Mastering JSR-303: Loading Messages from I18N Properties
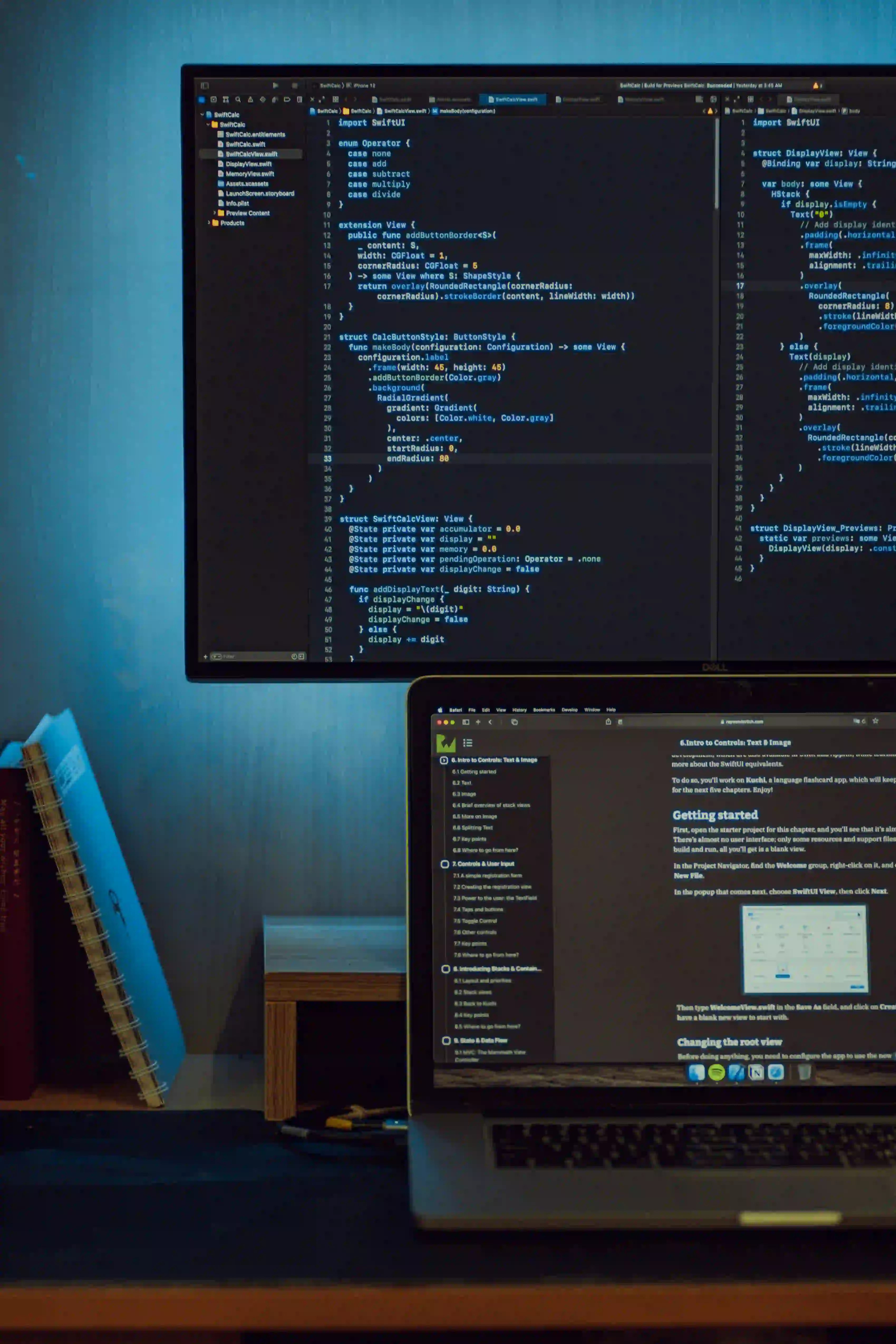
Mastering JSR-303: Loading Messages from I18N Properties
Java's Standard Reference (JSR) specification defines a set of constraints for bean validation. Specifically, JSR-303 introduces the concepts of validation annotations that allow developers to enforce validation rules on Java objects seamlessly. Furthermore, internationalization (I18N) is crucial for developing applications that can cater to multiple languages and regions. In this blog post, we will delve into the harmony between these two important concepts: JSR-303 validation and loading messages from I18N property files.
What is JSR-303?
JSR-303, known as Bean Validation, is a Java specification that provides a framework for metadata creation and validation in JavaBeans. The validation process helps ensure that bean properties adhere to defined constraints, thereby maintaining data integrity in applications.
Some key features of JSR-303 include:
- Annotations for validation: JSR-303 provides various annotations like
@NotNull
,@Size
, and@Email
. - Custom validation: Developers can create custom validation annotations.
- Validation message support: Messages can be defined for user feedback.
The Role of I18N Properties
Internationalization (I18N) enables applications to display messages in different languages. Properties files are a common way to store localized messages in Java, allowing you to keep your validation messages consistent with the language context of the user.
Consider this typical structure for I18N property files:
messages_en.properties
messages_fr.properties
In these files, you can define keys and values for localized messages.
Example of messages_en.properties
:
NotNull.field=The field cannot be null
Size.field=The field size must be between {min} and {max}
And for French in messages_fr.properties
:
NotNull.field=Le champ ne peut pas être nul
Size.field=La taille du champ doit être entre {min} et {max}
By following this approach, you ensure that your applications are accessible and friendly to users across different regions.
Loading Messages in JSR-303
To utilize these messages within your application, you must configure the ValidationMessages.properties file. By default, JSR-303 looks for a file named ValidationMessages.properties in the classpath.
Example Code Snippet
Let's look at an example of how to set up validation rules with JSR-303 and load messages from I18N properties for user feedback.
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
public class User {
@NotNull(message = "{NotNull.field}")
@Size(min = 2, max = 14, message = "{Size.field}")
private String username;
// constructors, getters, and setters
}
In this code snippet, we define a simple User
class with a username
field. The @NotNull
and @Size
annotations enforce validation constraints. The messages are referenced with keys pointing to the entries in the I18N properties files.
Setting Up the Validation Factory
In order to validate the User
class and retrieve those messages, you would need to create a Validator
instance as follows:
import javax.validation.Validation;
import javax.validation.Validator;
import javax.validation.ValidatorFactory;
public class ValidationUtil {
private static final Validator validator;
static {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
validator = factory.getValidator();
}
public static Validator getValidator() {
return validator;
}
}
Running the Validation Logic
Now that we have set up the validation factory, it's time to validate a User
object.
import javax.validation.ConstraintViolation;
import java.util.Set;
public class ValidationExample {
public static void main(String[] args) {
User user = new User(); // username is null to trigger validation
Set<ConstraintViolation<User>> violations = ValidationUtil.getValidator().validate(user);
for (ConstraintViolation<User> violation : violations) {
System.out.println(violation.getMessage());
}
}
}
In this main method, we create an instance of the User
class without initializing the username
field. When we invoke validate
, it returns a set of constraint violations where messages are pulled from the I18N properties files as per the keys specified in the annotations.
This code will output:
The field cannot be null
If you change the user's username to a string that's too short or too long, you will receive appropriate messages from your I18N properties.
Custom Validation with JSR-303
Custom validation can add more layered complexity and business logic to your validations. Here's how to implement a custom annotation.
Step 1: Define the Custom Annotation
Start by creating a custom annotation:
import javax.validation.Constraint;
import javax.validation.Payload;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Constraint(validatedBy = CustomValidator.class)
@Target({ElementType.FIELD, ElementType.METHOD, ElementType.ANNOTATION_TYPE, ElementType.PARAMETER})
@Retention(RetentionPolicy.RUNTIME)
public @interface ValidUsername {
String message() default "{ValidUsername.field}";
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
}
Step 2: Implement the Custom Validator
Now, implement the logic for this custom annotation.
import javax.validation.ConstraintValidator;
import javax.validation.ConstraintValidatorContext;
public class CustomValidator implements ConstraintValidator<ValidUsername, String> {
@Override
public void initialize(ValidUsername constraintAnnotation) {
}
@Override
public boolean isValid(String username, ConstraintValidatorContext context) {
return username != null && username.matches("[A-Za-z0-9_]+");
}
}
Step 3: Use the Custom Annotation
Link the custom annotation to your User
class:
public class User {
@ValidUsername(message = "{ValidUsername.field}")
private String username;
// constructors, getters, and setters
}
Internationalization of Custom Messages
For proper localization, make sure your property files include a key such as:
ValidUsername.field=This username is invalid. Only alphanumeric characters are allowed.
In conclusion, the combination of JSR-303 and I18N properties equips your Java application to not only enforce data integrity but also to convey meaningful messages to users in their native language. This enhances usability and accessibility across different regions and cultures.
Wrapping Up
In this blog post, we dissected the relationship between JSR-303 and I18N properties, demonstrating how to implement and load internationalized messages for validation errors. With these strategies, your applications will not only be more robust but also user-friendly for a global audience.
For more information about JSR-303, check out the official JSR-303 documentation. Happy coding!