Dynamic vs Static Typing: The Pitfalls You Need to Avoid
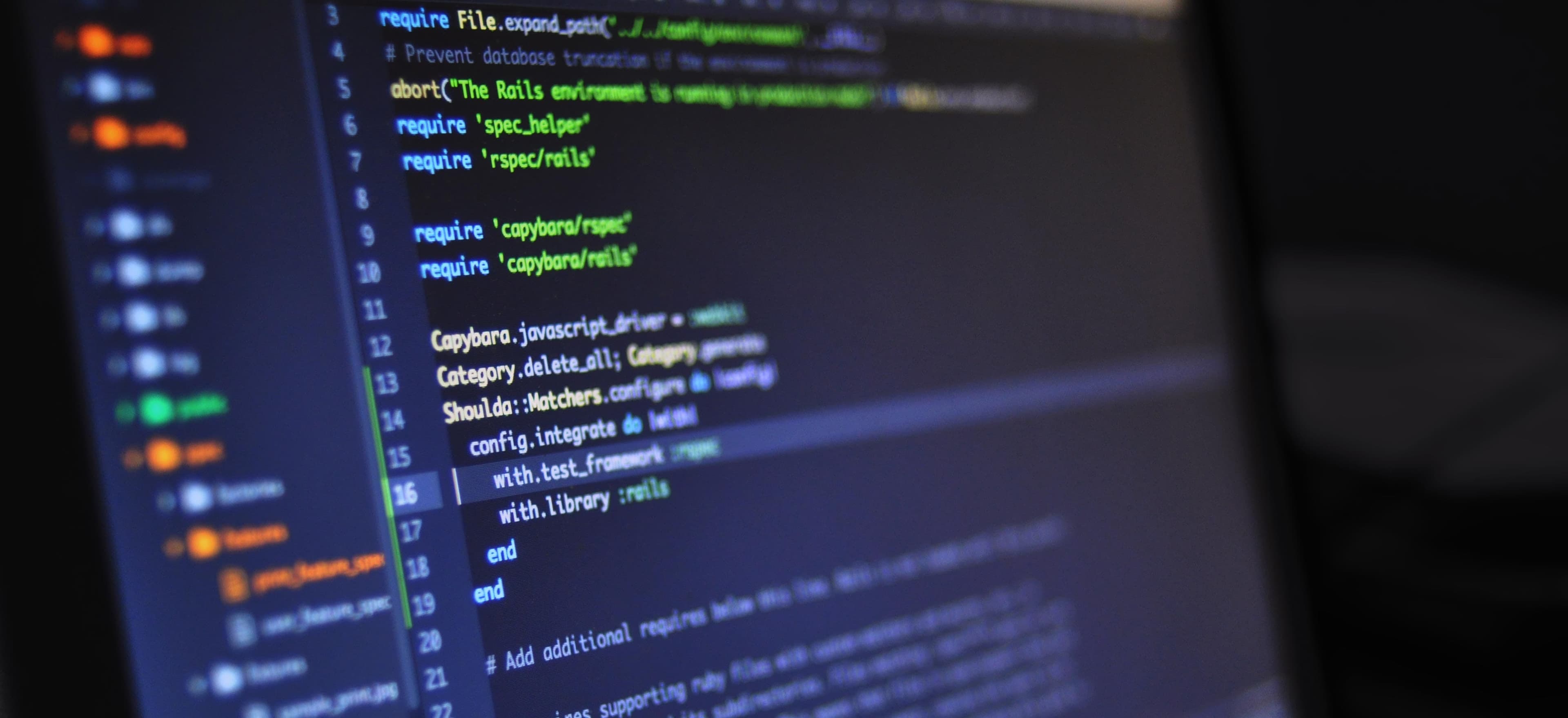
- Published on
Dynamic vs Static Typing: The Pitfalls You Need to Avoid
In the ever-evolving world of programming, the choice of a programming language can significantly impact your development experience. One debate that continues to be at the forefront of this discussion is about dynamic vs. static typing. While both have their own merits, they come with their unique challenges. In this post, we will explore these two typing systems, their advantages, pitfalls, and best practices to help you make an informed choice in your development journey.
Understanding Typing: What Does It Mean?
Before diving deeper, let's clarify what we mean by "typing" in programming. Typing refers to how a programming language handles data types. It dictates whether the type of a variable is determined at compile time (static typing) or at runtime (dynamic typing).
-
Static Typing: In static typing, the type of a variable is known at compile time. Once a variable is declared as a certain type, it cannot change. Languages that use static typing include Java, C++, and Go.
-
Dynamic Typing: In dynamic typing, a variable can hold any type of data and its type may change at runtime. Languages like Python, JavaScript, and Ruby embrace this flexibility.
The Case for Static Typing
-
Type Safety: One of the most significant advantages of static typing is type safety. Errors related to data types can be caught at compile time, preventing runtime issues.
public class Example { public static void main(String[] args) { int number = 5; String text = "Hello"; // The following line will cause a compile-time error // number = text; } }
In the above code, attempts to assign a
String
to anint
variable will throw a compile-time error, ensuring robust code. -
Improved Performance: Since types are known in advance, statically typed languages can compile code to run faster, leading to better performance in CPU-intensive applications.
-
Better IDE Support: Integrated development environments (IDEs) can provide powerful auto-completion, navigation, and refactoring tools when types are known. This speeds up the development process significantly.
The Pitfalls of Static Typing
While static typing provides several benefits, it also has notable pitfalls:
-
Verbosity: Static types often lead to more verbose code. This can make it daunting for beginners or for those transitioning from dynamic languages.
List<String> stringList = new ArrayList<String>();
In contrast, below is a more succinct representation in dynamic languages:
string_list = []
-
Reduced Flexibility: Once a variable type is declared statically, changing it becomes cumbersome. This lack of flexibility can slow development and easy experimentation, particularly in prototyping phases.
Advantages of Dynamic Typing
-
Simplicity and Conciseness: Dynamic typing allows for simpler code, requiring less boilerplate around type declarations.
number = 5 text = "Hello"
It provides a more straightforward way to define variables without tracking types explicitly.
-
Rapid Development: The flexibility of changing types and structures quickly facilitates rapid application development. This can be particularly beneficial in startups or projects requiring constant evolution.
-
Easier Prototyping: When "throwing ideas against the wall to see what sticks," a dynamic language is incredibly powerful.
The Pitfalls of Dynamic Typing
Dynamic typing, however, comes with its share of pitfalls:
-
Runtime Errors: As types are not enforced until runtime, errors may arise unexpectedly, leading to potential system failures or crashes.
number = 5 number = "Hello" # This is allowed # Later when trying to perform a numeric operation: print(number * 2) # This will raise an error at runtime
-
Lost Context: Code can become harder to follow since the type of a variable is not explicit, making it more challenging to understand what data is expected.
-
Debugging Challenges: Finding and fixing errors in dynamically typed languages can often require more effort. The absence of clear type expectations makes tracing errors difficult.
Choosing Between Dynamic and Static Typing
The decision to use a dynamically typed or statically typed language greatly depends on specific use cases, team familiarity, and project requirements. Here's a guideline to simplify your choice:
-
Use Dynamic Typing When:
- Rapid prototyping or iterative processes are essential.
- The project scope is well-defined and flexibility is crucial.
- You’re working in a domain where requirements frequently evolve.
-
Use Static Typing When:
- You are working on large codebases where maintainability and robustness are priorities.
- Performance matters, especially in data-heavy applications.
- Your team values strong type hints and better tooling support.
Bridging the Gap: Type Inference
Some modern languages offer a middle ground through features such as type inference, where the compiler can automatically deduce types, blending benefits of both typing paradigms. For example, Java's recent versions introduced local variable type inference:
var number = 5; // The compiler infers number as an int
var text = "Hello"; // The compiler infers text as a String
Lessons Learned
Ultimately, the debate between dynamic and static typing centers on preferences for flexibility versus safety. Understanding the advantages and pitfalls of both will set you on the right path for your projects. Remember, each program and team has distinct needs that can dictate the most suitable option.
For further exploration, consider reading more on static typing here and dynamic typing here.
By weighing these considerations against your project's goals, you can choose a typing system that offers the best advantages. As programming languages continue to evolve, staying informed on these typological debates can empower you to write cleaner, more maintainable, and efficient code.
Checkout our other articles