Enhancing Realism: Addressing Performance Issues in Unity3D 3D Simulations
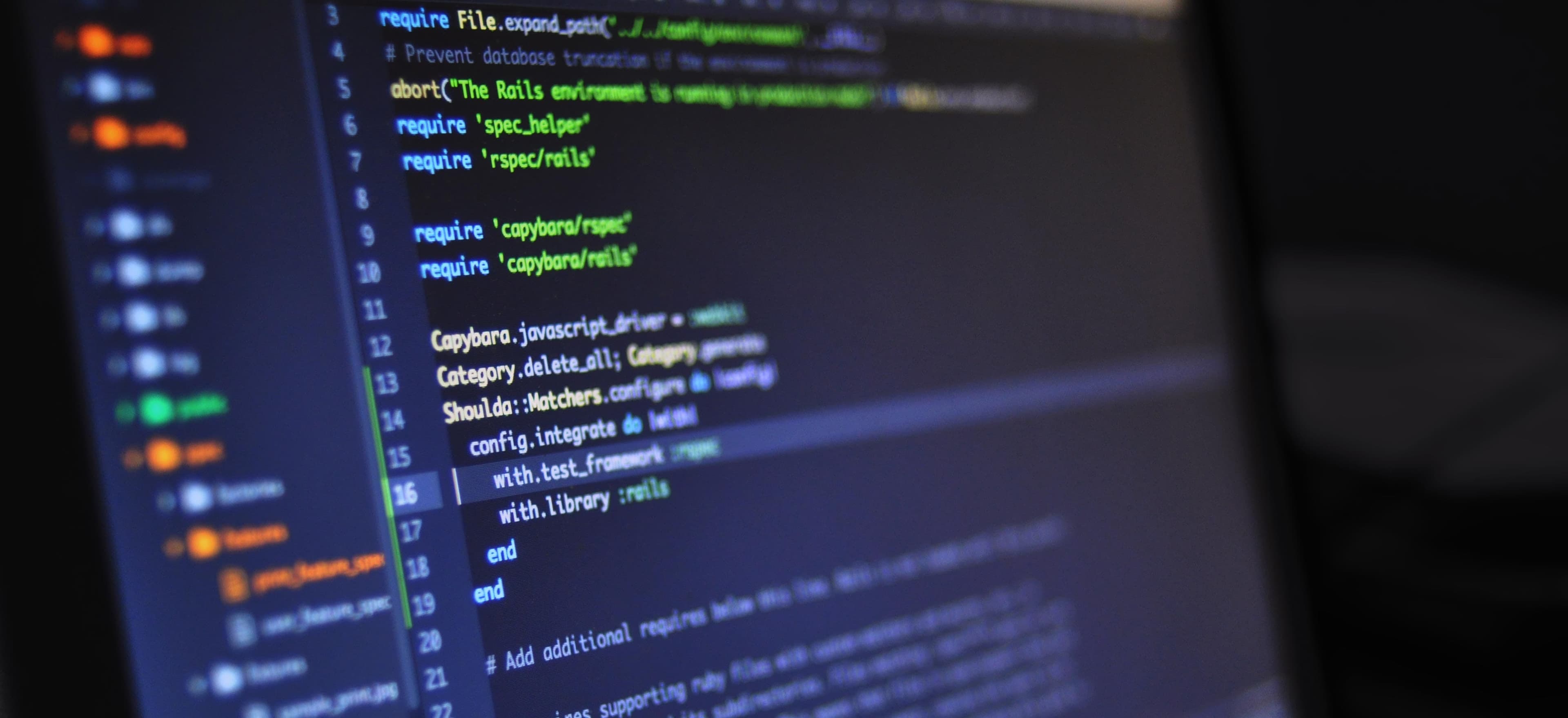
- Published on
Enhancing Realism: Addressing Performance Issues in Unity3D 3D Simulations
As developers, we constantly strive to create immersive and realistic 3D simulations in Unity3D. However, achieving realism comes with its own set of challenges, especially when it comes to optimizing performance. In this blog post, we will explore some common performance issues in Unity3D 3D simulations and discuss strategies to address them. Specifically, we will focus on optimizations to enhance realism in 3D simulations without sacrificing performance.
The Challenge of Realism and Performance
Realistic 3D simulations often involve complex models, high-resolution textures, advanced lighting effects, and intricate animations. While these elements contribute to the immersive experience, they can also put a strain on system resources, leading to performance issues such as low frame rates, stuttering, and lags. Balancing realism with performance is a crucial aspect of 3D simulation development, and it requires a strategic approach to optimization.
Identifying Performance Bottlenecks
Before delving into optimization techniques, it's important to identify the specific performance bottlenecks in your Unity3D 3D simulation. Common areas that can impact performance include:
- Draw Calls and Polygons: High numbers of draw calls and polygon counts can significantly impact the rendering performance of the simulation.
- Complex Shaders: Advanced and complex shaders can result in increased GPU workload, affecting frame rates.
- Physics Calculations: Detailed physics simulations and interactions can consume CPU resources, leading to performance issues.
- Texture Usage: Large textures and inefficient use of texture memory can cause memory-related performance issues.
Once you've pinpointed the performance bottlenecks, you can start implementing targeted optimizations to address them.
Optimization Techniques for Realistic 3D Simulations
Level of Detail (LOD) Techniques
One effective way to manage performance in realistic 3D simulations is by implementing Level of Detail (LOD) techniques. LOD allows you to render objects with varying levels of detail based on their distance from the camera. This means that objects farther away from the camera can be rendered with lower detail, reducing the overall rendering workload.
// Example of LODGroup setup in Unity3D
LODGroup lodGroup = GetComponent<LODGroup>();
lodGroup.SetLODs(lodLevels);
By utilizing LOD techniques for models, terrains, and other complex assets, you can maintain visual fidelity up close while optimizing performance for distant objects.
Texture Compression and Streaming
Large texture files can significantly impact performance and memory usage in 3D simulations. Implementing texture compression techniques, such as using formats like ASTC or DXT, can help reduce memory footprint and bandwidth requirements. Additionally, utilizing texture streaming can dynamically load and unload textures based on the proximity of the camera, further optimizing memory usage.
// Texture compression in Unity3D
Texture2D texture = Resources.Load<Texture2D>("myTexture");
texture.Compress(true);
By incorporating texture compression and streaming, you can minimize the performance impact of high-resolution textures, ensuring smoother rendering in realistic 3D simulations.
GPU Instancing for Efficient Rendering
When dealing with a large number of identical or similar objects in a scene, GPU instancing can significantly improve rendering performance. Instead of issuing individual draw calls for each object, GPU instancing allows the GPU to render multiple instances of the same mesh with a single draw call, reducing CPU overhead and improving overall rendering efficiency.
// Example of GPU instancing in Unity3D
Graphics.DrawMeshInstanced(mesh, 0, material, matrices);
By leveraging GPU instancing for objects like trees, rocks, or other repeated elements, you can optimize rendering performance while maintaining visual richness in your 3D simulations.
Asynchronous Loading and Background Processing
In complex 3D simulations, asset loading and processing can introduce performance overhead, especially during runtime. Implementing asynchronous loading techniques and background processing for resource-intensive tasks, such as scene loading, asset instantiation, and physics calculations, can help mitigate performance dips and minimize hitches during gameplay.
// Asynchronous scene loading in Unity3D
AsyncOperation asyncLoad = SceneManager.LoadSceneAsync("myScene", LoadSceneMode.Additive);
By offloading heavy tasks to background threads and utilizing asynchronous loading, you can ensure a smoother and more responsive experience in your realistic 3D simulations.
Key Takeaways
Developing realistic 3D simulations in Unity3D requires a careful balance of visual fidelity and performance optimization. By identifying performance bottlenecks and implementing targeted optimizations such as LOD techniques, texture compression, GPU instancing, and asynchronous loading, developers can enhance the realism of their simulations while delivering smooth and immersive experiences for users.
Incorporating these optimization strategies not only improves the performance of 3D simulations but also demonstrates a commitment to creating engaging and realistic virtual environments.
For further in-depth discussions on Unity3D optimization and 3D simulation development, you can explore resources such as the Unity3D official documentation and Unite conferences for insights from industry experts.
Remember, the pursuit of realism in 3D simulations is as much a technical endeavor as it is an artistic one, and finding the right balance between the two is key to crafting truly captivating virtual experiences.
Checkout our other articles