Common Pitfalls When Starting with Apache Mahout
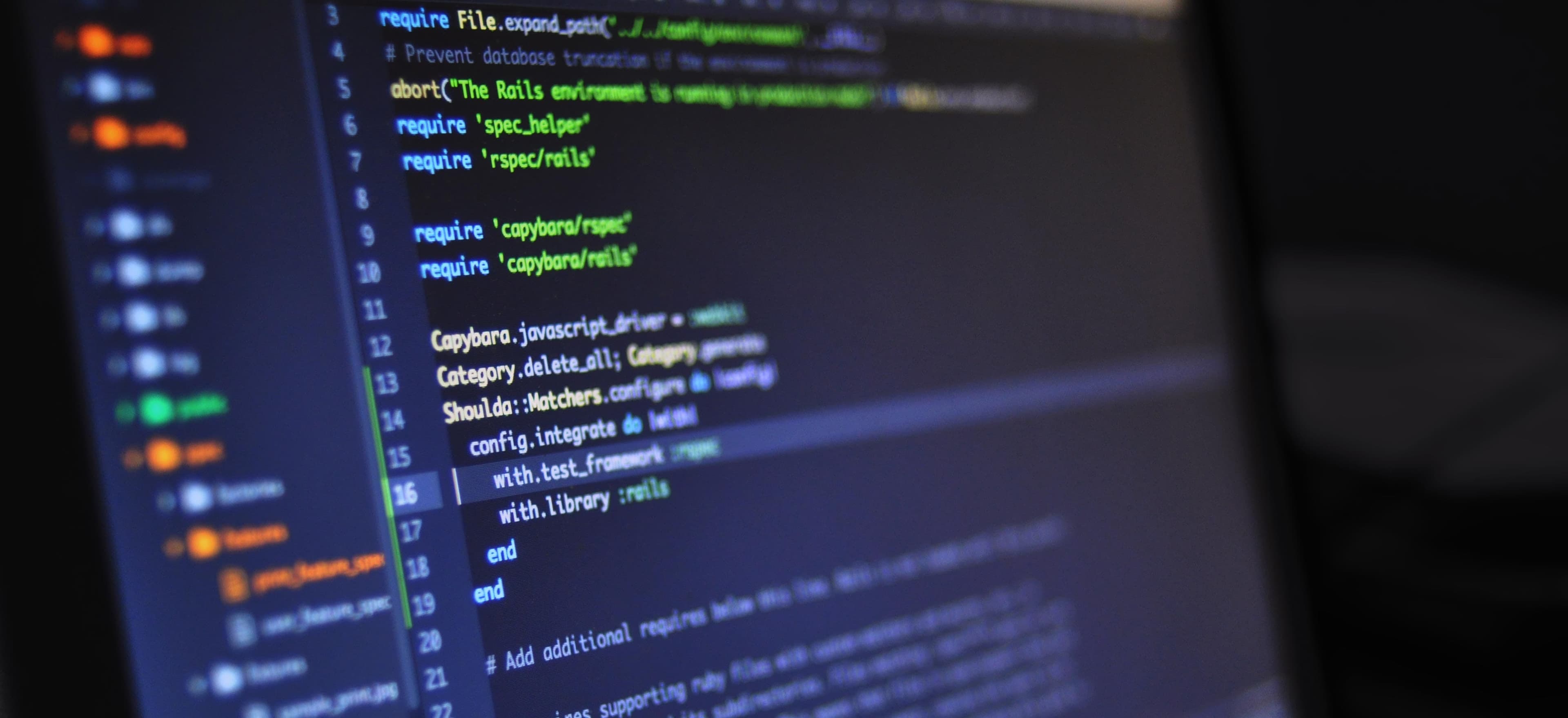
- Published on
Common Pitfalls When Starting with Apache Mahout
Apache Mahout is a powerful library for creating scalable machine learning applications. As you dive into Mahout, new users may encounter several common pitfalls that can hinder their progress. This blog post will outline these pitfalls, providing insights and tips for avoiding them. By understanding these challenges, you can streamline your journey into the world of scalable machine learning with Mahout.
A Quick Look to Apache Mahout
Apache Mahout is primarily known for its ability to write scalable algorithms focused on linear algebra, clustering, classification, and collaborative filtering. Leveraging the power of Apache Hadoop, Mahout offers the ability to work with vast datasets efficiently. However, the learning curve can be steep, and new users may face certain barriers when getting started.
Common Pitfall 1: Ambiguous Understanding of Libraries and Dependencies
One common misunderstanding when starting with Mahout is the confusion regarding its dependencies. Apache Mahout does not operate in a vacuum. It relies on several other libraries, such as Hadoop and Spark.
Why This Matters
Understanding the dependencies is crucial because they can affect how you implement Mahout in your projects. Failing to install or configure these dependencies correctly can lead to runtime errors or performance issues.
Solution
Make sure to refer to the official Mahout documentation for the latest information on dependencies. Plan your environment setup carefully:
- Install Hadoop: Ensure you have the proper version to match your Mahout installation.
- Set Up Spark: If you are using Mahout with Apache Spark, check for version compatibility and resource allocation.
Common Pitfall 2: Neglecting to Explore Data Preprocessing
Many new users jump straight into applying machine learning algorithms without adequately preprocessing their data. Data preprocessing is essential for achieving accurate and meaningful results.
Why This Matters
Unprocessed or poorly processed data can lead to misleading outcomes, reducing the effectiveness of your models.
Solution
Always incorporate a robust data preprocessing pipeline. Below is an example of a simple preprocessing step using Mahout.
import org.apache.mahout.math.DenseVector;
import org.apache.mahout.math.Vector;
public class DataPreprocessing {
public static void main(String[] args) {
// Raw data as a simple array
double[] rawData = {1.0, 2.0, 3.0, -1.0};
// Normalize the data
Vector vector = new DenseVector(rawData);
double mean = vector.zSum() / vector.size();
for(int i = 0; i < vector.size(); i++) {
vector.set(i, vector.get(i) - mean);
}
System.out.println("Normalized Data: " + vector);
}
}
In this example, we normalize our raw data by subtracting the mean. Normalization is key because it helps eliminate biases due to scale, making the machine learning algorithms work more efficiently.
Common Pitfall 3: Ignoring Algorithm Selection and Tuning
Another frequent pitfall is failing to choose the right algorithm for your specific problem or neglecting to tune its parameters. Not all algorithms fit every dataset, and poor choices can result in suboptimal performance.
Why This Matters
Algorithms have strengths and weaknesses. If you do not select an appropriate algorithm, you may overlook valuable insights hidden within your data.
Solution
- Understand your data: Before selecting an algorithm, analyze the size, type, and distribution of your data.
- Experiment with multiple algorithms: Leverage Mahout’s support for various machine learning algorithms like k-means clustering, random forests, etc.
Common Pitfall 4: Insufficient Model Evaluation
A frequent mistake is failing to properly evaluate your model's performance. Without adequate evaluation, it is impossible to know how well your model is performing and whether it can generalize to unseen data.
Why This Matters
Evaluating a model is critical for understanding its efficacy and reliability. It's essential to avoid overfitting and underfitting at all costs.
Solution
Use Mahout's built-in capabilities to evaluate your model. For classification tasks, you can use accuracy and confusion matrices. Here’s a simple example of how to evaluate a classification model with Mahout:
import org.apache.mahout.classifier.Classifier;
import org.apache.mahout.classifier.ConfusionMatrix;
// Assuming classifier is your trained model and testData is your dataset
Classifier classifier = ...;
Dataset testData = ...;
// Calculate the confusion matrix
ConfusionMatrix matrix = new ConfusionMatrix();
matrix.generateMatrix(classifier, testData);
// Output the confusion matrix
System.out.println("Confusion Matrix: " + matrix);
This snippet calculates and prints the confusion matrix, allowing you to visualize how well your model is performing.
Common Pitfall 5: Neglecting Parallelism and Scalability
One of Mahout’s key features is its ability to run on a distributed system, yet some newcomers neglect to take advantage of its parallel processing capabilities.
Why This Matters
By not optimizing for distributed computing, users may miss out on Mahout's capability to handle large datasets effectively, leading to inefficient processing.
Solution
Learn how to configure Mahout for distributed execution. Use Hadoop's MapReduce capabilities and utilize Mahout's built-in optimizations. Here's a conceptual framework on how to write a simple MapReduce job using Mahout.
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.mahout.mapreduce.clustering.ClusterWritable;
import org.apache.mahout.mapreduce.clustering.KMeansClusterer;
// Configuration for Hadoop job
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "K-means Clustering");
// Set up the job parameters
job.setMapperClass(YourMapperClass.class);
job.setReducerClass(YourReducerClass.class);
job.setJarByClass(YourMainClass.class);
job.setOutputKeyClass(ClusterWritable.class);
job.setOutputValueClass(...);
// Add input and output paths
FileInputFormat.setInputPaths(job, new Path("input/path"));
FileOutputFormat.setOutputPath(job, new Path("output/path"));
// Submit the job
System.exit(job.waitForCompletion(true) ? 0 : 1);
In this example, we are establishing a MapReduce job for K-means clustering. Remember to tweak the parameters and explore Mahout's API to take further advantage of faster processing.
Wrapping Up
Apache Mahout offers immense potential for building scalable machine learning models. However, it's important to be aware of the common pitfalls that can arise during the early stages of learning. Ensure you understand the dependencies, preprocess your data effectively, choose and tune algorithms carefully, rigorously evaluate model performance, and utilize the power of parallelism.
By navigating these challenges, you set yourself up for success in leveraging Mahout for your machine learning endeavors. For further reading, consider exploring Mahout's official documentation and community forums for additional support. Happy modeling!
Checkout our other articles