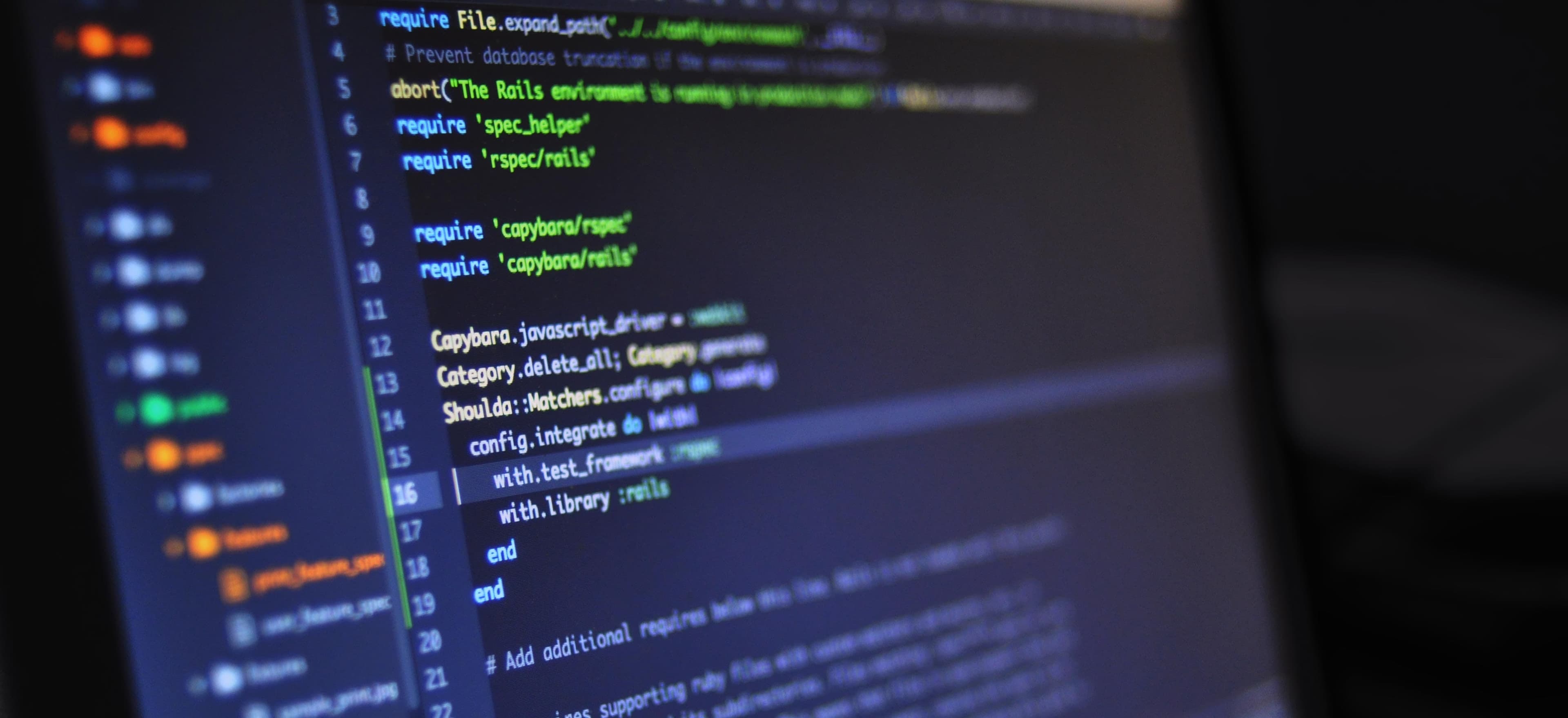
- Published on
Common Pitfalls in JavaFX Apps: Eizo Curator's Challenges
JavaFX, Java's standard framework for building rich desktop applications, offers a plethora of features that simplify UI development. However, those who delve into creating applications, such as Eizo Curator, a digital curation tool, may encounter several common pitfalls while navigating JavaFX's capabilities. This blog post will explore these challenges, offering guidance and examples to ensure a smoother application development experience.
Understanding JavaFX and Its Framework
Before diving into the pitfalls, it is important to comprehend what you are working with. JavaFX significantly differs from its predecessor, Swing, providing a robust canvas for building modern user interfaces. With features like CSS styling, built-in graphics libraries, and support for 3D graphics, JavaFX is a powerful tool.
For a more comprehensive overview of JavaFX features and benefits, you may find the official JavaFX documentation useful.
Common Pitfalls in JavaFX Development
1. Threading Issues: The JavaFX Application Thread
One of the most critical aspects to grasp in JavaFX is the importance of the JavaFX Application Thread (JFX) for UI updates. All interactions with UI components should occur on this thread; otherwise, you may encounter unexpected behavior, crashes, or performance bottlenecks.
Code Example
import javafx.application.Application;
import javafx.application.Platform;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ThreadExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Click me");
button.setOnAction(event -> {
// Updating UI on the Applications Thread
Platform.runLater(() -> {
System.out.println("Button clicked!");
});
});
StackPane root = new StackPane();
root.getChildren().add(button);
Scene scene = new Scene(root, 300, 250);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Here, Platform.runLater()
ensures that any UI component updates occur on the JFX thread. Failing to utilize this function when working with background threads would compromise your application’s stability.
2. Improper Resource Management
When developing applications that make extensive use of resources such as images, stylesheets, or media files, improper management can lead to memory leaks, especially in JavaFX applications.
Code Example
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class ResourceManagementExample extends Application {
private ImageView imageView;
@Override
public void start(Stage primaryStage) {
imageView = new ImageView(new Image("file:resources/image.png"));
StackPane root = new StackPane();
root.getChildren().add(imageView);
Scene scene = new Scene(root, 300, 250);
primaryStage.setScene(scene);
primaryStage.show();
}
@Override
public void stop() {
// Set imageView to null to allow garbage collection
imageView.setImage(null);
imageView = null;
}
public static void main(String[] args) {
launch(args);
}
}
In this example, nulled imageView
in the stop()
method allows garbage collection to reclaim memory. It's essential to manage resources effectively, particularly when the application runs for an extended time, to minimize memory footprint and leakage.
3. Inefficient Use of CSS
JavaFX allows for styling applications with CSS. However, using excessive or poorly structured CSS can lead to performance issues. Instead, keep your styles modular and organized.
Code Example
Here is a simple CSS stylesheet for a JavaFX application:
.button {
-fx-background-color: #2C3E50;
-fx-text-fill: white;
-fx-padding: 15px;
-fx-font-size: 14px;
}
.button:hover {
-fx-background-color: #34495E;
}
When using CSS, factor in the impact of unnecessary selectors, which may lead to sluggish performance.
4. Layout and Scene Graph Management
Creating complex user interfaces might lead to tangled layouts and inefficient scene graphs, making it difficult to manage. Understanding how JavaFX’s scene graph works is vital.
Instead of nesting layouts extensively, consider using layout managers like VBox
, HBox
, GridPane
, and others to achieve responsive designs without unnecessary complexity.
Code Example
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class LayoutExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
VBox vbox = new VBox(button1, button2);
Scene scene = new Scene(vbox, 200, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Using VBox
, in this case, provides a clear and manageable hierarchy within the scene graph. An organized scene graph can improve both performance and maintainability.
5. Ignoring Accessibility Features
Accessibility is vital for creating inclusive applications. JavaFX provides features to help in making applications accessible for users with disabilities. This includes setting tab order and using ARIA roles appropriately.
Ensure your applications support keyboard navigation and screen readers, improving usability for everyone.
Code Example
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class AccessibilityExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Accessible Button");
button.setAccessibleText("Click me to perform an action");
StackPane root = new StackPane();
root.getChildren().add(button);
Scene scene = new Scene(root, 300, 250);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In the example, setAccessibleText
enhances the UI’s accessibility. Emphasizing accessibility features from the start of your development process is best practice.
The Bottom Line
Creating a JavaFX application like Eizo Curator comes with unique challenges. By avoiding common pitfalls, utilizing resource management techniques, handling threading properly, and focusing on accessibility, you can create a robust application that performs well and is user-friendly.
Consider these best practices and principles to guide your development process. For more JavaFX tips and advanced techniques, check out the JavaFX official guides and become adept at building stunning user interfaces.
With this newfound understanding, you’ll be well-equipped to tackle your next JavaFX project confidently, paving the way for a successful application lifecycle. Happy coding!